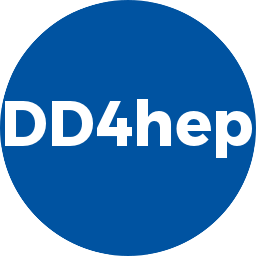 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
22 #include <Math/Polar2D.h>
30 : description(dsc), x_det(x_parent), sensitive(sd)
45 std::string nam = x_c.nameStr();
46 std::string val = x_c.valueStr();
55 auto is =
shapes.find(nam);
56 if ( is ==
shapes.end() ) {
60 except(
"VolumeBuilder",
"+++ Shape %s is already known to this builder unit. ",nam.c_str());
67 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
68 "+++ Register volume: %-20s shape:%-24s vis:%s sensitive:%s",
76 except(
"VolumeBuilder",
"+++ Volume %s is already known to this builder unit. ",nam.c_str());
88 except(
"VolumeBuilder",
"+++ Volume %s is not known to this builder unit. ",nam.c_str());
90 Volume vol = (*iv).second.second;
92 except(
"VolumeBuilder",
"+++ Failed to access volume %s from the local cache.",nam.c_str());
101 except(
"VolumeBuilder",
"+++ Tranformation %s is not known to this builder unit. ",nam.c_str());
103 return (*it).second.second;
108 auto is =
shapes.find(nam);
109 if ( is ==
shapes.end() ) {
115 except(
"VolumeBuilder",
"+++ Shape %s is not known to this builder unit. ",nam.c_str());
117 Solid solid = (*is).second.second;
119 except(
"VolumeBuilder",
"+++ Failed to access shape %s from the local cache.",nam.c_str());
130 nam =
handle.attr<std::string>(a);
131 auto is =
shapes.find(nam);
132 if ( is !=
shapes.end() ) {
133 except(
"VolumeBuilder",
"+++ The named shape %s is already known to this builder unit. "
134 "Cannot be overridden.",nam.c_str());
138 if ( !nam.empty() ) {
147 std::string build =
handle.attr<std::string>(a);
149 printout(INFO,
"VolumeBuilder",
150 "+++ Shape %s does NOT match build requirements. [Ignored]",nam.c_str());
156 std::string type = x.attr<std::string>(
_U(type));
158 if ( !solid.isValid() ) {
159 except(
"VolumeBuilder",
"+++ Failed to create shape %s of type: %s",
160 nam.c_str(), type.c_str());
163 if ( !nam.empty() ) {
167 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
168 "+++ Created shape of type: %s name: %s",type.c_str(), nam.c_str());
174 std::size_t len =
shapes.size();
177 std::string nam = x.
attr<std::string>(
_U(
name));
178 auto is =
shapes.find(nam);
179 if ( is ==
shapes.end() ) {
183 std::string build = c.attr<std::string>(x_build);
185 printout(INFO,
"VolumeBuilder",
186 "+++ Shape %s does NOT match build requirements. [Ignored]",nam.c_str());
191 std::string type = x.
attr<std::string>(
_U(type));
193 if ( !solid.isValid() ) {
194 except(
"VolumeBuilder",
"+++ Failed to create shape %s of type: %s",
195 nam.c_str(), type.c_str());
197 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
198 "+++ Building shape from XML: %s of type: %s",
199 nam.c_str(), solid->IsA()->GetName());
200 shapes.emplace(nam,std::make_pair(c,solid));
203 except(
"VolumeBuilder",
"+++ Shape %s is already known to this builder unit. "
204 "Cannot be overridden.",nam.c_str());
211 std::size_t len =
volumes.size();
216 std::string nam = x.attr<std::string>(
_U(
name));
220 std::string build = c.attr<std::string>(attr);
222 printout(INFO,
"VolumeBuilder",
223 "+++ Volume %s does NOT match build requirements. [Ignored]",nam.c_str());
229 if ( (attr=c.attr_nothrow(
_U(type))) ) {
230 std::string typ = c.attr<std::string>(attr);
232 vol.setAttributes(
description,x.regionStr(),x.limitsStr(),x.visStr());
233 volumes.emplace(nam,std::make_pair(c,vol));
235 if ( is_sensitive ) {
239 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
240 "+++ Building volume from XML: %-20s shape:%-24s vis:%s sensitive:%s",
241 nam.c_str(), solid->IsA()->GetName(), x.visStr().c_str(),
242 yes_no(is_sensitive));
248 if ( (attr=c.attr_nothrow(
_U(shape))) ) {
249 std::string ref = c.attr<std::string>(attr);
250 if ( !(solid=
getShape(ref)).isValid() )
continue;
253 else if ( (x_comp=x.
child(
_U(shape),
false)) ) {
254 if ( !(solid=
makeShape(x_comp)).isValid() )
continue;
260 Volume vol(nam, solid, mat);
263 volumes.emplace(nam,std::make_pair(c,vol));
265 if ( is_sensitive ) {
268 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
269 "+++ Building volume from XML: %-20s shape:%-24s vis:%s sensitive:%s",
270 nam.c_str(), solid->IsA()->GetName(), x.visStr().c_str(),
271 yes_no(is_sensitive));
275 bool is_assembly =
true;
276 is_assembly |= x.child(
_U(assembly),
false) != 0;
277 is_assembly |= c.attr_nothrow(
_U(assembly)) != 0;
282 volumes.emplace(nam,std::make_pair(c,vol));
283 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
284 "+++ Building assembly from XML: %-20s shape:%-24s vis:%s",
285 nam.c_str(), vol->GetShape()->IsA()->GetName(), x.visStr().c_str());
289 except(
"VolumeBuilder",
"+++ Failed to create volume %s - "
290 "It is neither Volume nor assembly....", nam.c_str());
302 except(
"VolumeBuilder",
"+++ The xml volume element has no 'logvol' or 'volume' attribute!");
304 std::string nam = c.
