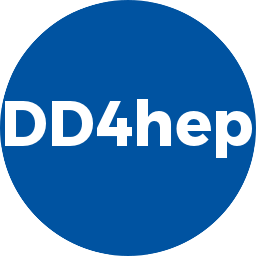 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DD4HEP_CONDITIONS_XMLCONDITONSLOADER_H
15 #define DD4HEP_CONDITIONS_XMLCONDITONSLOADER_H
34 typedef std::vector<Condition>
Buffer;
39 const IOV& req_validity,
48 const IOV& req_validity,
52 const IOV& req_validity,
60 except(
"ConditionsLoader",
"+++ update: Invalid call!");
86 const char* name = argc>0 ? argv[0] :
"XMLLoader";
105 const IOV& req_validity,
108 std::size_t len = conditions.size();
109 std::string fac =
"XMLConditionsParser";
113 char* argv[] = { (
char*)
handle.ptr(), (
char*)&stack, 0};
116 for (ConditionsStack::iterator c=stack.begin(); c!=stack.end(); ++c) {
119 except(
"ConditionsXmlLoader",
"Fix me: queueUpdate(e) not implemented");
122 if (
key == condition->hash ) {
124 conditions.emplace_back(condition);
134 return conditions.size()-len;
138 const IOV& req_validity,
141 std::size_t len = conditions.size();
147 const IOV* iov = condition->
iov;
149 if (
key == condition->hash ) {
150 conditions.emplace_back(condition);
152 return conditions.size()-len;
156 return conditions.size()-len;
160 const IOV& req_validity,
163 std::size_t len = conditions.size();
167 std::vector<Condition> keep;
170 const IOV* iov = condition->
iov;
172 if (
key == condition->hash ) {
173 conditions.emplace_back(condition);
176 keep.emplace_back(condition);
179 return conditions.size()-len;
Condition::key_type key_type
std::vector< Condition > RangeConditions
bool contains(const IOV &iov) const
Check for validity containment.
std::vector< Condition > Buffer
AlignmentCondition::Object * cond
size_t load_source(const std::string &nam, key_type key, const IOV &req_validity, RangeConditions &conditions)
virtual std::size_t load_range(key_type key, const IOV &req_validity, RangeConditions &conditions)
Load a condition set given a Detector Element and the conditions name according to their validity.
Sources m_sources
Property: input data source definitions.
bool isValid() const
Check the validity of the object held by the handle.
Class to easily access the properties of single XmlElements.
virtual std::size_t load_many(const IOV &, RequiredItems &, LoadedItems &, IOV &)
Optimized update using conditions slice data.
Basic conditions manager implementation.
Main condition object handle.
virtual ~ConditionsXmlLoader()
Default destructor.
Namespace for implementation details of the AIDA detector description toolkit.
Implementation of a stack of conditions assembled before application.
void * createPlugin(const std::string &factory, Detector &description, void *(*cast)(void *))
Handler for factories of type: ConstructionFactory.
Class describing the interval of validty.
std::vector< std::pair< key_type, ConditionsLoadInfo * > > RequiredItems
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
ConditionsXmlLoader(Detector &description, ConditionsManager mgr, const std::string &nam)
Default constructor.
Class supporting the basic functionality of an XML document including ownership.
static bool partial_match(const IOV &iov, const IOV &test)
Check if IOV 'test' is of same type and is at least partially contained in iov.
std::list< Entry * > ConditionsStack
Manager class for condition handles.
Interface for a generic conditions loader.
Class supporting to read and parse XML documents.
Detector & m_detector
Reference to main detector description object.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
std::map< key_type, Condition > LoadedItems
const IOV & iov() const
Access the IOV block.
void handle(const O *o, const C &c, F pmf)
virtual std::size_t load_single(key_type key, const IOV &req_validity, RangeConditions &conditions)
Load a condition set given a Detector Element and the conditions name according to their validity.
The intermediate conditions data used to populate the DetElement conditions.
Handle_t root() const
Access the ROOT eleemnt of the DOM document.