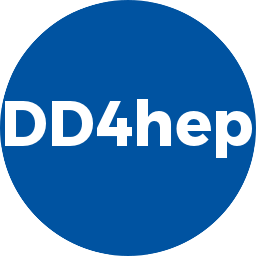 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
20 #include <G4ChargedGeantino.hh>
21 #include <G4Geantino.hh>
22 #include <G4IonTable.hh>
23 #include <G4ParticleDefinition.hh>
24 #include <G4ParticleTable.hh>
25 #include <G4VProcess.hh>
27 #include <TDatabasePDG.h>
28 #include <TParticlePDG.h>
99 #if __cplusplus >= 201103L
117 G4ParticleTable* tab = G4ParticleTable::GetParticleTable();
118 G4ParticleDefinition* def = tab->FindParticle(
particle->
pdgID);
123 const int L =
id / 10000000;
id %= 10000000;
124 const int Z =
id / 10000;
id %= 10000;
125 const int A =
id / 10;
id %= 10;
127 G4IonTable* tab_ion = G4IonTable::GetIonTable();
129 G4ParticleDefinition* def_ion = tab_ion->FindIon(Z, A, L, lvl);
132 printout(VERBOSE,
"Geant4Particle",
"+++ Returning ion with PDG %10d", def_ion->GetPDGEncoding());
134 }
else if(lvl == 0) {
136 printout(VERBOSE,
"Geant4Particle",
"+++ Creating ion with PDG %10d",
particle->
pdgID);
137 return tab_ion->GetIon(Z, A, L, 0.0);
140 printout(WARNING,
"Geant4Particle",
"+++ Cannot find excited ion with PDG %10d, setting excitation level to zero",
142 return tab_ion->GetIon(Z, A, L, 0.0);
147 return G4Geantino::Definition();
148 return G4ChargedGeantino::Definition();
155 if (
const G4ParticleDefinition* def =
definition() )
156 return def->GetParticleName();
158 TDatabasePDG* db = TDatabasePDG::Instance();
160 if ( pdef )
return pdef->GetName();
169 if (
const G4ParticleDefinition* def =
definition() )
170 return def->GetParticleType();
172 TDatabasePDG* db = TDatabasePDG::Instance();
174 if ( pdef )
return pdef->ParticleClass();
183 std::vector<G4ParticleDefinition*> results;
184 std::string exp = expression;
185 G4ParticleTable* pt = G4ParticleTable::GetParticleTable();
186 G4ParticleTable::G4PTblDicIterator* iter = pt->GetIterator();
189 if ( expression ==
"*" || expression ==
".(*)" ) {
191 G4ParticleDefinition* p = iter->value();
192 results.emplace_back(p);
197 int ret = ::regcomp(®, exp.c_str(), 0);
199 throw std::runtime_error(format(
"Geant4ParticleHandle",
"REGEX: Failed to compile particle name %s", exp.c_str()));
202 G4ParticleDefinition* p = iter->value();
203 ret = ::regexec(®, p->GetParticleName().c_str(), 0, NULL, 0);
205 results.emplace_back(p);
206 else if (ret == REG_NOMATCH)
210 ::regerror(ret, ®, msgbuf,
sizeof(msgbuf));
212 throw std::runtime_error(format(
"Geant4ParticleHandle",
"REGEX: Failed to match particle name %s err=%s", exp.c_str(), msgbuf));
222 G4ParticleTable* tab = G4ParticleTable::GetParticleTable();
223 G4ParticleDefinition* def = tab->FindParticle(expression);
243 return G4VProcess::GetProcessTypeName(
particle->
process->GetProcessType());
256 for(
auto i : temp) p->
daughters.insert(i+off);
260 for(
auto i : temp ) p->
parents.insert(i+off);
269 ::snprintf(text,
sizeof(text),
"/%d",*(p->
parents.begin()));
270 else if ( p->
parents.size() > 1 ) {
271 text[0]=
'/';text[1]=0;
273 ::snprintf(text+strlen(text),
sizeof(text)-strlen(text),
"%d ",i);
275 printout((dd4hep::PrintLevel)level,src,
276 "+++ %s %4d def [%-11s,%8s] reason:%8d E:%+.2e %3s #Dau:%3d #Par:%3d%-5s",
291 if ( p->
