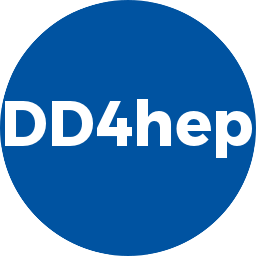 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4ACTIONPHASE_H
14 #define DDG4_GEANT4ACTIONPHASE_H
70 typedef std::vector<std::pair<Geant4Action*, Callback> >
Members;
75 const std::type_info*
m_argTypes[3] = {
nullptr,
nullptr,
nullptr };
80 const std::type_info& arg_type1,
const std::type_info& arg_type2);
88 const std::type_info*
const *
argTypes()
const {
98 template <
typename TYPE,
typename IF_TYPE,
typename A0,
typename R>
99 bool add(TYPE* member, R (IF_TYPE::*callback)(A0 arg)) {
100 typeinfoCheck(
typeid(A0), *
m_argTypes[0],
"Invalid ARG0 type. Failed to add phase callback.");
101 if (
dynamic_cast<IF_TYPE*
>(member)) {
102 return add(member,
Callback(member).make(callback));
104 throw unrelated_type_error(
typeid(TYPE),
typeid(IF_TYPE),
"Failed to add phase callback.");
107 template <
typename TYPE,
typename IF_TYPE,
typename A0,
typename A1,
typename R>
108 bool add(TYPE* member, R (IF_TYPE::*callback)(A0 arg0, A1 arg1)) {
109 typeinfoCheck(
typeid(A0), *
m_argTypes[0],
"Invalid ARG0 type. Failed to add phase callback.");
110 typeinfoCheck(
typeid(A1), *
m_argTypes[1],
"Invalid ARG1 type. Failed to add phase callback.");
111 if (
dynamic_cast<IF_TYPE*
>(member)) {
112 return add(member,
Callback(member).make(callback));
114 throw unrelated_type_error(
typeid(TYPE),
typeid(IF_TYPE),
"Failed to add phase callback.");
117 template <
typename TYPE,
typename IF_TYPE,
typename A0,
typename A1,
typename A2,
typename R>
118 bool add(TYPE* member, R (IF_TYPE::*callback)(A0 arg0, A1 arg1)) {
119 typeinfoCheck(
typeid(A0), *
m_argTypes[0],
"Invalid ARG0 type. Failed to add phase callback.");
120 typeinfoCheck(
typeid(A1), *
m_argTypes[1],
"Invalid ARG1 type. Failed to add phase callback.");
121 typeinfoCheck(
typeid(A2), *
m_argTypes[2],
"Invalid ARG2 type. Failed to add phase callback.");
122 if (
dynamic_cast<IF_TYPE*
>(member)) {
124 return add(member,
Callback(member).make(callback));
126 throw unrelated_type_error(
typeid(TYPE),
typeid(IF_TYPE),
"Failed to add phase callback.");
129 template <
typename TYPE,
typename PMF>
bool remove(TYPE* member) {
133 template <
typename TYPE,
typename PMF>
bool remove(TYPE* member, PMF callback) {
139 template <
typename A0>
void call(A0 a0);
140 template <
typename A0,
typename A1>
void call(A0 a0, A1 a1);
141 template <
typename A0,
typename A1,
typename A2>
void call(A0 a0, A1 a1, A2 a2);
147 #endif // DDG4_GEANT4ACTIONPHASE_H
bool add(TYPE *member, R(IF_TYPE::*callback)(A0 arg))
Add a new member to the phase.
bool add(TYPE *member, R(IF_TYPE::*callback)(A0 arg0, A1 arg1))
Add a new member to the phase.
bool remove(TYPE *member, PMF callback)
Remove an existing member callback from the phase. If not existing returns false.
std::vector< std::pair< Geant4Action *, Callback > > Members
void call(A0 a0, A1 a1, A2 a2)
const std::type_info *const * argTypes() const
Type of the first phase callback-argument.
Action phase definition. Client callback at various stage of the simulation processing.
Geant4PhaseAction(Geant4Context *context, const std::string &name)
Standard constructor.
bool remove(TYPE *member)
Remove all member callbacks from the phase. If not existing returns false.
Members m_members
Phase members (actions) being called for a particular phase.
const Callback & make(R(T::*pmf)())
Callback setup function for Callbacks with member functions with explicit return type taking no argum...
virtual bool add(Geant4Action *action, Callback callback)
Add a new member to the phase.
virtual void operator()()
Callback to generate primary particles.
bool add(TYPE *member, R(IF_TYPE::*callback)(A0 arg0, A1 arg1))
Add a new member to the phase.
Definition of the generic callback structure for member functions.
virtual Callback callback()
Create bound callback to operator()()
Default base class for all Geant 4 actions and derivates thereof.
const std::string & name() const
Access name of the action.
const Members & members() const
Access phase members.
Generic action for Geant4 phases.
void call()
Create action to execute phase members.
void execute(void *argument)
Execute all members in the phase context.
Namespace for the AIDA detector description toolkit.
virtual bool remove(Geant4Action *action, Callback callback)
Remove an existing member from the phase. If not existing returns false.
Geant4ActionPhase(Geant4Context *context, const std::string &name, const std::type_info &arg_type0, const std::type_info &arg_type1, const std::type_info &arg_type2)
Standard constructor.
const std::type_info * m_argTypes[3]
Type information of the argument type of the callback.
virtual ~Geant4PhaseAction()
Default destructor.
virtual ~Geant4ActionPhase()
Default destructor.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.