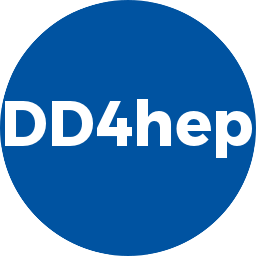 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DDG4_GEANT4HITDUMPACTION_H
14 #define DD4HEP_DDG4_GEANT4HITDUMPACTION_H
54 virtual void begin(
const G4Event* event)
override;
56 virtual void end(
const G4Event* event)
override;
83 #include <G4HCofThisEvent.hh>
92 m_needsControl =
true;
93 declareProperty(
"Collections",m_containers);
109 std::string nam = collection->GetName();
114 size_t nhits = coll->
GetSize();
115 for(
size_t i=0; i<nhits; ++i) {
118 if ( 0 != trk_hit ) {
119 trk_hits.emplace_back(trk_hit);
122 if ( 0 != cal_hit ) {
123 cal_hits.emplace_back(cal_hit);
126 if ( !trk_hits.empty() )
127 dump.
print(ALWAYS,nam,&trk_hits);
128 if ( !cal_hits.empty() )
129 dump.
print(ALWAYS,nam,&cal_hits);
135 G4HCofThisEvent* hce =
event->GetHCofThisEvent();
137 int nCol = hce->GetNumberOfCollections();
139 for (
int i = 0; i < nCol; ++i) {
146 warning(
"+++ [Event:%d] The value of G4HCofThisEvent is NULL.",event->GetEventID());
Geant4HitDumpAction(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual void end(const G4Event *event) override
Geant4EventAction interface: End-of-event callback.
virtual size_t GetSize() const override
Access the collection size.
virtual ~Geant4HitDumpAction()
Default destructor.
virtual void begin(const G4Event *event) override
Geant4EventAction interface: Begin-of-event callback.
Class to dump the records of the intrinsic Geant4 event model.
Generic hit container class using Geant4HitWrapper objects.
Geant4HitWrapper & hit(size_t which)
Access the hit wrapper.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
static void increment(T *)
Increment count according to type information.
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
void warning(const char *fmt,...) const
Support of warning messages.
Data structure to map particles produced during the generation and the simulation.
std::vector< SimpleCalorimeter::Hit * > CalorimeterHits
Concrete basic implementation of the Geant4 event action.
DDG4 tracker hit class used by the generic DDG4 tracker sensitive detector.
std::vector< SimpleTracker::Hit * > TrackerHits
Base class for geant4 hit structures used by the default DDG4 sensitive detector implementations.
static void decrement(T *)
Decrement count according to type information.
const std::string & name() const
Access name of the action.
DDG4 calorimeter hit class used by the generic DDG4 calorimeter sensitive detector.
CollectionNames m_containers
Property: collection names to be dumped.
std::vector< std::string > CollectionNames
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Namespace for the AIDA detector description toolkit.
Class to measure the energy of escaping tracks.
void dumpCollection(G4VHitsCollection *hc)
Dump single container of hits.
void print(PrintLevel level, Geant4ParticleHandle p) const
Print a single particle to the output logging using the specified print level.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.