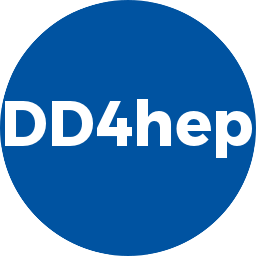 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
43 printout(FATAL,
"Geant4EventReader",
"No input action registered!");
44 throw std::runtime_error(
"Geant4EventReader: No input action registered!");
60 std::vector<Particle*> particles;
65 for_each(particles.begin(),particles.end(),detail::deleteObject<Particle>);
66 for_each(vertices.begin(),vertices.end(),detail::deleteObject<Vertex>);
73 if( parameters.empty() ) {
76 for (
auto const& pairNV : parameters ) {
77 printout(FATAL,
"EventReader::checkParameters",
"Unknown parameter name: %s with value %s",
79 pairNV.second.c_str());
81 throw std::runtime_error(
"Unknown parameter for event reader");
88 if ( event_number >= INT_MIN ) {
122 declareProperty(
"Input", m_input);
123 declareProperty(
"Sync", m_firstEvent=0);
124 declareProperty(
"Mask", m_mask = 0);
125 declareProperty(
"MomentumScale", m_momScale = 1.0);
126 declareProperty(
"HaveAbort", m_abort =
true);
127 declareProperty(
"Parameters", m_parameters = {});
128 declareProperty(
"AlternativeDecayStatuses", m_alternativeDecayStatuses = {});
129 m_needsControl =
true;
148 except(
"InputAction: No input file declared!");
157 abortRun(
"Error creating reader plugin.",
158 "Failed to create file reader of type %s. Cannot open dataset %s",
168 if ( !err.empty() ) {
169 abortRun(err,
"Error when creating reader for file %s",
m_input.c_str());
176 std::stringstream str;
177 str <<
"Geant4InputAction[" <<
name() <<
"]: Event " << i <<
" ";
184 std::vector<Particle*>& particles)
199 std::string msg =
issue(evid)+
"Error when moving to event - ";
201 else msg +=
" Unknown error condition";
220 std::string msg =
issue(evid)+
"Error when moving to event - ";
222 else msg +=
" Unknown error condition";
235 std::vector<Particle*> primaries;
254 if ( primaries.empty() )
return;
257 if ( vertices.empty() )
return;
259 print(
"+++ Particle interaction with %d generator particles and %d vertices ++++++++++++++++++++++++",
260 int(primaries.size()),
int(vertices.size()) );
263 for(
size_t i=0; i<vertices.size(); ++i ) {
268 for(
auto* primPart : primaries) {
272 p->psx = mom_scale*p->psx;
273 p->psy = mom_scale*p->psy;
274 p->psz = mom_scale*p->psz;
Class modelling a complete primary event with multiple interactions.
Geant4Context * context() const
Get the context (from the input action)
EventReaderStatus
Status codes of the event reader object. Anything with NOT low-bit set is an error.
void exception(const std::string &src, const std::string &msg)
Class modelling a single interaction with multiple primary vertices and particles.
Property & property(const std::string &name)
Access single property.
void abortRun(const std::string &exception, const char *fmt,...) const
Abort Geant4 Run by throwing a G4Exception with type RunMustBeAborted.
VertexMap vertices
The map of primary vertices for the particles.
ParticleMap particles
The map of particles participating in this primary interaction.
virtual ~Geant4EventReader()
Default destructor.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
UriReader * m_reader
Pointer to URI reader.
@ G4PARTICLE_GEN_DOCUMENTATION
int m_currEvent
Current event number.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
virtual EventReaderStatus readParticles(int event_number, Vertices &vertices, Particles &particles)=0
Read an event and fill a vector of MCParticles.
void error(const char *fmt,...) const
Support of error messages.
virtual EventReaderStatus moveToEvent(int event_number)
Move to the indicated event number.
virtual void registerRunParameters()
Register Run Parameters.
Concrete implementation of the Geant4 generator action base class.
virtual EventReaderStatus skipEvent()
Skip event. To be implemented for sequential sources.
virtual EventReaderStatus setParameters(std::map< std::string, std::string > &)
pass parameters to the event reader object
const std::string & name() const
Access name of the action.
Geant4InputAction * m_inputAction
The input action context.
PrintLevel outputLevel() const
Access the output level.
static TypeName split(const std::string &type_name)
Split string pair according to default delimiter ('/')
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
virtual void checkParameters(std::map< std::string, std::string > &)
make sure that all parameters have been processed, otherwise throw exceptions
TYPE value() const
Retrieve value.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
Helper class to indicate the end of the input file.
Data structure to access derived MC particle information.
Helper class to handle strings of the format "type/name".
dd4hep::detail::ReferenceBitMask< int > PropertyMask
std::vector< Vertex * > Vertices
void setInputAction(Geant4InputAction *action)
Set the input action.
bool hasDirectAccess() const
Flag if direct event access (by event sequence number) is supported (Default: false)
Geant4EventReader(const std::string &nam)
Initializing constructor.
User event context for DDG4.
void add(int id, Geant4PrimaryInteraction *interaction)
Add a new interaction object to the event.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.