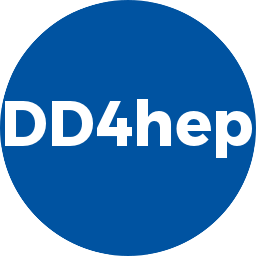 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_SURFACE_H
14 #define DDREC_SURFACE_H
79 double thickness_inner ,
double thickness_outer,
87 _th_i( thickness_inner ),
88 _th_o( thickness_outer ),
164 virtual std::vector< std::pair<Vector3D, Vector3D> >
getLines(
unsigned nMax=100) ;
276 virtual std::vector< std::pair<Vector3D, Vector3D> >
getLines(
unsigned nMax=100) ;
289 struct VolSurfaceList ;
309 std::copy( this->end() , sL->begin() , sL->end() ) ;
316 this->insert( this->end() , vsl.begin() , vsl.end() ) ;
342 VolSurfaceBase( typ, thickness_inner, thickness_outer, u_val,v_val, n_val, o_val, vol, id_val ) {
447 virtual std::vector< std::pair<Vector3D, Vector3D> >
getLines(
unsigned nMax=100) ;
467 VolSurface( new T( typ, thickness_inner, thickness_outer, u_val, v_val, n_val, o_val, vol , 0 ) ){
486 VolSurface( new
VolConeImpl( vol, typ_val, thickness_inner , thickness_outer, v_val, origin_val ) ) {}
504 std::unique_ptr<TGeoMatrix>
_wtM ;
600 virtual std::vector< std::pair< Vector3D, Vector3D> >
getLines(
unsigned nMax=100) ;
643 virtual double radius()
const ;
660 virtual double radius0()
const ;
663 virtual double radius1()
const ;
666 virtual double z0()
const ;
669 virtual double z1()
const ;
711 #endif // DDREC_SURFACE_H
virtual Vector3D v(const Vector3D &point=Vector3D()) const
SurfaceList()=default
defaul c'tor - allow to set ownership for surfaces
virtual double length_along_v() const
Volume volume() const
the volume to which this surface is attached.
virtual Vector3D localToGlobal(const Vector2D &point) const
virtual Vector3D localToGlobal(const Vector2D &point) const
bool checkOrthogonalToZ(const ISurface &surf, double epsilon=1.e-6) const
virtual const SurfaceType & type() const
VolCylinderImpl()
default c'tor
ConeSurface(DetElement det, VolSurface volSurf)
virtual double length_along_v() const
virtual double z1() const
the end z of the cone
virtual std::vector< std::pair< Vector3D, Vector3D > > getLines(unsigned nMax=100)
VolCylinder(Volume vol, SurfaceType typ_val, double thickness_inner, double thickness_outer, Vector3D origin_val)
virtual Vector3D normal(const Vector3D &point=Vector3D()) const
Access to the normal direction at the given point.
std::unique_ptr< TGeoMatrix > _wtM
virtual Vector3D localToGlobal(const Vector2D &point) const
virtual double length_along_v() const
VolSurface volSurface() const
The VolSurface attched to the volume.
virtual Vector3D localToGlobal(const Vector2D &point) const
VolPlaneImpl(SurfaceType typ, double thickness_inner, double thickness_outer, Vector3D u_val, Vector3D v_val, Vector3D n_val, Vector3D o_val, Volume vol, int id_val)
standard c'tor with all necessary arguments - origin is (0,0,0) if not given.
Volume volume() const
the volume to which this surface is attached.
virtual Vector3D u(const Vector3D &point=Vector3D()) const
virtual double distance(const Vector3D &point) const
virtual long64 id() const
The id of this surface.
virtual double outerThickness() const
DetElement detElement() const
The DetElement belonging to the surface volume.
SurfaceList(bool isOwner)
defaul c'tor - allow to set ownership for surfaces
virtual long64 id() const
The id of this surface - corresponds to DetElement id.
bool checkParallelToZ(const ISurface &surf, double epsilon=1.e-6) const
virtual Vector3D u(const Vector3D &point=Vector3D()) const
virtual double innerThickness() const
virtual Vector3D center() const
the center of the cone
virtual Vector3D localToGlobal(const Vector2D &point) const
virtual const IMaterial & outerMaterial() const
Access to the material in direction of the normal.
virtual Vector2D globalToLocal(const Vector3D &point) const
virtual void setNormal(const Vector3D &n)
setter for daughter classes
virtual ~VolSurfaceList()
virtual Vector3D normal(const Vector3D &point=Vector3D()) const
virtual double innerThickness() const
void setOuterMaterial(const IMaterial &mat)
set the outer Materal
virtual const IMaterial & innerMaterial() const
Access to the material in opposite direction of the normal.
virtual Vector2D globalToLocal(const Vector3D &point) const
VolSurfaceBase(const VolSurfaceBase &c)
Copy the from object.
VolSurfaceList(DetElement &det)
virtual Vector3D v(const Vector3D &point=Vector3D()) const
VolSurfaceHandle< VolPlaneImpl > VolPlane
VolSurfaceList * volSurfaceList(DetElement &det)
virtual const IMaterial & outerMaterial() const
Access to the material in direction of the normal.
VolConeImpl()
default c'tor
Handle class describing a detector element.
virtual bool insideBounds(const Vector3D &point, double epsilon=1e-4) const
Checks if the given point lies within the surface.
