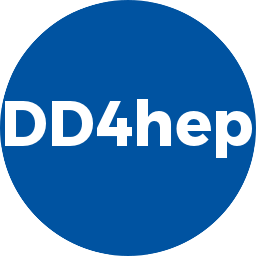 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <Math/Polar2D.h>
27 using dd4hep::printout;
30 static constexpr
const char* TAG_LIMITSETREF =
"limitsetref";
31 static constexpr
const char* TAG_REGIONREF =
"regionref";
32 static constexpr
const char* TAG_VISREF =
"visref";
34 static constexpr
const char* TAG_COMBINE_HITS =
"combine_hits";
35 static constexpr
const char* TAG_VERBOSE =
"verbose";
36 static constexpr
const char* TAG_TYPE =
"type";
37 static constexpr
const char* TAG_ECUT =
"ecut";
38 static constexpr
const char* TAG_HITS_COLLECTION =
"hits_collection";
46 std::string tag = c.tag();
48 if ( tag ==
"positionRPhiZ" ) {
49 if ( flag == 1 ) result = position * result;
50 else if ( flag == 2 ) result = (position * rotation) * result;
56 else if ( tag ==
"position" ) {
57 if ( flag == 1 ) result = position * result;
58 else if ( flag == 2 ) result = (position * rotation) * result;
59 Position pos(x_elt.x(0), x_elt.y(0), x_elt.z(0));
64 else if ( tag ==
"transformation" ) {
65 if ( flag == 1 ) result = position * result;
66 else if ( flag == 2 ) result = (position * rotation) * result;
68 result = trafo * result;
73 else if ( tag ==
"rotation" ) {
78 if ( flag == 1 ) result = position * result;
79 else if ( flag == 2 ) result = (position * rotation) * result;
85 const std::string& shape_type,
87 std::string fac = shape_type +
"__shape_constructor";
89 Solid solid =
Solid(PluginService::Create<TObject*>(fac, &description, &solid_elt));
92 PluginService::Create<TObject*>(fac, &description, &solid_elt);
93 dd4hep::except(
"xml::createShape",
"Failed to create solid of type %s [%s]",
103 std::string typ, tag = elt.tag();
104 PrintLevel lvl = ALWAYS;
107 printout(lvl,
"xml::createStdVolume",
"++ Processing tag: %-12s", tag.c_str());
108 if ( elt.hasAttr(
_U(material)) ) {
112 vol =
Volume(
"volume", sol, mat);
113 if ( elt.hasAttr(
_U(name)) ) vol->SetName(elt.nameStr().c_str());
114 vol.
setAttributes(description,elt.regionStr(),elt.limitsStr(),elt.visStr());
115 printout(lvl,
"xml::createStdVolume",
"++ --> name: %s vis: %s region: %s limits: %s",
117 elt.visStr(
"").c_str(),
118 elt.regionStr(
"").c_str(),
119 elt.limitsStr(
"").c_str());
120 elt = std::move(x_s);
122 else if ( elt.hasAttr(
_U(type)) ) {
124 if ( typ ==
"CAD_Assembly" || typ ==
"CAD_MultiVolume" || typ ==
"GenVolume" )
126 else if ( typ.substr(1) ==
"ssembly" )
128 if ( elt.hasAttr(
_U(name)) ) vol->SetName(elt.nameStr().c_str());
129 vol.
setAttributes(description,elt.regionStr(),elt.limitsStr(),elt.visStr());
130 printout(lvl,
"xml::createStdVolume",
"++ --> name: %s vis: %s region: %s limits: %s",
132 elt.visStr(
"").c_str(),
133 elt.regionStr(
"").c_str(),
134 elt.limitsStr(
"").c_str());
155 if ( xvol && ((xvol.hasAttr(
_U(material)) && xshap) || xvol.hasAttr(
_U(type))) ) {
161 if ( x_rot ) rot =
RotationZYX( x_rot.z(0),x_rot.y(0),x_rot.x(0) );
162 if ( x_pos ) pos =
Position( x_pos.x(0),x_pos.y(0),x_pos.z(0) );
178 if ( xshap && xshap.hasAttr(
_U(type)) ) {
184 if ( x_rot ) rot =
RotationZYX( x_rot.z(0),x_rot.y(0),x_rot.x(0) );
185 if ( x_pos ) pos =
Position( x_pos.x(0),x_pos.y(0),x_pos.z(0) );
192 dd4hep::except(
"xml::createStdVolume",
"Failed to create volume. No material specified!");
198 const std::string& typ,
200 if ( !typ.empty() ) {
202 std::string fac = typ +
"__volume_constructor";
204 TObject* obj = PluginService::Create<TObject*>(fac, &description, &elt);
208 PluginService::Create<TObject*>(fac, &description, &elt);
209 except(
"xml::createShape",
"Failed to create volume of type %s [%s]",
212 if ( e.hasAttr(
_U(name)) ) vol->SetName(e.attr<std::string>(
_U(name)).c_str());
213 vol.
