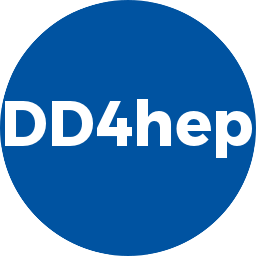 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #include <G4HCofThisEvent.hh>
25 #include <G4ParticleTable.hh>
57 TDirectory::TContext ctxt(
m_file);
59 info(
"+++ Closing ROOT output file %s",
m_file->GetName());
66 detail::deletePtr (
m_file);
72 Sections::const_iterator i =
m_sections.find(nam);
74 TDirectory::TContext ctxt(
m_file);
75 TTree* t =
new TTree(nam.c_str(), (
"Geant4 " + nam +
" information").c_str());
91 fname +=
_toString(run->GetRunID(),
".run%08d");
92 if ( idx != std::string::npos )
95 if ( !
m_file && !fname.empty() ) {
96 TDirectory::TContext ctxt(TDirectory::CurrentDirectory());
97 if ( !gSystem->AccessPathName(fname.c_str()) ) {
98 gSystem->Unlink(fname.c_str());
100 std::unique_ptr<TFile> file(TFile::Open(fname.c_str(),
"RECREATE",
"dd4hep Simulation data"));
102 file.reset(TFile::Open((fname+
".1").c_str(),
"RECREATE",
"dd4hep Simulation data"));
105 except(
"Failed to create ROOT output file:'%s'", fname.c_str());
107 if (file->IsZombie()) {
108 detail::deletePtr (
m_file);
109 except(
"Failed to open ROOT output file:'%s'", fname.c_str());
121 Branches::const_iterator i =
m_branches.find(nam);
123 const std::type_info& typ = type.type();
124 TClass* cl = TBuffer::GetClass(typ);
126 b =
m_tree->Branch(nam.c_str(), cl->GetName(), (
void*) 0);
127 b->SetAutoDelete(
false);
131 throw std::runtime_error(
"No ROOT TClass object availible for object type:" + typeName(typ));
137 Long64_t evt = b->GetEntries(), nevt = b->GetTree()->GetEntries(), num = nevt - evt;
146 int nbytes = b->Fill();
148 throw std::runtime_error(
"Failed to write ROOT collection:" + nam +
"!");
158 TObjArray* a =
m_tree->GetListOfBranches();
159 Long64_t evt =
m_tree->GetEntries() + 1;
160 Int_t nb = a->GetEntriesFast();
162 for (Int_t i = 0; i < nb; ++i) {
163 TBranch* br_ptr = (TBranch*) a->UncheckedAt(i);
164 Long64_t br_evt = br_ptr->GetEntries();
166 Long64_t num = evt - br_evt;
167 br_ptr->SetAddress(0);
186 Manip* manipulator = Geant4HitWrapper::manipulator<Geant4Particle>();
187 G4ParticleTable* table = G4ParticleTable::GetParticleTable();
188 const ParticleMap& pm = parts->
particles();
189 std::vector<void*> particles;
190 particles.reserve(pm.size());
191 for (
const auto& i : pm ) {
193 G4ParticleDefinition* def = table->FindParticle(p->pdgID);
194 p->charge = int(3.0 * (def ? def->GetPDGCharge() : -1.0));
195 particles.emplace_back((ParticleMap::mapped_type*)p);
197 fill(
"MCParticles",manipulator->vec_type,&particles);
205 std::string hc_nam = collection->GetName();
212 std::vector<void*> hits;
214 size_t nhits = coll->
GetSize();
218 for(
size_t i=0; i<nhits; ++i) {
221 if ( 0 != trk_hit ) {
227 if ( 0 != cal_hit ) {
229 for(Geant4HitData::Contributions::iterator j=c.begin(); j!=c.end(); ++j) {
238 error(
"+++ Exception while saving collection %s.",hc_nam.c_str());
virtual size_t GetSize() const override
Access the collection size.
virtual void saveEvent(OutputContext< G4Event > &ctxt) override
Callback to store the Geant4 event.
const ParticleMap & particles() const
Access the particle map.
Generic hit container class using Geant4HitWrapper objects.
Geant4HitWrapper & hit(size_t which)
Access the hit wrapper.
std::string _toString(bool value)
String conversions: boolean value to string.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Base class to output Geant4 event data to persistent media.
static void increment(T *)
Increment count according to type information.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
void getHitsUnchecked(std::vector< void * > &result)
Release all hits from the Geant4 container. Ownership stays with the container.
bool m_handleMCTruth
Property: Flag if Monte-Carlo truth should be followed and checked.
virtual ~Geant4Output2ROOT()
Default destructor.
virtual void commit(OutputContext< G4Event > &ctxt) override
Commit data at end of filling procedure.
Helper class for thread savety.
Data structure to map particles produced during the generation and the simulation.
void info(const char *fmt,...) const
Support of info messages.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
Geant4Output2ROOT(Geant4Context *context, const std::string &nam)
Standard constructor.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
std::vector< MonteCarloContrib > Contributions
TTree * m_tree
Reference to the event data tree.
int fill(const std::string &nam, const ComponentCast &type, void *ptr)
Fill single EVENT branch entry (Geant4 collection data)
virtual void commit(OutputContext< G4Event > &ctxt)
Commit data at end of filling procedure.
void error(const char *fmt,...) const
Support of error messages.
const ComponentCast & vector_type() const
Type information of the vector type for extracting data.
DDG4 tracker hit class used by the generic DDG4 tracker sensitive detector.
std::map< int, Particle * > ParticleMap
Base class for geant4 hit structures used by the default DDG4 sensitive detector implementations.
Geant4ParticleMap * m_truth
Reference to MC truth object.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
virtual void beginRun(const G4Run *run)
Callback to initialize storing the Geant4 information.
Branches m_branches
Branches in the event tree.
Contribution truth
Monte Carlo / Geant4 information.
DDG4 calorimeter hit class used by the generic DDG4 calorimeter sensitive detector.
TTree * section(const std::string &nam)
Create/access tree by name for non collection user data.
int particleID(int track, bool throw_if_not_found=true) const
Access the equivalent track id (shortcut to the usage of TrackEquivalents)
int trackID
Geant 4 Track identifier.
virtual void saveCollection(OutputContext< G4Event > &ctxt, G4VHitsCollection *collection) override
Callback to store each Geant4 hit collection.
Sections m_sections
Known file sections.
std::vector< std::string > m_disabledCollections
Property: vector with disabled collections.
std::string m_output
Property: "Output" output destination.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual void beginRun(const G4Run *run) override
Callback to store the Geant4 run information.
std::string m_section
Property: name of the event tree.
bool m_disableParticles
Property: vector with disabled collections.
Generic type manipulation class for generic hit structures created in Geant4 sensitive detectors.
Contributions truth
Hit contributions by individual particles.
bool m_filesByRun
Property: Flag if Monte-Carlo truth should be followed and checked.
Utility class describing the monte carlo contribution of a given particle to a hit.
virtual void closeOutput()
Close current output file.
TFile * m_file
Reference to the ROOT file to open.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.