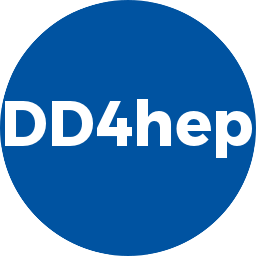 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
35 std::cout <<
" usage: print_materials compact.xml x0 y0 z0 x1 y1 z1 " << std::endl
36 <<
" -> prints the materials on a straight line between the two given points ( unit is cm) "
41 std::string inFile = argv[1] ;
43 std::stringstream sstr ;
44 sstr << argv[2] <<
" " << argv[3] <<
" " << argv[4] <<
" " << argv[5] <<
" " << argv[6] <<
" " << argv[7] ;
46 double x0,y0,z0,x1,y1,z1 ;
54 Detector& description = Detector::getInstance();
65 std::cout << std::endl <<
" ####### materials between the two points : " << p0 <<
"*cm and " << p1 <<
"*cm : " << std::endl ;
68 double sum_lambda = 0 ;
69 double path_length = 0 ;
70 for(
unsigned i=0,n=materials.size();i<n;++i){
73 double length = materials[i].second ;
78 double nLambda = length / mat.
intLength() ;
79 sum_lambda += nLambda ;
81 path_length += length ;
83 std::cout <<
" " << mat <<
" thickness: " << length <<
" path_length:" << path_length <<
" integrated_X0: " << sum_x0 <<
" integrated_lambda: " << sum_lambda << std::endl ;
86 std::cout <<
"############################################################################### " << std::endl << std::endl ;
91 std::cout <<
" averaged Material : " <<
" Z: " << avMat.
Z() <<
" A: " << avMat.
A() <<
" densitiy: " << avMat.
density()
93 <<
" interactionLength: " << avMat.
interactionLength() << std::endl << std::endl ;
96 std::cout <<
" Total length : " << path_length / dd4hep::mm <<
" mm " << std::endl ;
98 std::cout <<
" Integrated radiation lengths : " << path_length / avMat.
radiationLength() <<
" X0 " << std::endl ;
100 std::cout <<
" Integrated interaction lengths : " << path_length / avMat.
interactionLength() <<
" lambda " << std::endl ;
102 std::cout <<
"############################################################################### " << std::endl << std::endl ;
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
Namespace for the reconstruction part of the AIDA detector description toolkit.
virtual double radiationLength() const
radiation length - tgeo units
virtual double density() const
density
MaterialData createAveragedMaterial(const MaterialVec &materials)
virtual double interactionLength() const
interaction length - tgeo units
std::vector< std::pair< Material, double > > MaterialVec
double intLength() const
Access the interaction length of the underlying material.
Handle class describing a material.
DD4hep internal namespace.
Volume volume() const
Access to the logical volume of the detector element's placement.
virtual void fromCompact(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT)=0
Deprecated call (use fromXML): Read compact geometry description or alignment file.
virtual double A() const
averaged atomic number
const MaterialVec & materialsBetween(const Vector3D &p0, const Vector3D &p1, double epsilon=1e-4)
virtual double Z() const
averaged proton number
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
double radLength() const
Access the radiation length of the underlying material.
int main_wrapper(int argc, char **argv)