parents.size() == 0 ) text[0]=0;
292 else if ( p->
parents.size() == 1 ) ::snprintf(text,
sizeof(text),
"/%d",*(p->
parents.begin()));
293 else if ( p->
parents.size() > 1 ) ::snprintf(text,
sizeof(text),
"/%d..",*(p->
parents.begin()));
294 printout((dd4hep::PrintLevel)level,src,
295 "+++ %s %4d G4:%4d [%-12s,%8s] reason:%8d "
296 "E:%+.2e in record:%s #Par:%3d%-5s #Dau:%3d",
313 ::snprintf(text,
sizeof(text),
"/%d",*(p->
parents.begin()));
314 else if ( p->
parents.size() > 1 ) {
315 text[0]=
'/';text[1]=0;
317 ::snprintf(text+strlen(text),
sizeof(text)-strlen(text),
"%d ",i);
319 printout((dd4hep::PrintLevel)level,src,
320 "+++ %s ID:%3d %-12s status:%08X PDG:%6d Vtx:(%+.2e,%+.2e,%+.2e)[mm] "
321 "time: %+.2e [ns] #Dau:%3d #Par:%1d%-6s",
323 p->
vsx/CLHEP::mm,p->
vsy/CLHEP::mm,p->
vsz/CLHEP::mm,p->
time/CLHEP::ns,
336 ::snprintf(text,
sizeof(text),
"/%d",*(p->
parents.begin()));
337 else if ( p->
parents.size() > 1 ) {
338 text[0]=
'/';text[1]=0;
340 ::snprintf(text+strlen(text),
sizeof(text)-strlen(text),
"%d ",i);
342 printout((dd4hep::PrintLevel)level,src,
343 "+++%s ID:%3d %-12s stat:%08X PDG:%6d Mom:(%+.2e,%+.2e,%+.2e)[MeV] "
344 "time: %+.2e [ns] #Dau:%3d #Par:%1d%-6s",
346 p->
psx/CLHEP::MeV,p->
psy/CLHEP::MeV,p->
psz/CLHEP::MeV,p->
time/CLHEP::ns,
358 ::snprintf(text,
sizeof(text),
"/%d",*(p->
parents.begin()));
359 else if ( p->
parents.size() > 1 ) {
360 text[0]=
'/';text[1]=0;
362 ::snprintf(text+strlen(text),
sizeof(text)-strlen(text),
"%d ",i);
364 printout((dd4hep::PrintLevel)level,src,
365 "+++%s %3d %-12s stat:%08X PDG:%6d Mom:(%+.2e,%+.2e,%+.2e)[MeV] "
366 "Vtx:(%+.2e,%+.2e,%+.2e)[mm] #Dau:%3d #Par:%1d%-6s",
368 p->
psx/CLHEP::MeV,p->
psy/CLHEP::MeV,p->
psz/CLHEP::MeV,
369 p->
vsx/CLHEP::mm,p->
vsy/CLHEP::mm,p->
vsz/CLHEP::mm,
376 printout((dd4hep::PrintLevel)level,src,
377 "+++ %s %10s/%-7s %12s/%-10s %6s/%-6s %4s %4s "
378 "%-4s %-3s %-3s %-10s "
379 "%-5s %-6s %-3s %-3s %-20s %s",
380 tag,
"ID",
"G4-ID",
"Part-Name",
"PDG",
"Parent",
"G4-ID",
"#Par",
"#Dau",
381 "Prim",
"Sec",
">E",
"Energy",
382 "EMPTY",
"STAB",
"DEC",
"DOC",
383 "Process",
"Processing Flags");
387 using PropertyMask = dd4hep::detail::ReferenceBitMask<int>;
394 std::string proc =
'['+proc_name+(p->
process ?
"/" :
"")+proc_type+
']';
401 std::stringstream str;
403 for(
const auto i : p->
parents )
405 str <<
" Daughters: ";
408 printout((dd4hep::PrintLevel)level,src,
409 "+++ %s ID:%7d/%-7d %12s/%-10d %6d/%-6d %4d %4d %-4s %-3s %-3s %+.3e "
410 "%-5s %-4s %-3s %-3s %-20s %c%c%c%c -- %c%c%c%c%c%c%c%c%c %s",
445 printout((dd4hep::PrintLevel)level,src,
446 "+++ %s ID:%7d %12s %6d%-7s %7s %3s %5d %3s %+.3e %-4s %-7s %-3s %-3s %2d [%s%s%s] %c%c%c%c -- %c%c%c%c%c%c%c",
501 std::cout <<
"Particle map:" << std::endl;
503 std::snprintf(text,
sizeof(text),
" [%-4d:%p]",p.second->id,(
void*)p.second);
506 std::cout << std::endl;
510 std::cout << std::endl;
513 std::cout <<
"Equivalents:" << std::endl;
515 std::snprintf(text,
sizeof(text),
" [%-5d : %-5d]",p.first,p.second);
518 std::cout << std::endl;
522 std::cout << std::endl;
544 printout(ERROR,
"Geant4ParticleMap",
"+++ No Equivalent particle for track:%d."
