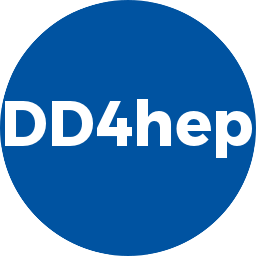 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETECTORIMP_H
14 #define DD4HEP_DETECTORIMP_H
26 #if !defined(DD4HEP_MUST_USE_DETECTORIMP_H) && !defined(G__ROOT)
27 #error "DetectorImp.h is a dd4hep internal header. Never use it in a depending compilation unit!"
46 class OpticalSurfaceManagerObject;
90 Int_t
saveObject(
const char *name=0, Int_t option=0, Int_t bufsize=0)
const;
122 virtual void fromXML(
const std::string& fname,
126 virtual void dump()
const override;
129 virtual long apply(
const char* factory,
int argc,
char** argv)
const override;
132 virtual void init()
override;
135 virtual void endDocument(
bool close_geometry)
override;
144 virtual void*
userExtension(
unsigned long long int key,
bool alert=
true)
const override;
155 virtual TGeoManager&
manager()
const override {
229 virtual std::string
constantAsString(
const std::string& name)
const override;
232 virtual long constantAsLong(
const std::string& name)
const override;
321 virtual const std::vector<DetElement>&
detectors(
const std::string& type,
bool throw_exc)
const override;
324 virtual std::vector<DetElement>
detectors(
const std::string& type1,
325 const std::string& type2,
326 const std::string& type3=
"",
327 const std::string& type4=
"",
328 const std::string& type5=
"" )
override;
331 virtual std::vector<std::string>
detectorTypes()
const override;
336 virtual std::vector<DetElement>
detectors(
unsigned int includeFlag,
337 unsigned int excludeFlag=0 )
const override;
340 #define __R return *this
416 virtual Int_t
Write(
const char *name=0, Int_t option=0, Int_t bufsize=0)
override {
421 virtual Int_t
Write(
const char *name=0, Int_t option=0, Int_t bufsize=0)
const override {
430 #if defined(__CINT__) || defined(__MAKECINT__) || defined(__CLING__) || defined(__ROOTCLING__)
431 #pragma link C++ class dd4hep::DetectorImp+;
433 #endif // DD4HEP_DETECTORIMP_H
virtual void setTrackingVolume(Volume vol) override
Set the tracking volume of the detector.
virtual const HandleMap & detectors() const override
Accessor to the map of sub-detectors.
virtual State state() const override
Access the state of the geometry.
State
The detector description states.
DetectorImp()
Default constructor used by ROOT I/O.
virtual Detector & add(Constant x) override
Add a new constant to the detector description.
This structure describes the internal data of the volume manager object.
ObjectHandleMap m_sensitive
The map of top level sub-detector sensitive detector objects indexed by the detector name.
Definition of the extension entry interface class.
virtual void init() override
Open the geometry at startup.
virtual Volume worldVolume() const override
Return handle to the world volume containing everything.
ObjectHandleMap m_display
The map of display attributes in use.
virtual DetectorBuildType buildType() const override
Access flag to steer the detail of building of the geometry/detector description.
virtual Material air() const override
Return handle to material describing air.
DetectorBuildType m_buildType
VolumeManager m_volManager;.
virtual DetElement trackers() const override
Return reference to detector element with all tracker devices.
Handle class to hold the information of a sensitive detector.
void append(const Handle< NamedObject > &e, bool throw_on_doubles=true)
Append entry to map.
virtual Detector & addReadout(const Handle< NamedObject > &x) override
Add a new detector readout by named reference to the detector description.
std::map< std::string, PropertyValues > Properties
virtual const HandleMap & limitsets() const override
Accessor to the map of limit settings.
DetectorImp & operator=(const DetectorImp ©)=delete
Disable assignment operator.
Handle class describing visualization attributes.
virtual Detector & addConstant(const Handle< NamedObject > &x) override
Add a new constant by named reference to the detector description.
virtual Detector & addField(const Handle< NamedObject > &x) override
Add a field component by named reference to the detector description.
Class implementing the ID encoding of the detector response.