attr<std::string>(attr);
310 except(
"VolumeBuilder",
311 "+++ Failed to locate volume %s [typo somewhere in the XML?]",
315 Volume daughter = (*iv).second.second;
318 std::string tr_nam = c.
attr<std::string>(attr);
321 except(
"VolumeBuilder",
322 "+++ Failed to locate name transformation %s [typo in the XML?]",
334 std::string phys_nam = c.
attr<std::string>(attr_nam);
335 pv->SetName(phys_nam.c_str());
338 if ( attr && !parent.
isValid() ) {
339 except(
"VolumeBuilder",
340 "+++ Failed to set DetElement placement for volume %s [Invalid parent]",
344 int elt_id = parent.
id();
345 std::string elt = c.
attr<std::string>(attr);
348 elt_id = c.
attr<
int>(attr);
349 elt += c.
attr<std::string>(attr);
369 except(
"VolumeBuilder",
"+++ The xml volume element has no 'logvol' or 'volume' attribute!");
371 std::string nam = x_phys.
attr<std::string>(attr);
377 except(
"VolumeBuilder",
378 "+++ Failed to locate volume %s [typo somewhere in the XML?]",
382 if ( attr_elt && !parent.
isValid() ) {
383 except(
"VolumeBuilder",
384 "+++ Failed to set DetElement placement for volume %s [Invalid parent]",
387 Volume daughter = (*iv).second.second;
391 std::string tr_nam = c.
attr<std::string>(attr_tr);
394 except(
"VolumeBuilder",
395 "+++ Failed to locate name transformation %s "
396 "[typo somewhere in the XML?]",
399 tr = (*it).second.second;
406 std::string elt, phys_nam;
409 phys_nam = x_phys.
attr<std::string>(
_U(
name))+
"_%d";
412 elt_id = parent.
id();
413 elt = c.
attr<std::string>(attr_elt);
415 int number = c.
attr<
int>(
_U(number));
416 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
"+++ Mother:%s place volume %s %d times.",
417 vol.
name(), daughter.
name(), number);
418 for(
int i=0; i<number; ++i) {
431 transformation *= tr;
437 std::size_t count = 0;
439 std::string ref = c.attr<std::string>(
_U(ref));
441 xml_h vols = doc->root();
442 printout(
debug ? ALWAYS : DEBUG,
"VolumeBuilder",
443 "++ Processing xml document %s.", doc->uri().c_str());
444 included_docs[ref] = std::unique_ptr<xml::DocumentHolder>(doc.release());
496 else if ( x_pos && x_rot ) {
Class to support the access to collections of XmlNodes (or XmlElements)
const Volume & setLimitSet(const Detector &description, const std::string &name) const
Set the limits to the volume. Note: If the name string is empty, the action is ignored.
Handle class to hold the information of a sensitive detector.
Handle class holding a placed volume (also called physical volume)
Solid solid() const
Access to Solid (Shape)
bool isValid() const
Check the validity of the object held by the handle.
const Volume & setRegion(const Detector &description, const std::string &name) const
Set the regional attributes to the volume. Note: If the name string is empty, the action is ignored.
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
Class to easily access the properties of single XmlElements.
bool isSensitive() const
Accessor if volume is sensitive (ie. is attached to a sensitive detector)
const char * name() const
Access the object name (or "" if not supported by the object)
Implementation class extending the ROOT assembly volumes (TGeoVolumeAssembly)
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
dd4hep::xml::Attribute xml_attr_t
dd4hep::xml::Component xml_comp_t
Handle class describing a material.
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
const Volume & setSensitiveDetector(const SensitiveDetector &obj) const
Assign the sensitive detector structure.
const XmlChar * attr_value(const Attribute attr) const
Access attribute value by the attribute (throws exception if not present)
Handle_t child(const Strng_t &tag_value, bool except=true) const
Access child by tag name. Thow an exception if required in case the child is not present.
Solid createShape(Detector &description, const std::string &shape_type, xml::Element element)
Create a solid shape using the plugin mechanism from the attributes of the XML element.
dd4hep::xml::Strng_t Unicode
bool buildMatch(const std::string &value, DetectorBuildType match)
Check if a build type matches the current.
Class supporting the basic functionality of an XML document including ownership.
Handle_t child(const XmlChar *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
ROOT::Math::Transform3D Transform3D
dd4hep::xml::Handle_t xml_h
ROOT::Math::XYZVector Position
Volume createVolume(Detector &description, const std::string &type, xml::Element element)
Create a volume using the plugin mechanism from the attributes of the XML element.
virtual DetectorBuildType buildType() const =0
Access flag to steer the detail of building of the geometry/detector description.
Class supporting to read and parse XML documents.
User abstraction class to manipulate XML elements within a document.
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Solid_type< TGeoShape > Solid
The main interface to the dd4hep detector description package.
int id() const
Get the detector identifier.
Transform3D createTransformation(xml::Element element)
Create layered transformation from xml information.
const Volume & setAttributes(const Detector &description, const std::string ®ion, const std::string &limits, const std::string &vis) const
Attach attributes to the volume.
VisAttr visAttributes() const
Access the visualisation attributes.
T attr(const Attribute a) const
Access typed attribute value by the XmlAttr.
dd4hep::xml::Dimension xml_dim_t
T attr(const XmlAttr *att) const
Access attribute with implicit return type conversion.
void handle(const O *o, const C &c, F pmf)
Attribute attr_nothrow(const XmlChar *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)