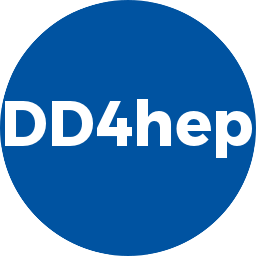 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4PARTICLE_H
15 #define DDG4_GEANT4PARTICLE_H
20 #include <Math/Vector4D.h>
23 class G4ParticleDefinition;
173 #ifndef __DDG4_STANDALONE_DICTIONARIES__
227 template <
typename T> std::vector<T>
pxPyPzM(T unit)
const;
233 const G4ParticleDefinition *
definition()
const;
238 void dump1(
int level,
const std::string& src,
const char* tag)
const;
240 void dump2(
int level,
const std::string& src,
const char* tag,
int g4id,
bool inrec)
const;
242 void dumpWithVertex(
int level,
const std::string& src,
const char* tag)
const;
243 void dumpWithMomentum(
int level,
const std::string& src,
const char* tag)
const;
245 static void header4(
int level,
const std::string& src,
const char* tag);
246 void dump4(
int level,
const std::string& src,
const char* tag)
const;
251 void offset(
int off)
const;
254 static std::vector<G4ParticleDefinition*>
g4DefinitionsRegEx(
const std::string& expression);
260 : particle(c.particle) {
292 return ROOT::Math::PxPyPzM4D<double>(p->
psx,p->
psy,p->
psz,p->
mass);
298 return ROOT::Math::Cartesian3D<double>(p->
vsx,p->
vsy,p->
vsz);
304 return ROOT::Math::Cartesian3D<double>(p->
vex,p->
vey,p->
vez);
365 int particleID(
int track,
bool throw_if_not_found=
true)
const;
371 #endif // DDG4_GEANT4PARTICLE_H
@ G4PARTICLE_CREATED_TRACKER_HIT
void offset(int off) const
Handlers.
Geant4ParticleMap()
Default constructor.
ParticleExtension()
Default constructor.
double momentum2() const
Scalar particle momentum squared.
@ G4PARTICLE_ABOVE_ENERGY_THRESHOLD
void dump1(int level, const std::string &src, const char *tag) const
Various output formats:
@ G4PARTICLE_SIM_PARENT_RADIATED
void dump() const
Dump content.
double mass
Particle mass.
std::string particleName() const
Access to the Geant4 particle name.
void dumpWithVertex(int level, const std::string &src, const char *tag) const
Output type 3:+++ "tag" ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
const ParticleMap & particles() const
Access the particle map.
@ G4PARTICLE_KEEP_PROCESS
size_t numDaughter() const
Accessor to the number of particle daughters.
Geant4Particle * addRef()
Increase reference count.
static std::vector< G4ParticleDefinition * > g4DefinitionsRegEx(const std::string &expression)
Access Geant4 particle definitions by regular expression.
ROOT::Math::PxPyPzM4D< double > FourVector
void adopt(ParticleMap &pm, TrackEquivalents &equiv)
Adopt particle maps.
Geant4ParticleHandle(Geant4Particle *part)
Default constructor.
std::string processName() const
Access to the creator process name.
Geant4Particle(const Geant4Particle ©)=delete
NO copy constructor.
ThreeVector endVertex() const
Access particle momentum, energy as 4 vector.
int g4Parent
not persistent
std::map< int, int > TrackEquivalents
Geant4Particle * operator->() const
Overloaded -> operator to access particle details.
virtual ~Geant4Particle()
Default destructor.
@ G4PARTICLE_GEN_STATUS_MASK
virtual ~Geant4ParticleMap()
Default destructor.
int charge3() const
Charge accessor (for python etc.)
@ G4PARTICLE_GEN_DOCUMENTATION
@ G4PARTICLE_SIM_BACKSCATTER
void dumpWithMomentumAndVertex(int level, const std::string &src, const char *tag) const
Output type 3:+++ <tag> ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
std::unique_ptr< ParticleExtension > extension
User data extension if required.
double charge() const
Geant4 charge of the particle.
Geant4Particle & get_data(Geant4Particle &c)
Assignment operator.
Data structure to map particles produced during the generation and the simulation.
virtual ~ParticleExtension()
Default destructor.
std::string particleType() const
Access to the Geant4 particle type.
std::vector< T > pxPyPzM(T unit) const
Access particle momentum, energy as 4 vector.
void dump2(int level, const std::string &src, const char *tag, int g4id, bool inrec) const
Output type 2:+++ "tag" 20 G4: 7 def:0xde4eaa8 [gamma , gamma] reason: 20 E:+3.304035e+01 in record:Y...
std::set< int > Particles
@ G4PARTICLE_SIM_DECAY_CALO
size_t numParent() const
Accessor to the number of particle parents.
std::map< int, Particle * > ParticleMap
const TrackEquivalents & equivalents() const
Access the map of track equivalents.
@ G4PARTICLE_GEN_GENERATOR
ROOT::Math::Cartesian3D< double > ThreeVector
FourVector pxPyPzM() const
Access particle momentum, energy as 4 vector.
void dump4(int level, const std::string &src, const char *tag) const
@ G4PARTICLE_LAST_NOTHING
Geant4ParticleHandle & operator=(Geant4Particle *part)
Assignment operator.
Particles daughters
The list of daughters of this MC particle.
@ G4PARTICLE_SIM_DECAY_TRACKER
Geant4ParticleProperties
Track properties.
double momentum() const
Scalar particle momentum.
std::string processTypeName() const
Access to the creator process type name.
double time
Particle creation time.
const G4VProcess * process
Reference to the G4VProcess, which created this track.
static G4ParticleDefinition * g4DefinitionsExact(const std::string &expression)
Access Geant4 particle definitions by exact match.
double pex
The track momentum at the end vertex.
int particleID(int track, bool throw_if_not_found=true) const
Access the equivalent track id (shortcut to the usage of TrackEquivalents)
@ G4PARTICLE_HAS_SECONDARIES
double vex
The end vertex.
double vsx
The starting vertex.
void clear()
Clear particle maps.
double psx
The track momentum at the start vertex.
const G4ParticleDefinition * definition() const
Access the Geant4 particle definition object (expensive!)
ParticleMap particleMap
Mapping of particles of this event.
@ G4PARTICLE_SIM_LEFT_DETECTOR
Geant4Particle()
not persistent
double mass() const
Geant4 mass of the particle.
Geant4Particle * particle
Particle pointer.
Namespace for the AIDA detector description toolkit.
Data structure to access derived MC particle information.
void dumpWithMomentum(int level, const std::string &src, const char *tag) const
Output type 3:+++ <tag> ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
Particles parents
The list of parents of this MC particle.
ThreeVector startVertex() const
Access particle momentum, energy as 4 vector.
TrackEquivalents equivalentTracks
Map associating the G4Track identifiers with identifiers of existing MCParticles.
@ G4PARTICLE_CREATED_CALORIMETER_HIT
Base class to extend the basic particle class used by DDG4 with user information.
Data structure to store the MC particle information.
double time() const
Geant4 time of the particle.
double energy() const
Scalar particle energy.
bool isValid() const
Check if the particle map was ever filled (ie. some particle handler was present)
Geant4Particle & operator=(const Geant4Particle ©)=delete
NO assignment operation.
void release()
Decrease reference count. Deletes object if NULL.
double properTime
Proper time.
static void header4(int level, const std::string &src, const char *tag)
void removeDaughter(int id_daughter)
Remove daughter from set.
int ref
Reference counter.