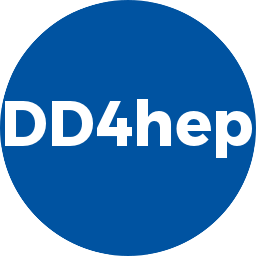 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETAIL_OBJECTSINTERNA_H
14 #define DD4HEP_DETAIL_OBJECTSINTERNA_H
71 ConstantObject(
const std::string& nam,
const std::string& val,
const std::string& typ);
170 HitCollection(
const std::string&
name,
const std::string&
key=
"",
long k_min=~0x0,
long kmax=~0x0);
191 std::vector<HitCollection>
hits;
208 typedef std::vector<std::pair<std::string, const BitFieldElement*> >
FieldMap;
209 typedef std::vector<std::pair<size_t, std::string> >
FieldIDs;
227 const std::vector<BitFieldElement*>& fields()
const {
235 #endif // DD4HEP_DETAIL_OBJECTSINTERNA_H
std::vector< std::string > user_limits
References to user limits.
std::string name
Hit collection name.
long key_min
Range values of the key is not empty.
Concrete object implementation of the IDDescriptorObject Handle.
BitFieldCoder decoder
Decoder object.
Segmentation segmentation
Handle to the readout segmentation.
FieldIDs fieldIDs
String map of id descriptors.
Definition of the HitCollection parameters used by the Readout.
Concrete object implementation of the Readout Handle.
virtual ~ReadoutObject()
Default destructor.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
std::string key
Discriminator key name from the <id> string.
IDDescriptorObject()
Default constructor.
Class implementing the ID encoding of the detector response.
std::vector< std::pair< size_t, std::string > > FieldIDs
const std::vector< BitFieldElement > & fields() const
Volume readoutWorld
Handle to the volume.
virtual ~IDDescriptorObject()
Default destructor.
ConstantObject(const ConstantObject &)
Private copy constructor.
IDDescriptor id
Handle to the field descriptor.
std::set< Limit >::iterator iterator
Iterator definitions.
HitCollection()
Default constructor.
virtual ~LimitSetObject()
Default destructor.
std::string description
The description string to build the bit-field descriptors.
Handle class holding a placed volume (also called physical volume)
LimitSetObject()
Standard constructor.
unsigned char showDaughters
Concrete object implementation of the Region Handle.
virtual ~VisAttrObject()
Default destructor.
unsigned char drawingStyle
ReadoutObject()
Standard constructor.
std::vector< std::pair< std::string, const BitFieldElement * > > FieldMap
std::string dataType
Constant type.
Concrete object implementation for the Constant handle.
ConstantObject & operator=(const ConstantObject &)
Private assignment operator.
VisAttrObject()
Standard constructor.
virtual ~RegionObject()
Default destructor.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
HitCollection & operator=(const HitCollection &c)
Assignment operator.
Handle class supporting generic Segmentations of sensitive detectors.
FieldMap fieldMap
Map of id-fields in the descriptor.
Concrete object implementation of the LimitSet Handle.
std::set< Limit > limits
Particle specific limits.
std::set< Limit > cuts
Particle specific production cuts.
std::vector< HitCollection > hits
Hit collection container (if defined)
virtual ~ConstantObject()
Default destructor.
ConstantObject()
Default constructor for ROOT persistency.
std::set< Limit >::const_iterator const_iterator
Concrete object implementation of the VisAttr Handle.
RegionObject()
Standard constructor.