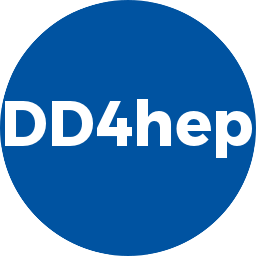 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_VECTOR3D_H
14 #define DDREC_VECTOR3D_H 1
21 namespace dd4hep{
namespace rec {
56 Vector3D(
double x_val,
double y_val,
double z_val ) :
96 inline const Vector3D&
fill(
double x_val,
double y_val,
double z_val) {
97 _x = x_val ;
_y = y_val ;
_z = z_val ;
103 inline double x()
const {
return _x ; }
106 inline double y()
const {
return _y ; }
109 inline double z()
const {
return _z ; }
112 inline double&
x() {
return _x ; }
115 inline double&
y() {
return _y ; }
118 inline double&
z() {
return _z ; }
124 case 0:
return _x ; break ;
125 case 1:
return _y ; break ;
126 case 2:
return _z ; break ;
133 case 0:
return _x ; break ;
134 case 1:
return _y ; break ;
135 case 2:
return _z ; break ;
137 static double dummy(0.0) ;
142 inline double phi()
const {
144 return _x == 0.0 &&
_y == 0.0 ? 0.0 : atan2(
_y,
_x);
148 inline double rho()
const {
166 inline double r()
const {
173 inline double r2()
const {
181 return _x == 0.0 &&
_y == 0.0 &&
_z == 0.0 ? 0.0 : atan2(
rho(),
_z) ;
186 return _x *
v.x() +
_y *
v.y() +
_z *
v.z() ;
195 _x *
v.y() -
_y *
v.x() ) ;
207 inline operator const double*()
const {
296 if( a.
x() == b.
x() && a.
y() == b.
y() && a.
z() == b.
z() )
305 return Vector3D( s *
v.x() , s *
v.y() , s *
v.z() ) ;
316 return v0.
dot( v1 ) ;
336 inline Vector3D::Vector3D(
double rho_val,
double phi_val,
double z_val, Vector3D::Cylindrical (&)() ) : _z(z_val) {
338 _x = rho_val * cos( phi_val ) ;
339 _y = rho_val * sin( phi_val ) ;
347 inline Vector3D::Vector3D(
double r_val,
double phi_val,
double theta_val, Vector3D::Spherical (&)() ) {
348 double rst = r_val * sin( theta_val ) ;
349 _x = rst * cos( phi_val ) ;
350 _y = rst * sin( phi_val ) ;
351 _z = r_val * cos( theta_val ) ;
363 <<
" ) - [ phi: " <<
v.phi()
364 <<
" , rho: " <<
v.rho() <<
" ] "
365 <<
" [ theta: " <<
v.theta()
366 <<
" , r: " <<
v.r() <<
" ] " ;
const Vector3D & fill(const T &v)
fill vector from arbitrary class that defines operator[]
Vector3D operator+(const Vector3D &a, const Vector3D &b)
Vector3D operator*(double s, const Vector3D &v)
double * array()
direct access to data as double* - allows modification
Vector3D operator-(const Vector3D &a, const Vector3D &b)
static Cylindrical cylindrical()
static Cartesian cartesian()
double & operator[](int i)
Vector3D(double x, double y, double z, T(&)())
Vector3D(double x_val=0., double y_val=0., double z_val=0.)
Default constructor.
const Vector3D & fill(const double *v)
fill vector from double array
Vector3D & operator=(const Vector3D &v)
Vector3D(const double *v)
Vector3D cross(const Vector3D &v) const
const double * const_array() const
direct access to data as const double*
double operator[](int i) const
static Spherical spherical()
const Vector3D & fill(double x_val, double y_val, double z_val)
fill from double values
double dot(const Vector3D &v) const
bool operator==(const Vector3D &a, const Vector3D &b)
Namespace for the AIDA detector description toolkit.
Vector3D(const Vector3D &v)
bool isEqual(const Vector3D &b, double epsilon=1e-6)
std::ostream & operator<<(std::ostream &os, const BitField64 &b)
Vector3D(double x_val, double y_val, double z_val)