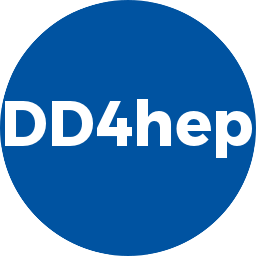 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
50 string name = x_det.nameStr();
56 sensitive.
setType(
"escape_counter");
59 vector<double> rmin,rmax,z;
60 string vis = mod.visStr().empty() ? x_det.visStr() : mod.visStr();
64 rmin.push_back(dim.rmin());
65 rmax.push_back(dim.rmax());
66 z.push_back(dim.z()/2);
69 throw runtime_error(
"ZylinderShell["+name+
"]> Not enough Z planes. minimum is 2!");
72 Volume volume(name, cone, mat);
73 volume.setVisAttributes(description, vis);
74 volume.setSensitiveDetector(sensitive);
75 pv = assembly.placeVolume(volume);
81 sdet.setPlacement(pv);
Class to support the access to collections of XmlNodes (or XmlElements)
Handle class to hold the information of a sensitive detector.
#define DECLARE_DETELEMENT(name, func)
Handle class holding a placed volume (also called physical volume)
PlacedVolume & addPhysVolID(const std::string &name, int value)
Add identifier.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
Class to easily access the properties of single XmlElements.
Implementation class extending the ROOT assembly volumes (TGeoVolumeAssembly)
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
dd4hep::xml::Component xml_comp_t
Handle class describing a material.
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
DD4hep internal namespace.
dd4hep::xml::DetElement xml_det_t
Class describing a Polycone shape.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
SensitiveDetector & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).