545 " Monte Carlo truth record looks broken!",g4_id);
@ G4PARTICLE_CREATED_TRACKER_HIT
void offset(int off) const
Handlers.
@ G4PARTICLE_ABOVE_ENERGY_THRESHOLD
void dump1(int level, const std::string &src, const char *tag) const
Various output formats:
@ G4PARTICLE_SIM_PARENT_RADIATED
void dump() const
Dump content.
double mass
Particle mass.
std::string particleName() const
Access to the Geant4 particle name.
void dumpWithVertex(int level, const std::string &src, const char *tag) const
Output type 3:+++ "tag" ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
@ G4PARTICLE_KEEP_PROCESS
static std::vector< G4ParticleDefinition * > g4DefinitionsRegEx(const std::string &expression)
Access Geant4 particle definitions by regular expression.
void adopt(ParticleMap &pm, TrackEquivalents &equiv)
Adopt particle maps.
std::string processName() const
Access to the creator process name.
int g4Parent
not persistent
static void increment(T *)
Increment count according to type information.
std::map< int, int > TrackEquivalents
virtual ~Geant4Particle()
Default destructor.
virtual ~Geant4ParticleMap()
Default destructor.
@ G4PARTICLE_GEN_DOCUMENTATION
@ G4PARTICLE_SIM_BACKSCATTER
void dumpWithMomentumAndVertex(int level, const std::string &src, const char *tag) const
Output type 3:+++ <tag> ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
std::unique_ptr< ParticleExtension > extension
User data extension if required.
Geant4Particle & get_data(Geant4Particle &c)
Assignment operator.
Data structure to map particles produced during the generation and the simulation.
virtual ~ParticleExtension()
Default destructor.
std::string particleType() const
Access to the Geant4 particle type.
void dump2(int level, const std::string &src, const char *tag, int g4id, bool inrec) const
Output type 2:+++ "tag" 20 G4: 7 def:0xde4eaa8 [gamma , gamma] reason: 20 E:+3.304035e+01 in record:Y...
@ G4PARTICLE_SIM_DECAY_CALO
size_t numParent() const
Accessor to the number of particle parents.
std::map< int, Particle * > ParticleMap
static void decrement(T *)
Decrement count according to type information.
void dump4(int level, const std::string &src, const char *tag) const
Particles daughters
The list of daughters of this MC particle.
@ G4PARTICLE_SIM_DECAY_TRACKER
std::string processTypeName() const
Access to the creator process type name.
double time
Particle creation time.
const G4VProcess * process
Reference to the G4VProcess, which created this track.
static G4ParticleDefinition * g4DefinitionsExact(const std::string &expression)
Access Geant4 particle definitions by exact match.
double pex
The track momentum at the end vertex.
int particleID(int track, bool throw_if_not_found=true) const
Access the equivalent track id (shortcut to the usage of TrackEquivalents)
@ G4PARTICLE_HAS_SECONDARIES
double vex
The end vertex.
double vsx
The starting vertex.
void clear()
Clear particle maps.
double psx
The track momentum at the start vertex.
const G4ParticleDefinition * definition() const
Access the Geant4 particle definition object (expensive!)
ParticleMap particleMap
Mapping of particles of this event.
@ G4PARTICLE_SIM_LEFT_DETECTOR
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Particle()
not persistent
Geant4Particle * particle
Particle pointer.
Data structure to access derived MC particle information.
void dumpWithMomentum(int level, const std::string &src, const char *tag) const
Output type 3:+++ <tag> ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
Particles parents
The list of parents of this MC particle.
TrackEquivalents equivalentTracks
Map associating the G4Track identifiers with identifiers of existing MCParticles.
@ G4PARTICLE_CREATED_CALORIMETER_HIT
Data structure to store the MC particle information.
dd4hep::detail::ReferenceBitMask< int > PropertyMask
double energy() const
Scalar particle energy.
bool isValid() const
Check if the particle map was ever filled (ie. some particle handler was present)
void release()
Decrease reference count. Deletes object if NULL.
double properTime
Proper time.
static void header4(int level, const std::string &src, const char *tag)
void removeDaughter(int id_daughter)
Remove daughter from set.
int ref
Reference counter.