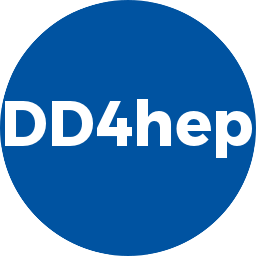 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_MATERIALMANAGER_H
14 #define DDREC_MATERIALMANAGER_H
30 typedef std::vector< std::pair< Material, double > >
MaterialVec;
31 typedef std::vector< std::pair< PlacedVolume, double > >
PlacementVec;
96 os <<
" " << m.
name() <<
" Z: " << m.
Z() <<
" A: " << m.
A() <<
" density: " << m.
density()
98 <<
" interactionLength: " << m.
intLength() ;
104 for(
unsigned i=0,n=m.size() ; i<n ; ++i ) {
105 os <<
" material: " << m[i].first <<
" thickness: " << m[i].second << std::endl ;
115 #endif // DDREC_MATERIALMANAGER_H
const Material & materialAt(const Vector3D &pos)
MaterialVec _mV
Cached materials.
MaterialData createAveragedMaterial(const MaterialVec &materials)
Handle class holding a placed volume (also called physical volume)
std::vector< std::pair< Material, double > > MaterialVec
const char * name() const
Access the object name (or "" if not supported by the object)
double intLength() const
Access the interaction length of the underlying material.
PlacedVolume _pv
Cached nodes.
double A() const
atomic number of the underlying material
double density() const
density of the underlying material
Handle class describing a material.
Handle class holding a placed volume (also called physical volume)
double Z() const
proton number of the underlying material
const PlacementVec & placementsBetween(const Vector3D &p0, const Vector3D &p1, double epsilon=1e-4)
Vector3D _p0
cached last points
PlacedVolume placementAt(const Vector3D &pos)
const MaterialVec & materialsBetween(const Vector3D &p0, const Vector3D &p1, double epsilon=1e-4)
std::vector< std::pair< PlacedVolume, double > > PlacementVec
Namespace for the AIDA detector description toolkit.
std::ostream & operator<<(std::ostream &io, const DCH_info &d)
TGeoManager * _tgeoMgr
Reference to the TGeoManager.
double radLength() const
Access the radiation length of the underlying material.