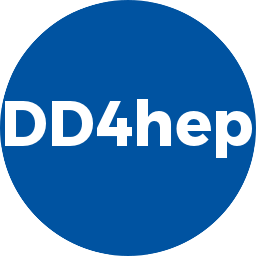 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
28 : m_detDesc(description), m_det(), m_stopScanning(false)
31 std::string det_name = argv[0];
32 std::string n = det_name[0] ==
'-' ? det_name.substr(1) : det_name;
35 std::stringstream err;
36 err <<
"The subdetector " << det_name <<
" is not known to the geometry.";
37 printout(INFO,
"SurfaceInstaller",err.str().c_str());
38 except(det_name, err.str());
40 printout(INFO,
m_det.
name(),
"+++ Processing SurfaceInstaller for subdetector: '%s'",
m_det.
name());
43 except(
"SurfaceInstaller",
"The plugin takes at least one argument. No argument supplied");
50 printout(FATAL,
"SurfaceInstaller",
"+++ Surfaces for: %s",
det);
51 printout(FATAL,
"SurfaceInstaller",
"+++ %s.",msg.c_str());
52 printout(FATAL,
"SurfaceInstaller",
"+++ You sure you apply the correct plugin to generate");
53 printout(FATAL,
"SurfaceInstaller",
"+++ surfaces for a detector of type %s",typ.c_str());
54 except(
"SurfaceInstaller",
"+++ Failed to install Surfaces to detector "+std::string(
det));
68 TGeoMatrix* mat = component.
placement()->GetMatrix();
69 const double* trans = mat->GetTranslation();
76 std::stringstream log;
84 log <<
"Lookup " <<
" Detector[" << det_elts.size() <<
"]: " << elt_path;
87 log <<
" " <<
" Places[" << all_nodes.size() <<
"]: " << node_path;
90 log <<
" " <<
" detail::matrix[" << all_nodes.size() <<
"]: ";
91 for(PlacementPath::const_reverse_iterator i=all_nodes.rbegin(); i!=all_nodes.rend(); ++i) {
93 log << (
void*)(placed->GetMatrix()) <<
" ";
94 if ( placed->GetUserExtension() ) {
96 for(PlacedVolume::VolIDs::const_iterator j=vid.begin(); j!=vid.end(); ++j) {
97 log << (*j).first <<
":" << (*j).second <<
" ";
101 if ( i+1 == all_nodes.rend() ) log <<
"( -> " << placed->GetName() <<
")";
108 <<
" Sensitive: " << (vol.
isSensitive() ?
"YES" :
"NO ")
109 <<
" Volume: " << (
void*)vol.
ptr() <<
" "
114 std::cout << component.
name() <<
": " << pv.
name() << std::endl;
121 for (_C::const_iterator i=children.begin(); !
m_stopScanning && i!=children.end(); ++i)
const Children & children() const
Access to the list of children.
virtual DetElement detector(const std::string &name) const =0
Retrieve a subdetector element by its name from the detector description.
DetElement parent() const
Access to the detector elements's parent.
std::string type() const
Access detector type (structure, tracker, calorimeter, etc.).
Handle class holding a placed volume (also called physical volume)
PlacedVolume placement() const
Access to the physical volume of this detector element.
Solid solid() const
Access to Solid (Shape)
bool isValid() const
Check the validity of the object held by the handle.
bool isSensitive() const
Accessor if volume is sensitive (ie. is attached to a sensitive detector)
const char * name() const
Access the object name (or "" if not supported by the object)
DetElement m_det
Reference to the detector element of the subdetector.
virtual void install(DetElement e, PlacedVolume pv)
Install volume information. Default implementation only prints!
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
void scan()
Scan through tree of detector elements.
SurfaceInstaller()=delete
No default constructor.
Volume parentVolume(DetElement component) const
Shortcut to access the parent detectorelement's volume.
void invalidInstaller(const std::string &msg) const
Indicate error message and throw exception.
detail::tools::PlacementPath PlacementPath
std::map< std::string, DetElement > Children
const double * placementTranslation(DetElement component) const
Shortcut to access the translation vector of a given component.
detail::tools::ElementPath ElementPath
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
std::string toString(int precision=2) const
Conversion to string for pretty print.
Volume volume() const
Logical volume of this placement.
The main interface to the dd4hep detector description package.
const PlacedVolumeExtension::VolIDs & volIDs() const
Access to the volume IDs.
bool m_stopScanning
Flag to inhibit useless further scans.