STD_Conditions m_std_conditions
Standard conditions.
virtual const HandleMap & regions() const override
Accessor to the map of region settings.
virtual Detector & addDetector(const Handle< NamedObject > &x) override
Add a new subdetector by named reference to the detector description.
virtual const HandleMap & sensitiveDetectors() const override
Retrieve a sensitive detector by its name from the detector description.
virtual void fromCompact(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT) override
Read compact geometry description or alignment file.
virtual DetElement detector(const std::string &name) const override
Retrieve a subdetector element by its name from the detector description.
virtual Detector & add(LimitSet x) override
Add a new limit set to the detector description.
ObjectHandleMap m_idDict
Map of readout IDDescriptors indexed by hit collection name.
virtual Detector & add(IDDescriptor x) override
Add a new id descriptor to the detector description.
virtual DetElement world() const override
Return reference to the top-most (world) detector element.
virtual Detector & addLimitSet(const Handle< NamedObject > &x) override
Add a new limit set by named reference to the detector description.
Properties & properties() const override
Access to properties.
virtual Detector & addIDSpecification(const Handle< NamedObject > &x) override
Add a new id descriptor by named reference to the detector description.
Class describing a field overlay with several sources.
virtual long constantAsLong(const std::string &name) const override
Typed access to constants: long values.
Handle class describing a material.
virtual void declareParent(const std::string &detector_name, const DetElement &parent) override
Register new mother volume using the detector name.
virtual Detector & addSensitiveDetector(const Handle< NamedObject > &x) override
Add a new sensitive detector by named reference to the detector description.
virtual VisAttr visAttributes(const std::string &name) const override
Retrieve a visualization attribute by its name from the detector description.
DetectorTypeMap m_detectorTypes
Inventory of detector types.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const override
Retrieve a sensitive detector by its name from the detector description.
ObjectHandleMap m_regions
Map of regions settings for the simulation.
virtual const HandleMap & readouts() const override
Accessor to the map of readout structures.
virtual void setHeader(Header h) override
Accessor to the header entry.
virtual TGeoManager & manager() const override
Access the geometry manager of this instance.
virtual VolumeManager volumeManager() const override
Return handle to the VolumeManager.
virtual Volume parallelWorldVolume() const override
Return handle to the world volume containing the volume with the tracking devices.
Helper class to access default temperature and pressure.
virtual Detector & add(Readout x) override
Add a new detector readout to the detector description.
Handle class describing a detector element.
Handle class describing a constant (define) object in description.
Handle class holding a placed volume (also called physical volume)
virtual const HandleMap & fields() const override
Accessor to the map of field entries, which together form the global field.
DetectorImp(DetectorImp &©)=delete
not persistent
Class to support the retrieval of detector elements and volumes given a valid identifier.
virtual ~DetectorImp()
Standard destructor.
Detector::State m_state
Detector description state.
virtual VisAttr invisible() const override
Return handle to "invisible" visualization attributes.
VolumeManager m_volManager
Volume manager reference.
ObjectHandleMap m_readouts
Map of readout descriptors indexed by subdetector name.
Handle class describing a set of limits as they are used for simulation.
virtual void dump() const override
Stupid legacy method.
virtual Detector & add(SensitiveDetector x) override
Add a new sensitive detector to the detector description.
virtual const HandleMap & visAttributes() const override
Accessor to the map of visualisation attributes.
ObjectHandleMap m_detectors
The map of top level sub-detector objects indexed by name.
virtual Constant constant(const std::string &name) const override
Retrieve a constant by its name from the detector description.
virtual long apply(const char *factory, int argc, char **argv) const override
Manipulate geometry using facroy converter.
virtual void * removeUserExtension(unsigned long long int key, bool destroy=true) override
Remove an existing extension object from the Detector instance. If not destroyed, the instance is ret...
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
Int_t saveObject(const char *name=0, Int_t option=0, Int_t bufsize=0) const
ROOT I/O call.
virtual const HandleMap & idSpecifications() const override
Accessor to the map of ID specifications.
DetectorImp(const DetectorImp ©)=delete
Disable copy constructor.
