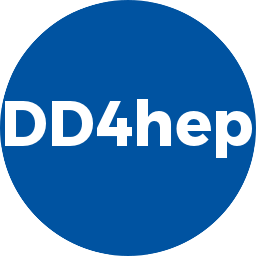 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
28 #include <G4Version.hh>
29 #include <G4TouchableHistory.hh>
30 #include <G4VSensitiveDetector.hh>
31 #include <G4VGFlashSensitiveDetector.hh>
32 #if G4VERSION_NUMBER < 1070
40 const G4FastTrack* track,
41 G4TouchableHistory* hist) = 0;
44 #include <G4VFastSimSensitiveDetector.hh>
86 virtual public G4VGFlashSensitiveDetector,
99 G4VGFlashSensitiveDetector(),
114 this->G4VSensitiveDetector::SetFilter(
this);
121 {
return this->G4VSensitiveDetector::SensitiveDetectorName; }
123 virtual std::string
path() const override final
124 {
return this->G4VSensitiveDetector::GetPathName(); }
126 virtual std::string
fullPath() const override final
127 {
return this->G4VSensitiveDetector::GetFullPathName(); }
130 {
return this->G4VSensitiveDetector::isActive(); }
133 {
return this->G4VSensitiveDetector::GetCollectionID(i); }
136 {
return this->G4VSensitiveDetector::GetROgeometry(); }
144 virtual G4bool
Accept(
const G4Step* step)
const override final
154 G4TouchableHistory* hist)
override final
158 G4TouchableHistory* hist)
override final
160 const GFlashEnergySpot* esp = sp->GetEnergySpot();
161 G4FastHit hit(esp->GetPosition(), esp->GetEnergy());
162 Geant4FastSimSpot spot(&hit, sp->GetOriginatorTrack(), (sp->GetTouchableHandle())());
167 const G4FastTrack* track,
168 G4TouchableHistory* hist)
override final
178 if ( coll.empty() ) {
179 except(
"Geant4Sensitive: No collection defined for %s [Invalid name]",
c_name());
181 collectionName.emplace_back(coll);
182 return collectionName.size()-1;
virtual size_t defineCollection(const std::string &coll) override
Initialize the usage of a hit collection. Returns the collection identifier.
virtual std::string path() const override final
G4VSensitiveDetector internals: Access to the detector path name.
dd4hep::sim::Geant4SensDet Geant4tracker
Lower versions of Geant4 do not provide G4VFastSimSensitiveDetector.
Handle class to hold the information of a sensitive detector.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history)
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
Geant4SensDetActionSequence * sensitiveAction(const std::string &name)
Access to the sensitive detector action from the actioncontainer object.
virtual void clear()
G4VSensitiveDetector interface: Method invoked if the event was aborted.
Geant4Context * m_context
Reference to the Geant4 context.
RefCountedSequence()
Default constructor.
virtual G4VReadOutGeometry * readoutGeometry() const override
Access to the readout geometry of the sensitive detector.
virtual const std::string & sensitiveType() const
Access to the sensitive type of the detector.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
RefCountedSequence(T *seq)
Initializing constructor.
static void increment(T *)
Increment count according to type information.
virtual bool isActive() const override
Is the detector active?
int m_outputLevel
Default property: Output level.
virtual void clear() override
G4VSensitiveDetector interface: Method invoked if the event was aborted.
Geant4Context * workerContext()
Thread's Geant4 execution context.
std::size_t defineCollections(Geant4ActionSD *sens_det)
Called at construction time of the sensitive detector to declare all hit collections.
Spot definition for fast simulation and GFlash.
virtual G4bool ProcessHits(const G4FastHit *hit, const G4FastTrack *track, G4TouchableHistory *hist)=0
Geant4 Fast simulation interface.
Geant4Kernel & worker(unsigned long thread_identifier, bool create_if=false)
Access worker instance by its identifier.
virtual std::string fullPath() const override final
G4VSensitiveDetector internals: Access to the detector path name.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
PrintLevel getOutputLevel(const std::string object) const
Retrieve the global output level of a named object.
virtual ~Geant4SensDet()=default
Destructor.
#define DECLARE_GEANT4SENSITIVEDETECTOR(id)
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
virtual const std::string & sensitiveType() const override final
Access to the sensitive type of the detector.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
virtual void end(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the end of each event.
virtual ~RefCountedSequence()
Default destructor.
static void decrement(T *)
Decrement count according to type information.
virtual void Initialize(G4HCofThisEvent *hce) override final
Method invoked at the begining of each event.
bool accept(const G4Step *step) const
Callback before hit processing starts. Invoke all filters.
Geant4SensDet(const std::string &nam, Detector &description)
Constructor. The detector element is identified by the name.
Default base class for all Geant 4 actions and derivates thereof.
static unsigned long int thread_self()
Access thread identifier.
virtual SensitiveDetector sensitiveDetector() const override final
Access to the Detector sensitive detector handle.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
SensitiveDetector m_sensitive
Access to the geant4 sensitive detector handle.
virtual G4bool ProcessHits(G4GFlashSpot *sp, G4TouchableHistory *hist) override final
GFLASH interface.
Interface class to access properties of the underlying Geant4 sensitive detector structure.
dd4hep::sim::Geant4SensDet Geant4SensDet
static Geant4Kernel & instance(Detector &description)
Instance accessor.
const char * c_name() const
Access name of the action.
The sequencer to host Geant4 sensitive actions called if particles interact with sensitive elements.
virtual void EndOfEvent(G4HCofThisEvent *hce) override final
Method invoked at the end of each event.
dd4hep::sim::Geant4SensDet Geant4calorimeter
Namespace for the AIDA detector description toolkit.
virtual G4bool Accept(const G4Step *step) const override final
Callback if the sequence should be accepted or filtered off.
G4String GetName() const
Overload to avoid ambiguity between G4VSensitiveDetector and G4VSDFilter.
The main interface to the dd4hep detector description package.
Private helper to support sequence reference counting.
virtual void begin(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the begining of each event.
Concrete implementation of the G4VSensitiveDetector calling the registered sequence object.
virtual G4bool ProcessHits(G4Step *step, G4TouchableHistory *hist) override final
Method for generating hit(s) using the information of G4Step object.
G4VFastSimSensitiveDetector()=default
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
virtual ~G4VFastSimSensitiveDetector()=default
virtual G4int GetCollectionID(G4int i) override final
This is a utility method which returns the hits collection ID.
virtual bool process(const G4Step *step, G4TouchableHistory *history)
G4VSensitiveDetector interface: Method for generating hit(s) using the information of G4Step object.
virtual G4bool ProcessHits(const G4FastHit *hit, const G4FastTrack *track, G4TouchableHistory *hist) override final
Geant4 Fast simulation interface.