setAttributes(description,e.regionStr(),e.limitsStr(),e.visStr());
216 dd4hep::except(
"xml::createVolume",
"Failed to create volume. No materiaWNo type specified!");
225 std::string det_name = x_det.nameStr();
235 if( x_env.hasChild(
_U(position) ) ) {
238 pos =
Position( env_pos.x(),env_pos.y(),env_pos.z() );
240 if( x_env.hasChild(
_U(rotation) ) ) {
243 rot =
RotationZYX( env_rot.z(),env_rot.y(),env_rot.x() );
247 if( x_shape.typeStr() ==
"Assembly" ){
248 envelope =
Assembly( det_name+
"_assembly" );
254 dd4hep::except(
"createPlacedEnvelope",
255 "Cannot create envelope volume : %s for detector %s.",
256 x_shape.typeStr().c_str(), det_name.c_str());
259 envelope =
Volume( det_name+
"_envelope", env_solid, env_mat );
267 if( useRot && usePos ){
280 envelope.
setAttributes( description,x_det.regionStr(),x_det.limitsStr(),x_env.visStr());
286 std::string det_name = x_det.nameStr();
290 unsigned int typeFlag = x_dettype.type();
291 printout(DEBUG,
"Utilities",
"+++ setDetectorTypeFlags for detector: %s set to 0x%x", det_name.c_str(), typeFlag );
294 catch(
const std::runtime_error& err) {
295 printout(INFO,
"Utilities",
296 "+++ setDetectorTypeFlags for detector: %s not set.",
298 printout(DEBUG,
"Utilities",
299 "+++ setDetectorTypeFlags encountered an error:\n%s", err.what());
304 template <
typename TYPE>
305 std::size_t _propagate(
bool debug,
306 bool apply_to_children,
310 std::size_t count = 0;
311 if ( !vol->IsAssembly() ) {
312 printout(
debug ? dd4hep::ALWAYS : dd4hep::DEBUG,
"VolumeConfig",
"++ Volume: %s apply setting %s", vol.
name(), item.name());
316 if ( apply_to_children ) {
317 std::set<dd4hep::Volume> handled;
318 for (Int_t idau = 0, ndau = vol->GetNdaughters(); idau < ndau; ++idau) {
320 if ( handled.find(
v) == handled.end() ) {
322 count += _propagate(
debug, apply_to_children,
v, item, apply);
337 std::size_t count = 0;
340 bool debug = x_dbg ? element.
attr<
bool>(x_dbg) :
false;
341 PrintLevel lvl =
debug ? ALWAYS : DEBUG;
342 for(
xml_coll_t coll(element,
"*"); coll; coll++ ) {
344 std::string nam = itm.nameStr(
"UN-NAMED");
346 if ( itm.tag() == TAG_REGIONREF ) {
349 printout(lvl,
"VolumeConfig",
"++ %-12s: %-10s REGIONs named '%s'",
350 vol.
name(), region.
isValid() ?
"Set" :
"Invalidate", nam.c_str());
352 else if ( itm.tag() == TAG_LIMITSETREF ) {
355 printout(lvl,
"VolumeConfig",
"++ %-12s: %-10s LIMITSETs named '%s'",
356 vol.
name(), limitset.
isValid() ?
"Set" :
"Invalidate", nam.c_str());
358 else if ( itm.tag() == TAG_VISREF ) {
361 printout(lvl,
"VolumeConfig",
"++ %-12s: %-10s VISATTRs named '%s'",
362 vol.
name(), attrs.
isValid() ?
"Set" :
"Invalidate", nam.c_str());
364 else if ( !ignore_unknown ) {
365 except(
"VolumeConfig",
"++ Unknown Volume property: %s [Ignored]", itm.tag().c_str());
368 printout(DEBUG,
"VolumeConfig",
"++ Unknown Volume property: %s [Ignored]", itm.tag().c_str());
373 except(
"VolumeConfig",
"++ Invalid XML handle to configure DetElement!");
382 std::size_t count = 0;
383 if ( sensitive.
isValid() && element ) {
385 bool debug = x_dbg ? element.
attr<
bool>(x_dbg) :
false;
386 PrintLevel lvl =
debug ? ALWAYS : DEBUG;
388 for(
xml_coll_t coll(element,
"*"); coll; coll++ ) {
390 if ( itm.tag() == TAG_COMBINE_HITS ) {
391 bool value = itm.attr<
bool>(
_U(value));
394 printout(lvl,
"SensDetConfig",
"++ %s Set property 'combine_hits' to %s",
395 sensitive.
name(), true_false(value));
397 else if ( itm.tag() == TAG_VERBOSE ) {
398 bool value = itm.attr<
bool>(
_U(value));
401 printout(lvl,
"SensDetConfig",
"++ %s Set property 'verbose' to %s",
402 sensitive.
