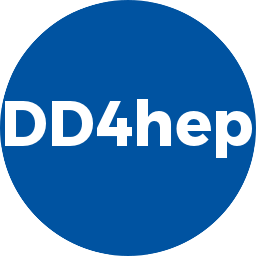 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
34 #include <G4Threading.hh>
35 #include <G4AutoLock.hh>
39 G4Mutex creation_mutex=G4MUTEX_INITIALIZER;
45 template <
typename TYPE>
static inline TYPE* checked_value(TYPE* p) {
49 except(
"Geant4Handle",
"Attempt to access an invalid object of type:%s!",
50 typeName(
typeid(TYPE)).c_str());
74 std::string _t = typeName(
typeid(TYPE));
75 printout(DEBUG,
"Geant4Handle",
"Object factory for %s not found. Try out %s",
76 typ.
second.c_str(), _t.c_str());
77 object = PluginService::Create<Geant4Action*>(_t, ctxt, typ.
second);
79 size_t idx = _t.rfind(
':');
80 if (idx != std::string::npos)
81 _t = std::string(_t.substr(idx + 1));
82 printout(DEBUG,
"Geant4Handle",
"Try out object factory for %s",_t.c_str());
83 object = PluginService::Create<Geant4Action*>(_t, ctxt, typ.
second);
87 TYPE* ptr =
dynamic_cast<TYPE*
>(object);
91 std::string _t = typeName(
typeid(TYPE));
92 except(
"Geant4Handle",
"Failed to convert object of type '%s' with name '%s' to handle of type '%s'!",
95 except(
"Geant4Handle",
"Failed to create object of type %s!", typ.
first.c_str());
99 template <
typename TYPE,
typename CONT>
102 const std::string& type_name,
103 const std::string& shared_typ,
109 typedef typename TYPE::shared_type _ST;
111 _ST*
object = (_ST*)_create_object<TYPE>(kernel,s_type);
112 CONT& container = (k.*pmf)();
115 G4AutoLock protection_lock(&creation_mutex);
116 value = container.get(typ.
second);
118 value = _create_object<TYPE>(k,typ);
119 container.adopt(value);
124 value->info(
"+++ Created shared object for %s of type %s.",
125 typ.
second.c_str(),typeName(
typeid(TYPE)).c_str());
128 TYPE* value = _create_object<TYPE>(k,typ);
132 template <
typename TYPE>
137 template <
typename TYPE>
139 value = _create_object<TYPE>(kernel,
TypeName::split(type_name_char ? type_name_char :
"????"));
157 value = checked_value(p);
164 return pm[property_name];
168 return checked_value(value);
176 return checked_value(value);
180 return checked_value(value);
184 return checked_value(value);
192 if ( value ) value->addRef();
193 if ( point ) point->release();
207 if ( pointer != value ) {
210 if ( value ) value->addRef();
227 except(
"KernelHandle",
"Cannot access worker context [Invalid Handle]");
238 "Geant4SharedRunAction", shared,
null());
243 "Geant4SharedRunAction", shared,
null());
249 "Geant4SharedEventAction", shared,
null());
254 "Geant4SharedEventAction", shared,
null());
260 "Geant4SharedGeneratorAction", shared,
null());
265 "Geant4SharedGeneratorAction", shared,
null());
271 "Geant4SharedTrackingAction", shared,
null());
276 "Geant4SharedTrackingAction", shared,
null());
282 "Geant4SharedSteppingAction", shared,
null());
287 "Geant4SharedSteppingAction", shared,
null());
293 "Geant4SharedStackingAction", shared,
null());
298 "Geant4SharedStackingAction", shared,
null());
302 const std::string& detector,
bool ) {
315 printout(ERROR,
"Geant4Handle<Geant4Sensitive>",
"Exception: %s", e.what());
318 printout(ERROR,
"Geant4Handle<Geant4Sensitive>",
"Exception: Unknown exception");
320 except(
"Geant4Handle<Geant4Sensitive>",
321 "Failed to create sensitive object of type %s for detector %s!",
322 type_name.c_str(), detector.c_str());
TYPE * value
Pointer to referenced object.
The property class to assign options to actions.
virtual DetElement detector(const std::string &name) const =0
Retrieve a subdetector element by its name from the detector description.
void exception(const std::string &src, const std::string &msg)
Property & operator[](const std::string &property_name) const
Property accessor.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
Handle to Geant4 actions with built-in creation mechanism.
KernelHandle worker()
Access to worker thread.
Geant4Context * workerContext()
Thread's Geant4 execution context.
Detector & detectorDescription() const
Access to detector description.
Geant4Kernel & worker(unsigned long thread_identifier, bool create_if=false)
Access worker instance by its identifier.
TYPE * _create_object(Geant4Kernel &kernel, const TypeName &typ)
Geant4StackingActionSequence & stackingAction()
Access stacking action sequence.
~Geant4Handle()
Default destructor.
static Detector & getInstance(const std::string &name="default")
—Factory method----—
Handle class describing a detector element.
Geant4EventActionSequence & eventAction()
Access run action sequence.
Geant4Kernel * value
Pointer to referenced object.
Geant4TrackingActionSequence & trackingAction()
Access tracking action sequence.
Geant4Handle & operator=(const Geant4Handle &handle)
Assignment operator.
Geant4RunActionSequence & runAction()
Access run action sequence.
Default base class for all Geant 4 actions and derivates thereof.
static unsigned long int thread_self()
Access thread identifier.
TYPE * release()
Release the underlying object.
TYPE * operator->() const
Access to the underlying object.
Geant4SteppingActionSequence & steppingAction()
Access stepping action sequence.
static TypeName split(const std::string &type_name)
Split string pair according to default delimiter ('/')
Geant4Handle()=default
Default constructor.
void destroy()
Destroy referenced object (program termination)
Handle to Geant4 actions with built-in creation mechanism.
bool operator!() const
Validity check.
KernelHandle()
Default constructor.
bool isMultiThreaded() const
static Geant4Kernel & instance(Detector &description)
Instance accessor.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Manager to ease the handling of groups of properties.
Class, which allows all Geant4Action to be stored.
TYPE * get() const
Access to the underlying object.
TYPE * _create_share(Geant4Kernel &kernel, CONT &(Geant4ActionContainer::*pmf)(), const std::string &type_name, const std::string &shared_typ, bool shared, TYPE *)
Namespace for the AIDA detector description toolkit.
Helper class to handle strings of the format "type/name".
The main interface to the dd4hep detector description package.
Geant4GeneratorActionSequence & generatorAction()
Access generator action sequence.
The base class for Geant4 sensitive detector actions implemented by users.
void checked_assign(TYPE *p)
Geant4Kernel & master() const
Thread's master context.
void handle(const O *o, const C &c, F pmf)
Geant4Action * action() const
Access to the underlying object.
Generic context to extend user, run and event information.