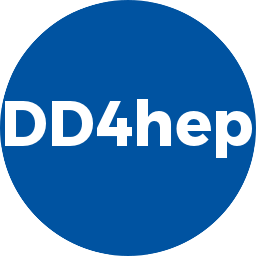 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_SURFACEINSTALLER_H
14 #define DD4HEP_SURFACEINSTALLER_H 1
61 typedef std::map<TGeoVolume*, SurfaceData* >
Surfaces;
98 template <
typename T>
static long run(
Detector& description,
int argc,
char** argv);
103 T installer(description, argc, argv);
108 #define DECLARE_SURFACE_INSTALLER(name,class) \
110 template long SurfaceInstaller::run< class >(Detector& description,int argc,char** argv); \
112 DECLARE_APPLY(name,SurfaceInstaller::run< class >)
117 #if defined(DD4HEP_USE_SURFACEINSTALL_HELPER)
122 #ifndef SURFACEINSTALLER_DATA
155 void handle_arguments(
int argc,
char** argv);
158 Installer() =
delete;
160 Installer(
const Installer&
copy) =
delete;
164 virtual ~Installer() =
default;
173 template <
typename T>
bool checkShape(
const T& shape)
const {
174 if ( shape.isValid() )
return true;
175 invalidInstaller(
"Shape is not of the required type:"+dd4hep::typeName(
typeid(T)));
181 template <
typename UserData>
182 Installer<UserData>::Installer(
dd4hep::Detector& description,
int argc,
char** argv)
183 :
dd4hep::SurfaceInstaller(description, argc, argv), data()
185 handle_arguments(argc, argv);
189 template <
typename UserData>
191 Surfaces::const_iterator is = m_surfaces.find(vol.
ptr());
192 if ( is != m_surfaces.end() ) {
193 VolSurface surf((*is).second);
201 template <
typename UserData>
203 m_surfaces.insert(std::make_pair(surf.
volume().
ptr(),surf.
ptr()));
208 template <
typename UserData>
void Installer<UserData>::handle_arguments(
int,
char**) {}
209 #ifndef SURFACEINSTALLER_DATA
210 template <>
void Installer<SURFACEINSTALLER_DATA>::handle_arguments(
int,
char**) {}
214 typedef Installer<SURFACEINSTALLER_DATA> InstallerClass;
218 #endif // DD4HEP_SURFACEINSTALLER_H
std::map< TGeoVolume *, SurfaceData * > Surfaces
SurfaceInstaller & operator=(const SurfaceInstaller ©)=delete
No assignment.
#define SURFACEINSTALLER_DATA
Handle class holding a placed volume (also called physical volume)
Volume volume() const
the volume to which this surface is attached.
DetElement m_det
Reference to the detector element of the subdetector.
#define DD4HEP_USE_SURFACEINSTALL_HELPER
rec::SurfaceType SurfaceType
virtual void install(DetElement e, PlacedVolume pv)
Install volume information. Default implementation only prints!
VolSurfaceHandle< VolPlaneImpl > VolPlane
VolSurfaceList * volSurfaceList(DetElement &det)
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
void scan()
Scan through tree of detector elements.
SurfaceInstaller()=delete
No default constructor.
Volume parentVolume(DetElement component) const
Shortcut to access the parent detectorelement's volume.
void invalidInstaller(const std::string &msg) const
Indicate error message and throw exception.
static long run(Detector &description, int argc, char **argv)
Executor.
Surfaces m_surfaces
Map of surface instances keyed by the logical volume.
Detector & m_detDesc
Reference to the Detector instance.
detail::tools::PlacementPath PlacementPath
#define DECLARE_SURFACE_INSTALLER(name, class)
const double * placementTranslation(DetElement component) const
Shortcut to access the translation vector of a given component.
detail::tools::ElementPath ElementPath
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
Base class to implement surface installers for known detector patterns.
void stopScanning()
Set flag to stop scanning volumes and detector elements.
virtual ~SurfaceInstaller()=default
Default destructor.
rec::VolSurfaceBase SurfaceData
SurfaceInstaller(const SurfaceInstaller ©)=delete
No copy constructor.
bool m_stopScanning
Flag to inhibit useless further scans.
VolSurfaceBase * ptr() const
pointer to underlying object