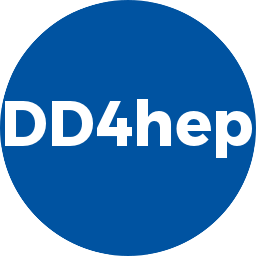 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #include <G4Allocator.hh>
29 : cast(c), vec_type(
v) {
47 void* Geant4HitWrapper::operator
new(size_t) {
55 void Geant4HitWrapper::operator
delete(
void *p) {
63 m_data.second = manipulator<InvalidHit>();
71 m_data.second = manipulator<InvalidHit>();
88 return m_manipulator->cast;
93 return m_manipulator->vec_type;
103 m_lastHit = ULONG_MAX;
111 WrappedHits::const_iterator i = m_hits.begin();
112 if ( m_flags.bits.repeatedLookup && m_lastHit < m_hits.size() ) {
113 if ( (p = cmp(*(i+m_lastHit))) != 0 )
return p;
115 for (
size_t cnt=0; i != m_hits.end(); ++i, ++cnt) {
116 if ((p = cmp(*i)) != 0) {
126 Keys::const_iterator i=m_keys.find(
key);
127 if ( i == m_keys.end() )
return 0;
128 m_lastHit = (*i).second;
129 return &m_hits.at(m_lastHit);
134 result->reserve(m_hits.size());
135 for (
size_t j = 0, n = m_hits.size(); j < n; ++j) {
138 if (&cast == &m->
cast)
139 result->emplace_back(w.
release());
141 result->emplace_back(m->
cast.apply_downCast(cast, w.
release()));
143 m_lastHit = ULONG_MAX;
149 result->reserve(m_hits.size());
150 for (
size_t j = 0, n = m_hits.size(); j < n; ++j) {
153 if (&cast == &m->
cast)
154 result->emplace_back(w.
data());
156 result->emplace_back(m->
cast.apply_downCast(cast, w.
data()));
162 result.reserve(m_hits.size());
163 for (
size_t j = 0, n = m_hits.size(); j < n; ++j) {
165 result.emplace_back(w.
release());
167 m_lastHit = ULONG_MAX;
173 result.reserve(m_hits.size());
174 for (
size_t j = 0, n = m_hits.size(); j < n; ++j) {
176 result.emplace_back(w.
data());
Generic class template to compare/select hits in Geant4HitCollection objects.
virtual void clear()
Clear the collection (Deletes all valid references to real hits)
HitManipulator(const ComponentCast &c, const ComponentCast &v)
Initializing Constructor.
void * release()
Pointer/Object release.
static void increment(T *)
Increment count according to type information.
void getHitsUnchecked(std::vector< void * > &result)
Release all hits from the Geant4 container. Ownership stays with the container.
void * findHit(const Compare &cmp)
Find hit in a collection by comparison of attributes.
void * data()
Pointer/Object access.
virtual ~Geant4HitWrapper()
Default destructor.
Generic wrapper class for hit structures created in Geant4 sensitive detectors.
HitManipulator::Wrapper Wrapper
const ComponentCast & vector_type() const
Type information of the vector type for extracting data.
virtual ~Compare()
Default destructor.
static void decrement(T *)
Decrement count according to type information.
Geant4HitWrapper * findHitByKey(VolumeID key)
Find hit in a collection by comparison of the key.
Wrapper releaseData()
Release data for copy.
G4ThreadLocal G4Allocator< Geant4HitWrapper > * HitWrapperAllocator
void releaseHitsUnchecked(std::vector< void * > &result)
Release all hits from the Geant4 container and pass ownership to the caller.
void newInstance()
Notification to increase the instance counter.
const ComponentCast & type() const
Type information of the object stored.
virtual ~Geant4HitCollection()
Default destructor.
dd4hep::DDSegmentation::VolumeID VolumeID
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
const ComponentCast & cast
Generic type manipulation class for generic hit structures created in Geant4 sensitive detectors.
void releaseData(const ComponentCast &cast, std::vector< void * > *result)
Release all hits from the Geant4 container and pass ownership to the caller.
HitManipulator * manip() const
Access to cast grammar.
~HitManipulator()
Default destructor.
void getData(const ComponentCast &cast, std::vector< void * > *result)
Release all hits from the Geant4 container. Ownership stays with the container.
Wrapper m_data
Wrapper data.