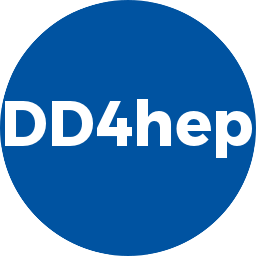 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
28 string name = x_det.nameStr();
29 Tube tub (x_tube.rmin(),x_tube.rmax(),x_tube.zhalf());
32 vol.setVisAttributes(description, x_det.visStr());
33 vol.setLimitSet(description, x_det.limitsStr());
34 vol.setRegion(description, x_det.regionStr());
35 if ( x_det.isSensitive() ) {
38 vol.setSensitiveDetector(sens);
46 if ( x_det.hasAttr(
_U(
id)) ) {
49 sdet.setPlacement(phv);
Handle class to hold the information of a sensitive detector.
#define DECLARE_DETELEMENT(name, func)
Handle class holding a placed volume (also called physical volume)
PlacedVolume & addPhysVolID(const std::string &name, int value)
Add identifier.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
Class to easily access the properties of single XmlElements.
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
dd4hep::xml::Component xml_comp_t
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
DD4hep internal namespace.
dd4hep::xml::DetElement xml_det_t
ROOT::Math::Transform3D Transform3D
ROOT::Math::XYZVector Position
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
ROOT::Math::RotationZYX RotationZYX
Class describing a tube shape of a section of a tube.
dd4hep::xml::Dimension xml_dim_t
SensitiveDetector & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).