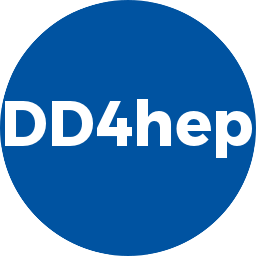 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
67 PRINT(
"%s> calling Geant4TestGeneratorAction(event_id=%d Context: run=%p evt=%p)",
84 PRINT(
"%s> calling begin(run_id=%d,num_event=%d Context:%p)",
m_type.c_str(), run->GetRunID(),
85 run->GetNumberOfEventToBeProcessed(), &
context()->run());
90 PRINT(
"%s> calling end(run_id=%d, num_event=%d Context:%p)",
91 m_type.c_str(), run->GetRunID(), run->GetNumberOfEvent(), &
context()->run());
96 PRINT(
"%s> calling beginEvent(event_id=%d Context: run=%p evt=%p)",
102 PRINT(
"%s> calling endEvent(event_id=%d Context: run=%p evt=%p)",
118 PRINT(
"%s> calling begin(event_id=%d Context: run=%p (%d) evt=%p (%d))",
119 m_type.c_str(), evt->GetEventID(),
126 PRINT(
"%s> calling end(event_id=%d Context: run=%p (%d) evt=%p (%d))",
134 PRINT(
"%s> calling beginRun(run_id=%d,num_event=%d Context:%p)",
135 m_type.c_str(), run->GetRunID(),
136 run->GetNumberOfEventToBeProcessed(),
context()->runPtr());
141 PRINT(
"%s> calling endRun(run_id=%d, num_event=%d Context:%p)",
142 m_type.c_str(), run->GetRunID(),
143 run->GetNumberOfEvent(),
context()->runPtr());
158 PRINT(
"%s> calling begin(track=%d, parent=%d, position=(%f,%f,%f) Context: run=%p evt=%p)",
159 m_type.c_str(), trk->GetTrackID(),
160 trk->GetParentID(), trk->GetPosition().x(), trk->GetPosition().y(), trk->GetPosition().z(),
166 PRINT(
"%s> calling end(track=%d, parent=%d, position=(%f,%f,%f) Context: run=%p evt=%p)",
167 m_type.c_str(), trk->GetTrackID(),
168 trk->GetParentID(), trk->GetPosition().x(), trk->GetPosition().y(), trk->GetPosition().z(),
207 PRINT(
"%s> calling classifyNewTrack(track=%d, parent=%d, position=(%f,%f,%f) Context: run=%p evt=%p)",
208 m_type.c_str(), trk->GetTrackID(),
209 trk->GetParentID(), trk->GetPosition().x(), trk->GetPosition().y(), trk->GetPosition().z(),
230 PRINT(
"%s> calling begin(num_coll=%d, coll=%s Context: run=%p evt=%p)",
231 m_type.c_str(), hce->GetNumberOfCollections(),
232 c ? c->GetName().c_str() :
"None", &
context()->run(), &
context()->event());
238 PRINT(
"%s> calling end(num_coll=%d, coll=%s Context: run=%p evt=%p)",
239 m_type.c_str(), hce->GetNumberOfCollections(),
240 c ? c->GetName().c_str() :
"None", &
context()->run(), &
context()->event());
246 PRINT(
"%s> calling process(track=%d, dE=%f, dT=%f len=%f, First,last in Vol=(%c,%c), coll=%s Context: run=%p evt=%p)",
247 m_type.c_str(), step->GetTrack()->GetTrackID(),
248 step->GetTotalEnergyDeposit(), step->GetDeltaTime(),
249 step->GetStepLength(), step->IsFirstStepInVolume() ?
'Y' :
'N',
250 step->IsLastStepInVolume() ?
'Y' :
'N',
251 c ? c->GetName().c_str() :
"None", &
context()->run(), &
context()->event());
void operator()(const G4Step *, G4SteppingManager *) override
User stepping callback.
virtual void end(const G4Event *) override
End-of-event callback.
Concrete implementation of the Geant4 stepping action sequence.
Geant4TestEventAction(Geant4Context *c, const std::string &n)
Standard constructor with initializing arguments.
Geant4TestStepAction(Geant4Context *c, const std::string &n)
Standard constructor with initializing arguments.
virtual void begin(const G4Track *) override
Begin-of-tracking callback.
void begin(const G4Run *) override
begin-of-run callback
Geant4TestBase(Geant4Action *action, const std::string &typ)
Standard constructor.
virtual ~Geant4TestSensitive()
Default destructor.
Generic hit container class using Geant4HitWrapper objects.
void endRun(const G4Run *)
End-of-run callback.
static void increment(T *)
Increment count according to type information.
virtual void prepare(G4StackManager *) override
Preparation callback.
virtual void newStage(G4StackManager *) override
New-stage callback.
Geant4HitCollection * collection(std::size_t which)
Retrieve the hits collection associated with this detector by its serial number.
virtual TrackClassification classifyNewTrack(G4StackManager *, const G4Track *) override
Return TrackClassification with enum G4ClassificationOfNewTrack or NoTrackClassification.
virtual ~Geant4TestTrackAction()
Default destructor.
virtual ~Geant4TestRunAction()
Default destructor.
Concrete basic implementation of the Geant4 event action.
Handle class describing a detector element.
void beginEvent(const G4Event *)
begin-of-event callback
virtual ~Geant4TestStepAction()
Default destructor.
virtual void begin(const G4Event *) override
begin-of-event callback
void endEvent(const G4Event *)
End-of-event callback.
Geant4TestSensitive(Geant4Context *c, const std::string &n, DetElement det, Detector &description)
Standard constructor with initializing arguments.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
Geant4TestStackAction(Geant4Context *c, const std::string &n)
Standard constructor with initializing arguments.
Concrete implementation of the Geant4 generator action base class.
virtual ~Geant4TestGeneratorAction()
Default destructor.
virtual void operator()(G4Event *) override
Callback to generate primary particles.
Default base class for all Geant 4 actions and derivates thereof.
virtual ~Geant4TestBase()
Default destructor.
Geant4TestRunAction(Geant4Context *c, const std::string &n)
Standard constructor with initializing arguments.
virtual void end(G4HCofThisEvent *) override
End-of-tracking callback.
Geant4TestGeneratorAction(Geant4Context *c, const std::string &n)
Standard constructor with initializing arguments.
virtual ~Geant4TestEventAction()
Default destructor.
Default base class for all geant 4 tracking actions used in DDG4.
virtual void begin(G4HCofThisEvent *) override
Begin-of-tracking callback.
void end(const G4Run *) override
End-of-run callback.
Concrete basic implementation of the Geant4 run action base class.
void beginRun(const G4Run *)
begin-of-run callback
virtual void end(const G4Track *) override
End-of-tracking callback.
virtual bool process(const G4Step *, G4TouchableHistory *) override
Method for generating hit(s) using the information of G4Step object.
The main interface to the dd4hep detector description package.
Concrete implementation of the Geant4 stacking action base class.
Convenience namespace to separate test classes from the DDG4 simulation toolkit.
The base class for Geant4 sensitive detector actions implemented by users.
virtual ~Geant4TestStackAction()
Default destructor.
Common base class for test action.
Geant4HitCollection * collectionByID(std::size_t id)
Retrieve the hits collection associated with this detector by its collection identifier.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4TestTrackAction(Geant4Context *c, const std::string &n)
Standard constructor with initializing arguments.