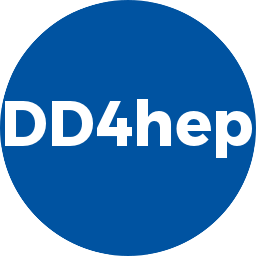 |
DD4hep
1.28.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDREC_ISURFACE_H
14 #define DDREC_ISURFACE_H
24 namespace dd4hep {
namespace rec {
126 virtual double z0()
const=0 ;
127 virtual double z1()
const=0 ;
179 SurfaceType(
unsigned prop0 ,
unsigned prop1 ,
unsigned prop2,
unsigned prop3 ) :
_bits(0) {
187 SurfaceType(
unsigned prop0 ,
unsigned prop1 ,
unsigned prop2,
unsigned prop3,
unsigned prop4 ) :
_bits(0) {
244 unsigned long otherBits = otherType.
_bits.to_ulong() ;
245 unsigned long theseBits =
_bits.to_ulong() ;
247 return ( otherBits & theseBits ) == otherBits ;
299 <<
"] cone[" << t.
isCone()
317 os <<
" id: " << std::hex << s.
id() << std::dec <<
" type : " << s.
type() << std::endl
318 <<
" u : " << s.
u() <<
" v : " << s.
v() <<
" normal : " << s.
normal() <<
" origin : " << s.
origin() << std::endl ;
324 os <<
" cylinder radius : " << cyl->
radius() << std::endl ;
326 const ICone* cone =
dynamic_cast< const ICone*
> ( &s ) ;
328 os <<
" cone radius0: " << cone->
radius0() <<
" cone radius1: " << cone->
radius1() << std::endl ;
338 #endif // DDREC_ISURFACE_H
bool isSimilar(const SurfaceType &otherType) const
true if all properties of otherType are also true for this type.
virtual const IMaterial & outerMaterial() const =0
Access to the material in direction of the normal.
bool checkOrthogonalToZ(const ISurface &surf, double epsilon=1.e-6) const
virtual Vector3D normal(const Vector3D &point=Vector3D()) const =0
Access to the normal direction at the given point.
SurfaceType(unsigned prop0, unsigned prop1)
virtual double z0() const =0
virtual double distance(const Vector3D &point) const =0
bool isZDisk() const
true if this is a plane orthogonal to Z
bool isZCone() const
true if this is a cone parallel to Z
virtual ~ISurface()
Destructor.
virtual double innerThickness() const =0
virtual const Vector3D & origin() const =0
virtual Vector3D v(const Vector3D &point=Vector3D()) const =0
virtual double radius0() const =0
bool isOrthogonalToZ() const
true if surface is orthogonal to Z
virtual double radius() const =0
bool isZPlane() const
true if this is a plane parallel to Z
bool checkParallelToZ(const ISurface &surf, double epsilon=1.e-6) const
virtual ~ICylinder()
Destructor.
virtual bool insideBounds(const Vector3D &point, double epsilon=1.e-4) const =0
Checks if the given point lies within the surface.
virtual const IMaterial & innerMaterial() const =0
Access to the material in opposite direction of the normal.
bool isCone() const
true if this a conical surface
bool isZCylinder() const
true if this is a cylinder parallel to Z
SurfaceTypes
enum for defining the bits used to decode the properties
virtual Vector2D globalToLocal(const Vector3D &point) const =0
bool isHelper() const
true if surface is helper surface for navigation
virtual long64 id() const =0
The id of this surface - corresponds to DetElement id ( or'ed with the placement ids )
virtual double z1() const =0
SurfaceType()
default c'tor
virtual double outerThickness() const =0
virtual ~ICone()
Destructor.
virtual double radius1() const =0
virtual double length_along_u() const =0
bool isVisible() const
true if surface is not invisble - for drawing only
virtual Vector3D center() const =0
SurfaceType(unsigned prop0)
bool isUnbounded() const
true if the surface is unbounded ( ISurface::insideBounds() does not check volume boundaries)
bool isSensitive() const
true if surface is sensitive
virtual Vector3D localToGlobal(const Vector2D &point) const =0
bool isPlane() const
true if this a planar surface
SurfaceType(unsigned prop0, unsigned prop1, unsigned prop2, unsigned prop3)
Namespace for the AIDA detector description toolkit.
std::ostream & operator<<(std::ostream &io, const DCH_info &d)
virtual Vector3D center() const =0
bool isMeasurement1D() const
true if the measurement is only 1D, i.e. the second direction v is not used
SurfaceType(unsigned prop0, unsigned prop1, unsigned prop2, unsigned prop3, unsigned prop4)
void setProperty(unsigned prop, bool val=true)
set the given peorperty
bool isCylinder() const
true if this a cylindrical surface
virtual const SurfaceType & type() const =0
properties of the surface encoded in Type.
virtual Vector3D u(const Vector3D &point=Vector3D()) const =0
virtual double length_along_v() const =0
bool isParallelToZ() const
true if surface is parallel to Z
SurfaceType(unsigned prop0, unsigned prop1, unsigned prop2)