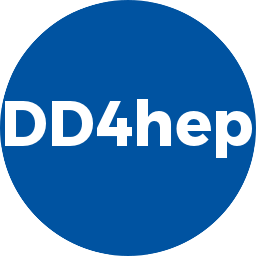 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 string det_name = x_det.nameStr();
27 bool reflect = x_det.reflect();
35 string l_nam = det_name+
_toString(l_num,
"_layer%d");
36 double zmin = x_layer.inner_z();
37 double rmin = x_layer.inner_r();
38 double rmax = x_layer.outer_r();
39 double z = zmin, layerWidth = 0.;
44 layerWidth += thickness;
46 Tube l_tub(rmin,rmax,layerWidth,2*
M_PI);
47 Volume l_vol(l_nam,l_tub,air);
48 l_vol.setVisAttributes(description,x_layer.visStr());
51 double thick = x_slice.thickness();
53 string s_nam = l_nam+
_toString(s_num,
"_slice%d");
54 Volume s_vol(s_nam,
Tube(rmin,rmax,thick), mat);
56 if ( x_slice.isSensitive() ) {
58 s_vol.setSensitiveDetector(sens);
60 s_vol.setAttributes(description,x_slice.regionStr(),x_slice.limitsStr(),x_slice.visStr());
61 pv = l_vol.placeVolume(s_vol,
Position(0,0,z-zmin-layerWidth/2+thick/2));
66 pv = assembly.placeVolume(l_vol,
Position(0,0,zmin+layerWidth/2.));
69 layer.setPlacement(pv);
78 if ( x_det.hasAttr(
_U(combineHits)) ) {
83 sdet.setPlacement(pv);
Class to support the access to collections of XmlNodes (or XmlElements)
Handle class to hold the information of a sensitive detector.
#define DECLARE_DETELEMENT(name, func)
Handle class holding a placed volume (also called physical volume)
DetElement clone(int flag) const
Clone (Deep copy) the DetElement structure.
PlacedVolume & addPhysVolID(const std::string &name, int value)
Add identifier.
std::string _toString(bool value)
String conversions: boolean value to string.
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
DetElement & add(DetElement sub_element)
Add new child to the detector structure.
Class to easily access the properties of single XmlElements.
DetElement & setCombineHits(bool value, SensitiveDetector &sens)
Setter: Combine hits attribute.
Implementation class extending the ROOT assembly volumes (TGeoVolumeAssembly)
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
dd4hep::xml::Component xml_comp_t
Handle class describing a material.
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
Handle class describing a detector element.
virtual Material air() const =0
Return handle to material describing air.
Handle class holding a placed volume (also called physical volume)
DD4hep internal namespace.
dd4hep::xml::DetElement xml_det_t
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
ROOT::Math::Transform3D Transform3D
ROOT::Math::XYZVector Position
ROOT::Math::RotationY RotationY
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
Class describing a tube shape of a section of a tube.
SensitiveDetector & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).