name(), true_false(value));
404 else if ( itm.tag() == TAG_TYPE ) {
405 std::string value = itm.valueStr();
408 printout(lvl,
"SensDetConfig",
"++ %s Set property 'type' to %s",
409 sensitive.
name(), value.c_str());
411 else if ( itm.tag() == TAG_ECUT ) {
412 double value = itm.attr<
double>(
_U(value));
415 printout(lvl,
"SensDetConfig",
"++ %s Set property 'ecut' to %f",
416 sensitive.
name(), value);
418 else if ( itm.tag() == TAG_HITS_COLLECTION ) {
421 printout(lvl,
"SensDetConfig",
"++ %s Set property 'hits_collection' to %s",
422 sensitive.
name(), itm.valueStr().c_str());
425 except(
"SensDetConfig",
426 "++ Unknown Sensitive Detector property: %s [Failure]",
432 except(
"SensDetConfig",
433 "FAILED: No valid sensitive detector. Configuration could not be applied!");
std::size_t configVolume(dd4hep::Detector &detector, dd4hep::xml::Handle_t element, dd4hep::Volume volume, bool propagate, bool ignore_unknown_attr=false)
Configure volume properties from XML element.
Class to support the access to collections of XmlNodes (or XmlElements)
const Volume & setLimitSet(const Detector &description, const std::string &name) const
Set the limits to the volume. Note: If the name string is empty, the action is ignored.
SensitiveDetector & setEnergyCutoff(double value)
Set energy cut off.
Handle class to hold the information of a sensitive detector.
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
const XmlAttr * Attribute
SensitiveDetector & setHitsCollection(const std::string &spec)
Assign the name of the hits collection.
SensitiveDetector & setVerbose(bool value)
Set flag to handle hits collection.
PlacedVolume & addPhysVolID(const std::string &name, int value)
Add identifier.
std::size_t debug(const std::string &src, const std::string &msg)
bool isValid() const
Check the validity of the object held by the handle.
virtual Region region(const std::string &name) const =0
Retrieve a region object by its name from the detector description.
const Volume & setRegion(const Detector &description, const std::string &name) const
Set the regional attributes to the volume. Note: If the name string is empty, the action is ignored.
ROOT::Math::Rotation3D Rotation3D
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
Class to easily access the properties of single XmlElements.
const char * name() const
Access the object name (or "" if not supported by the object)
Implementation class extending the ROOT assembly volumes (TGeoVolumeAssembly)
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
dd4hep::xml::Component xml_comp_t
Handle class describing a material.
DetElement & setTypeFlag(unsigned int types)
Set the flag word encoding detector types ( ideally use dd4hep::DetType for encoding )
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
Handle class describing a set of limits as they are used for simulation.
Solid createShape(Detector &description, const std::string &shape_type, xml::Element element)
Create a solid shape using the plugin mechanism from the attributes of the XML element.
dd4hep::xml::DetElement xml_det_t
Handle class describing a region as used in simulation.
Helper to debug plugin manager calls.
ROOT::Math::RhoZPhiVector PositionRhoZPhi
Helper class to encapsulate a unicode string.
virtual LimitSet limitSet(const std::string &name) const =0
Retrieve a limitset by its name from the detector description.
std::string missingFactory(const std::string &name) const
Helper to check factory existence.
void setDetectorTypeFlag(dd4hep::xml::Handle_t e, dd4hep::DetElement sdet)
Handle_t child(const XmlChar *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
virtual const HandleMap & visAttributes() const =0
Accessor to the map of visualisation attributes.
ROOT::Math::Transform3D Transform3D
ROOT::Math::XYZVector Position
Volume createPlacedEnvelope(dd4hep::Detector &description, dd4hep::xml::Handle_t e, dd4hep::DetElement sdet)
Volume createVolume(Detector &description, const std::string &type, xml::Element element)
Create a volume using the plugin mechanism from the attributes of the XML element.
Class describing a box shape.
T * ptr() const
Access to the held object.
User abstraction class to manipulate XML elements within a document.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Solid_type< TGeoShape > Solid
The main interface to the dd4hep detector description package.
int id() const
Get the detector identifier.
Transform3D createTransformation(xml::Element element)
Create layered transformation from xml information.
SensitiveDetector & setCombineHits(bool value)
Set flag to handle hits collection.
ROOT::Math::RotationZYX RotationZYX
const Volume & setAttributes(const Detector &description, const std::string ®ion, const std::string &limits, const std::string &vis) const
Attach attributes to the volume.
Volume createStdVolume(Detector &description, xml::Element element)
Create a simple volume using the shape plugin mechanism from the attributes of the XML element.
T attr(const Attribute a) const
Access typed attribute value by the XmlAttr.
dd4hep::xml::Dimension xml_dim_t
SensitiveDetector & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).
std::size_t configSensitiveDetector(dd4hep::Detector &detector, dd4hep::SensitiveDetector sensitive, dd4hep::xml::Handle_t element)
Configure sensitive detector from XML element.
Attribute attr_nothrow(const XmlChar *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)