Handle class holding a placed volume (also called physical volume)
virtual Vector2D globalToLocal(const Vector3D &point) const
virtual long64 id() const
The id of this surface - always 0 for VolSurfaces.
virtual Vector3D localToGlobal(const Vector2D &point) const
virtual Vector2D globalToLocal(const Vector3D &point) const
virtual void setV(const Vector3D &v)
setter for daughter classes
virtual Vector3D normal(const Vector3D &point=Vector3D()) const
virtual const SurfaceType & type() const
virtual double distance(const Vector3D &point) const
virtual double distance(const Vector3D &point) const
VolSurface & operator=(const VolSurface &vsurf)
virtual const Vector3D & origin() const
SurfaceList(const SurfaceList &, const DetElement &)
required c'tor for extension mechanism
virtual double length_along_u() const
virtual double radius() const
the radius of the cylinder (rho of the origin vector)
Surface()=delete
default c'tor etc. removed
virtual double length_along_u() const
virtual double outerThickness() const
virtual Vector3D u(const Vector3D &point=Vector3D()) const
virtual ~SurfaceList()
d'tor deletes all owned surfaces
virtual Vector3D volumeOrigin() const
virtual std::vector< std::pair< Vector3D, Vector3D > > getLines(unsigned nMax=100)
virtual const Vector3D & origin() const
SurfaceList(const SurfaceList &other)=default
copy c'tor
virtual const SurfaceType & type() const
virtual const IMaterial & innerMaterial() const
Access to the material in opposite direction of the normal.
VolSurface(VolSurfaceBase *p)
Constructor to be used with an existing object.
void setOuterMaterial(const IMaterial &mat)
set the outer Materal
VolSurface()
default c'tor
Volume volume() const
The volume that has the surface attached.
VolSurfaceHandle(Volume vol, SurfaceType typ, double thickness_inner, double thickness_outer, Vector3D u_val, Vector3D v_val, Vector3D n_val, Vector3D o_val=Vector3D(0., 0., 0.))
virtual double z0() const
the start z of the cone
virtual bool insideBounds(const Vector3D &point, double epsilon=1e-4) const
Checks if the given point lies within the surface.
virtual double radius0() const
the start radius of the cone
virtual double innerThickness() const
VolSurfaceBase()=default
default c'tor
void setInnerMaterial(const IMaterial &mat)
set the innerMaterial
virtual const IMaterial & innerMaterial() const
Access to the material in opposite direction of the normal.
virtual ~VolSurfaceBase()=default
virtual Vector3D normal(const Vector3D &point=Vector3D()) const
Access to the normal direction at the given point.
Surface(Surface const &)=delete
virtual void setU(const Vector3D &u)
setter for daughter classes
virtual std::vector< std::pair< Vector3D, Vector3D > > getLines(unsigned nMax=100)
void setInnerMaterial(const IMaterial &mat)
set the inner Material
virtual Vector3D u(const Vector3D &point=Vector3D()) const
Namespace for the AIDA detector description toolkit.
virtual double length_along_u() const
virtual Vector2D globalToLocal(const Vector3D &point) const
SurfaceList(const DetElement &)
required c'tor for extension mechanism
virtual Vector3D normal(const Vector3D &point=Vector3D()) const
virtual const Vector3D & origin() const
virtual double radius1() const
the end radius of the cone
virtual Vector3D v(const Vector3D &point=Vector3D()) const
VolCone(Volume vol, SurfaceType typ_val, double thickness_inner, double thickness_outer, Vector3D v_val, Vector3D origin_val)
VolPlaneImpl()
default c'tor
virtual void setOrigin(const Vector3D &o)
setter for daughter classes
void setProperty(unsigned prop, bool val=true)
set the given peorperty
virtual bool insideBounds(const Vector3D &point, double epsilon=1.e-4) const
Checks if the given point lies within the surface.
virtual double distance(const Vector3D &point) const
virtual Vector3D u(const Vector3D &point=Vector3D()) const
virtual Vector3D v(const Vector3D &point=Vector3D()) const
VolSurface(const VolSurface &vsurf)
Constructor to be used with an existing object.
VolSurfaceBase(SurfaceType typ, double thickness_inner, double thickness_outer, Vector3D u_val, Vector3D v_val, Vector3D n, Vector3D o, Volume vol, int identifier)
virtual Vector2D globalToLocal(const Vector3D &point) const
Surface & operator=(Surface const &)=delete
virtual double distance(const Vector3D &point) const
virtual double distance(const Vector3D &point) const
virtual Vector3D center() const
the center of the cylinder
virtual double outerThickness() const
virtual Vector3D v(const Vector3D &point=Vector3D()) const
CylinderSurface(DetElement det, VolSurface volSurf)
Standard c'tor.
VolSurfaceBase * ptr() const
pointer to underlying object
virtual std::vector< std::pair< Vector3D, Vector3D > > getLines(unsigned nMax=100)
create outer bounding lines for the given symmetry of the polyhedron
virtual Vector3D u(const Vector3D &point=Vector3D()) const
VolSurfaceList(const VolSurfaceList &vsl, DetElement &)
virtual Vector3D normal(const Vector3D &point=Vector3D()) const
Access to the normal direction at the given point.
virtual const IMaterial & outerMaterial() const
Access to the material in direction of the normal.