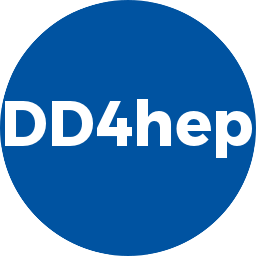 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #ifndef DD4HEP_GRAMMAR_H
22 #define DD4HEP_GRAMMAR_H
40 class GrammarRegistry;
76 void (*
bind)(
void* pointer) = 0;
78 void (*
copy)(
void* to,
const void* from) = 0;
123 static void invalidConversion(
const std::type_info& from,
const std::type_info& to);
125 static void invalidConversion(
const std::string& value,
const std::type_info& to);
144 virtual const Cast&
cast()
const;
146 virtual bool equals(
const std::type_info& other_type)
const = 0;
148 virtual const std::type_info&
type()
const = 0;
154 virtual std::string
str(
const void* ptr)
const;
156 virtual bool fromString(
void* ptr,
const std::string& value)
const;
158 virtual int evaluate(
void* ptr,
const std::string& value)
const;
180 virtual const std::type_info&
type()
const override;
183 virtual bool equals(
const std::type_info& other_type)
const override;
185 virtual size_t sizeOf()
const override;
187 virtual void destruct(
void* pointer)
const override;
189 template <
typename... Args>
void construct(
void* pointer, Args... args)
const;
209 return other_type ==
typeid(TYPE);
219 TYPE* obj = (TYPE*)pointer;
224 template <
typename TYPE>
template <
typename... Args>
226 new(pointer) TYPE(std::forward<Args>(args)...);
239 template <
typename T>
static std::string
str(
const BasicGrammar&,
const void*) {
return ""; }
254 spec.
bind = detail::constructObject<T>;
255 spec.
copy = detail::copyObject<T>;
256 spec.
str = GrammarRegistry::str<T>;
257 return pre_note_specs<T>(spec);
260 return pre_note_specs<T>({});
264 #endif // DD4HEP_GRAMMAR_H
static void pre_note(const std::type_info &info, const BasicGrammar &(*fcn)(), specialization_t specs)
Instance factory.
const std::string name
Instance type name.
unsigned long long int key_type
key_type hash() const
Access the hash value for this grammar type.
virtual int evaluate(void *ptr, const std::string &value) const
Evaluate string value if possible before calling boost::spirit.
virtual size_t sizeOf() const =0
Access the object size (sizeof operator)
virtual bool equals(const std::type_info &other_type) const =0
Access to the type information.
static const GrammarRegistry & pre_note()
std::size_t info(const std::string &src, const std::string &msg)
bool operator==(const specialization_t ©) const
Equality operator.
virtual bool equals(const std::type_info &other_type) const override
Access to the type information.
const key_type hash_value
Instance hash code.
TClass * initialized_clazz() const
Access the ROOT class for complex objects.
BasicGrammar(const std::string &typ)
Default constructor.
void(* copy)(void *to, const void *from)=0
Opaque copy constructor.
Concrete type dependent grammar definition.
virtual std::string str(const void *ptr) const
Serialize an opaque value to a string.
virtual ~Grammar()
Default destructor.
virtual bool fromString(void *ptr, const std::string &value) const
Set value from serialized string. On successful data conversion TRUE is returned.
void construct(void *pointer, Args... args) const
Bind opaque address to object.
int data_type() const
Access ROOT data type for fundamentals.
specialization_t(const specialization_t ©)=default
Copy constructor.
specialization_t(specialization_t &©)=default
Move constructor.
const std::string & type_name() const
Access to the type information name.
static const BasicGrammar & instance()
Instance factory.
specialization_t & operator=(const specialization_t ©)=default
Copy assignment.
bool(* fromString)(const BasicGrammar &gr, void *ptr, const std::string &value)=0
PropertyGrammar overload: Retrieve value from string.
virtual size_t sizeOf() const override
Access the object size (sizeof operator)
TClass * root_class
Cached TClass reference for speed improvements.
static std::string str(const BasicGrammar &, const void *)
PropertyGrammar overload: Serialize a property to a string.
Base class describing string evaluation to C++ objects using boost::spirit.
Grammar registry interface.
const Cast * cast
Ponter to ABI Cast structure.
static const BasicGrammar & get(const std::type_info &info)
Access grammar by type info.
static const GrammarRegistry & pre_note(int)
virtual const std::type_info & type() const override
PropertyGrammar overload: Access to the type information.
GrammarRegistry()=default
Default constructor.
void(* bind)(void *pointer)=0
Bind opaque address to object.
specialization_t & operator=(specialization_t &©)=default
Move assignment.
virtual void setCast(const Cast *cast) const
Set cast structure.
std::string(* str)(const BasicGrammar &gr, const void *ptr)=0
PropertyGrammar overload: Serialize a property to a string.
bool inited
Initialization flag.
struct dd4hep::BasicGrammar::specialization_t specialization
specialization_t()=default
Default constructor.
int initialized_data_type() const
Access ROOT data type for fundamentals.
virtual void destruct(void *pointer) const override
Opaque object destructor.
virtual const std::type_info & type() const =0
Access to the type information.
TClass * clazz() const
Access the ROOT class for complex objects.
Namespace for the AIDA detector description toolkit.
int root_data_type
Cached TDataType information for fundamental types.
virtual const Cast & cast() const
Access ABI object cast.
void initialize() const
Second step initialization after the virtual table is fixed.
Structure to be filled if automatic object parsing from string is supposed to be supported.
virtual void destruct(void *pointer) const =0
Opaque object destructor.
static void invalidConversion(const std::type_info &from, const std::type_info &to)
Error callback on invalid conversion.
virtual ~BasicGrammar()
Default destructor.
Grammar()
Standard constructor.
static const GrammarRegistry & instance()
Registry instance singleton.
static const GrammarRegistry & pre_note_specs(BasicGrammar::specialization_t specs)