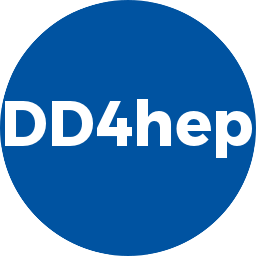 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4DATA_H
15 #define DDG4_GEANT4DATA_H
18 #include <Math/Vector3D.h>
33 typedef ROOT::Math::XYZVector
Position;
40 class Geant4FastSimSpot;
72 typedef std::vector<long>
Seeds;
151 float x = 0.0,
y = 0.0,
z = 0.0;
162 MonteCarloContrib(
int track_id,
int pdg,
double dep,
double time_stamp,
double len)
167 MonteCarloContrib(
int track_id,
int pdg,
double dep,
double time_stamp,
double len,
float* pos,
float* mom)
170 x(pos[0]),
y(pos[1]),
z(pos[2]),
px(mom[0]),
py(mom[1]),
pz(mom[2])
174 MonteCarloContrib(
int track_id,
int pdg,
double dep,
double time_stamp,
double len,
double* pos,
double* mom)
176 x(float(pos[0])),
y(float(pos[1])),
z(float(pos[2])),
177 px(float(mom[0])),
py(float(mom[1])),
pz(float(mom[2]))
183 x(float(pos.
x())),
y(float(pos.
y())),
z(float(pos.
z())),
184 px(float(mom.
x())),
py(float(mom.
y())),
pz(float(mom.
z()))
285 Hit(
int track_id,
int pdg_id,
double deposit,
double time_stamp,
double len=0.0,
const Position& p={0.0, 0.0, 0.0},
const Direction& d={0.0, 0.0, 0.0});
299 Hit&
storePoint(
const G4Step* step,
const G4StepPoint* point);
358 #endif // DDG4_GEANT4DATA_H
Direction momentum
Hit direction.
SimpleRun()
Default constructor.
Position position
Hit position.
MonteCarloContrib(MonteCarloContrib &&c)=default
Move constructor.
float px
Proper particle momentum when generating the hit of the contributing particle.
Generic user-extendible data extension class.
long flag
User flag to classify hits.
MonteCarloContrib Contribution
Direction momentum() const
Access momentum.
int pdgID
Particle ID from the PDG table.
virtual ~Hit()
Default destructor.
Seeds seeds
Random number generator seeds.
Hit(Hit &&c)=delete
Move constructor.
DataExtension()
Default constructor.
double length
Length of this step.
int runID
Run identifiers.
MonteCarloContrib & operator=(MonteCarloContrib &&c)=default
Assignment operator (move)
Position position() const
Access position.
Geant4Calorimeter SimpleCalorimeter
std::unique_ptr< DataExtension > extension
User data extension if required.
MonteCarloContrib(int track_id, int pdg, double dep, double time_stamp, double len, const Position &pos, const Direction &mom)
Initializing constructor.
Simple event description structure. Used in the default I/O mechanism.
Hit(const Hit &c)=delete
copy constructor
void setPosition(const Position &pos)
Set position of the contribution.
void copyFrom(const Hit &c)
Explicit assignment operation.
virtual ~Hit()
Default destructor.
MonteCarloContrib(int track_id, int pdg, double dep, double time_stamp, double len, double *pos, double *mom)
Initializing constructor.
long long int cellID
cellID
Spot definition for fast simulation and GFlash.
virtual ~Geant4HitData()
Default destructor.
Hit & operator=(const Hit &c)=delete
Copy assignment operator.
std::vector< MonteCarloContrib > Contributions
MonteCarloContrib(const MonteCarloContrib &c)=default
Copy constructor.
DDG4 tracker hit class used by the generic DDG4 tracker sensitive detector.
SimpleEvent()
Default constructor.
double length
Length of the track segment contributing to this hit.
long g4ID
Original Geant 4 track identifier of the creating track (debugging)
Hit()
Default constructor.
double deposit
Total energy deposit in this hit.
Hit & operator=(Hit &&c)=delete
Move assignment operator.
Base class for geant4 hit structures used by the default DDG4 sensitive detector implementations.
virtual ~SimpleRun()
Default destructor.
Hit()
Default constructor (for ROOT)
Helper class to define structures used by the generic DDG4 calorimeter sensitive detector.
MonteCarloContrib()=default
Default constructor.
Hit & operator=(const Hit &c)=delete
Copy assignment operator.
Contribution truth
Monte Carlo / Geant4 information.
Geant4HitData()
Default constructor.
Helper class to define structures used by the generic DDG4 tracker sensitive detector.
void setMomentum(const Direction &dir)
Set memonetum of the contribution.
double energyDeposit
Total energy deposit.
DDG4 calorimeter hit class used by the generic DDG4 calorimeter sensitive detector.
Hit(Hit &&c)=delete
Move constructor.
int trackID
Geant 4 Track identifier.
void clear()
Clear data content.
int numEvents
Number of events in this run.
ROOT::Math::XYZVector Position
Geant4HitData SimpleHit
Backward compatibility definitions.
Geant4Tracker SimpleTracker
float x
Proper position of the hit contribution.
virtual ~DataExtension()
Default destructor.
void setPosition(double pos_x, double pos_y, double pos_z)
Set position of the contribution.
Contributions truth
Hit contributions by individual particles.
std::vector< long > Seeds
int runID
Run identifiers.
Hit(const Hit &c)=delete
copy constructor
Namespace for the AIDA detector description toolkit.
static Contribution extractContribution(const G4Step *step)
Extract the MC contribution for a given hit from the step information.
Hit & storePoint(const G4Step *step, const G4StepPoint *point)
Store Geant4 point and step information into tracker hit structure.
Utility class describing the monte carlo contribution of a given particle to a hit.
double energyDeposit
Energy deposit in the tracker hit.
Hit & operator=(Hit &&c)=delete
Move assignment operator.
int eventID
Event identifier.
Hit & clear()
Clear hit content.
Simple run description structure. Used in the default I/O mechanism.
MonteCarloContrib & operator=(const MonteCarloContrib &c)=default
Assignment operator (copy)
double time
Timestamp when this energy was deposited.
Position position
Hit position.
void setMomentum(double mom_x, double mom_y, double mom_z)
Set memonetum of the contribution.
virtual ~SimpleEvent()
Default destructor.