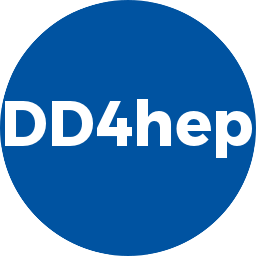 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #ifndef DDG4_GEANT4INPUTACTION_H
25 #define DDG4_GEANT4INPUTACTION_H
31 #include <Parsers/Parsers.h>
93 std::string
const& parameterName,
94 T& parameter, T defaultValue ) {
96 if( parameters.find( parameterName ) != parameters.end() ) {
98 parameters.erase( parameterName );
100 parameter = std::move(defaultValue);
213 #endif // DDG4_GEANT4INPUTACTION_H
Geant4Context * context() const
Get the context (from the input action)
EventReaderStatus
Status codes of the event reader object. Anything with NOT low-bit set is an error.
std::vector< Particle * > Particles
void _getParameterValue(std::map< std::string, std::string > ¶meters, std::string const ¶meterName, T ¶meter, T defaultValue)
transform the string parameter value into the type of parameter
virtual ~Geant4EventReader()
Default destructor.
int m_currEvent
Current event number.
virtual EventReaderStatus readParticles(int event_number, Vertices &vertices, Particles &particles)=0
Read an event and fill a vector of MCParticles.
virtual EventReaderStatus moveToEvent(int event_number)
Move to the indicated event number.
@ EVENT_READER_NO_FACTORY
virtual void registerRunParameters()
Register Run Parameters.
Concrete implementation of the Geant4 generator action base class.
int currentEventNumber() const
return current Event Number
virtual EventReaderStatus skipEvent()
Skip event. To be implemented for sequential sources.
virtual EventReaderStatus setParameters(std::map< std::string, std::string > &)
pass parameters to the event reader object
int parse(Property &result, const std::string &input)
std::string m_name
File name to be opened and read.
bool m_directAccess
Flag if direct event access is supported. To be explicitly set by subclass constructors.
const std::string & name() const
Access name of the action.
Geant4InputAction * m_inputAction
The input action context.
Basic geant4 event reader class. This interface/base-class must be implemented by concrete readers.
virtual void checkParameters(std::map< std::string, std::string > &)
make sure that all parameters have been processed, otherwise throw exceptions
Namespace for the AIDA detector description toolkit.
@ EVENT_READER_NO_PRIMARIES
Data structure to store the MC particle information.
Data structure to store the MC vertex information.
std::vector< Vertex * > Vertices
void setInputAction(Geant4InputAction *action)
Set the input action.
bool hasDirectAccess() const
Flag if direct event access (by event sequence number) is supported (Default: false)
Geant4EventReader(const std::string &nam)
Initializing constructor.
const std::string & name() const
File name.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.