Handle class describing a region as used in simulation.
virtual const HandleMap & constants() const override
Accessor to the map of constants.
virtual IDDescriptor idSpecification(const std::string &name) const override
Retrieve a id descriptor by its name from the detector description.
virtual void endDocument(bool close_geometry) override
Close the geometry.
virtual Material material(const std::string &name) const override
Retrieve a matrial by its name from the detector description.
virtual Detector & add(VisAttr x) override
Add a new visualisation attribute to the detector description.
Data implementation class of the Detector interface.
virtual Volume trackingVolume() const override
Return handle to the world volume containing the volume with the tracking devices.
Detector::Properties m_properties
virtual Detector & addRegion(const Handle< NamedObject > &x) override
Add a new detector region by named reference to the detector description.
ObjectHandleMap m_fields
The map of electro magnet field components for the global overlay field.
virtual const STD_Conditions & stdConditions() const override
Access default conditions (temperature and pressure.
virtual Volume pickMotherVolume(const DetElement &sd) const override
Access mother volume by detector element.
Class supporting to read data given a URI.
std::map< std::string, Handle< NamedObject > > HandleMap
Type definition of a map of named handles.
virtual Region region(const std::string &name) const override
Retrieve a region object by its name from the detector description.
detail::OpticalSurfaceManagerObject * m_surfaceManager
Optical surface manager.
virtual void * userExtension(unsigned long long int key, bool alert=true) const override
Access an existing extension object from the Detector instance.
virtual Detector & add(CartesianField x) override
Add a field component to the detector description.
virtual void * addUserExtension(unsigned long long int key, ExtensionEntry *entry) override
Add an extension object to the Detector instance.
virtual std::string constantAsString(const std::string &name) const override
Typed access to constants: access string values.
virtual Detector & addVisAttribute(const Handle< NamedObject > &x) override
Add a new visualisation attribute by named reference to the detector description.
virtual void fromXML(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT) override
Read any XML file.
Volume m_parallelWorldVol
virtual CartesianField field(const std::string &name) const override
Retrieve a subdetector element by its name from the detector description.
Material m_materialVacuum
std::map< std::string, std::vector< DetElement > > DetectorTypeMap
Cached map with detector types:
virtual Readout readout(const std::string &name) const override
Retrieve a readout object by its name from the detector description.
virtual void setStdConditions(double temp, double pressure) override
Set the STD temperature and pressure.
virtual LimitSet limitSet(const std::string &name) const override
Retrieve a limitset by its name from the detector description.
DetectorBuildType
Detector description build types.
Namespace for the AIDA detector description toolkit.
Data implementation class of the Detector interface.
virtual Material vacuum() const override
Return handle to material describing vacuum.
virtual Int_t Write(const char *name=0, Int_t option=0, Int_t bufsize=0) override
TObject overload: We need to set the Volume and PlacedVolume extensions to be persistent.
void mapDetectorTypes()
Internal helper to map detector types once the geometry is closed.
virtual OpticalSurfaceManager surfaceManager() const override
Access the optical surface manager.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
TGeoManager * m_manager
Reference to the geometry manager object from ROOT.
virtual Int_t Write(const char *name=0, Int_t option=0, Int_t bufsize=0) const override
TObject overload: We need to set the Volume and PlacedVolume extensions to be persistent.
virtual std::vector< std::string > detectorTypes() const override
Access the availible detector types.
virtual Handle< NamedObject > getRefChild(const HandleMap &e, const std::string &name, bool throw_if_not=true) const
virtual Detector & add(Region x) override
Add a new detector region to the detector description.
ClassDefOverride(DetectorImp, 100)
void imp_loadVolumeManager()
Local method (no interface): Load volume manager.
virtual OverlayedField field() const override
Return handle to the combined electromagentic field description.
virtual double constantAsDouble(const std::string &name) const override
Typed access to constants: double values.
Concrete implementation class of the Detector interface.
ObjectHandleMap m_limits
Map of limit sets.
virtual Detector & add(DetElement x) override
Add a new subdetector to the detector description.
virtual Header header() const override
Accessor to the header entry.
Class to support the handling of optical surfaces.
Base class describing any field with 3D cartesian vectors for the field strength.