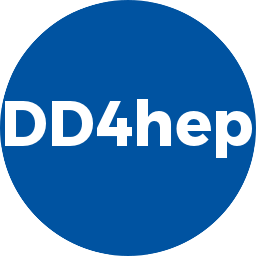 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
28 const std::string& nam,
30 const std::string& coll)
31 :
Geant4Filter(ctxt, nam), m_readout(ro), m_collection(0), m_key(0)
34 for(
size_t i=0; i<ro->hits.size(); ++i) {
36 if ( c.
name == coll ) {
42 except(
"+++ Custom collection name '%s' not defined in the Readout object: %s.",
43 coll.c_str(), ro.
name());
std::string name
Hit collection name.
long key_min
Range values of the key is not empty.
Definition of the HitCollection parameters used by the Readout.
const BitFieldElement * field(const std::string &field_name) const
Get the field descriptor of one field by name.
const HitCollection * m_collection
Collection index.
std::string key
Discriminator key name from the <id> string.
FieldID value(CellID bitfield) const
calculate this field's value given an external 64 bit bitmap
Helper class to ease the extraction of information from a G4FastSimSpot object.
virtual ~Geant4ReadoutVolumeFilter()
Default destructor.
static void increment(T *)
Increment count according to type information.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
const char * name() const
Access the object name (or "" if not supported by the object)
Spot definition for fast simulation and GFlash.
Helper class to ease the extraction of information from a G4Step object.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
VolumeID volumeID(const G4VTouchable *touchable) const
Access CELLID by placement path.
const G4VTouchable * touchable() const
static void decrement(T *)
Decrement count according to type information.
The Geant4VolumeManager to facilitate optimized lookups of cell IDs from touchables.
Geant4VolumeManager volumeManager() const
Access the volume manager.
virtual bool operator()(const G4Step *step) const override
Filter action. Return true if hits should be processed.
Geant4ReadoutVolumeFilter(Geant4Context *context, const std::string &name, Readout ro, const std::string &coll)
Standard constructor.
dd4hep::DDSegmentation::VolumeID VolumeID
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Base class to construct filters for Geant4 sensitive detectors.
Handle to the implementation of the readout structure of a subdetector.
IDDescriptor idSpec() const
Access IDDescription structure.
const BitFieldElement * m_key
Bit field value from ID descriptor.
const G4VTouchable * preTouchable() const
Generic context to extend user, run and event information.