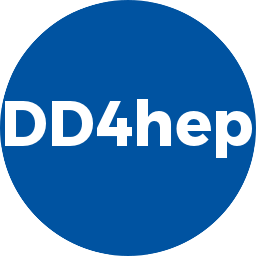 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4HANDLE_H
15 #define DDG4_GEANT4HANDLE_H
41 template <
typename TYPE>
class Geant4Handle {
44 TYPE*
null() {
return nullptr; }
83 operator TYPE*()
const;
123 #endif // DDG4_GEANT4HANDLE_H
TYPE * value
Pointer to referenced object.
The property class to assign options to actions.
Geant4Kernel * operator->() const
Access to the underlying object.
Property & operator[](const std::string &property_name) const
Property accessor.
Geant4Kernel * get() const
Access to the underlying object.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
Handle to Geant4 actions with built-in creation mechanism.
KernelHandle worker()
Access to worker thread.
Geant4Handle(T *typ)
Cross type initialization.
~Geant4Handle()
Default destructor.
Geant4Kernel * value
Pointer to referenced object.
~KernelHandle()
Default destructor.
Geant4Handle & operator=(const Geant4Handle &handle)
Assignment operator.
Default base class for all Geant 4 actions and derivates thereof.
TYPE * release()
Release the underlying object.
TYPE * operator->() const
Access to the underlying object.
Geant4Handle()=default
Default constructor.
void destroy()
Destroy referenced object (program termination)
Handle to Geant4 actions with built-in creation mechanism.
bool operator!() const
Validity check.
KernelHandle()
Default constructor.
TYPE * get() const
Access to the underlying object.
Namespace for the AIDA detector description toolkit.
void checked_assign(TYPE *p)
Geant4Handle(Geant4Kernel &ctxt, const std::string &type_name, const std::string &detector, bool shared=false)
Constructor only implemented for sensitive objects.
KernelHandle(const KernelHandle &k)
Copy constructor.
void handle(const O *o, const C &c, F pmf)
Geant4Action * action() const
Access to the underlying object.