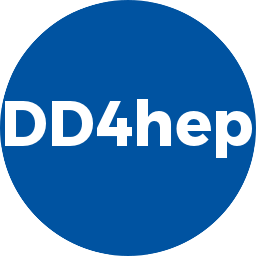 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
33 printout(level,
m_tag,
" +++ TrackID: %6d %12d %6d %-7s %3s %5d %6s %8.3g %-4s %-7s %-7s %-3s",
53 printout(level,
m_tag,
" +++ TrackID: %6d [key:%d] %12d %6d %-7s %3s %5d %6s %8.3g %-4s %-7s %-7s %-3s",
72 PrintLevel low_lvl = level == ALWAYS ? ALWAYS : PrintLevel(level-1);
73 printout(level,
m_tag,
"+++ Track container: %-21s --------------- Track KEEP reasoning ---------------",container.c_str());
74 printout(level,
m_tag,
"+++ # of Tracks:%6d PDG Parent Primary Secondary Energy %-8s Calo Tracker Process/Par",
75 int(parts->size()),
"in [MeV]");
86 PrintLevel low_lvl = level == ALWAYS ? ALWAYS : PrintLevel(level-1);
88 const ParticleMap& pm = parts->
particles();
89 printout(level,
m_tag,
"+++ Geant4 Particle map %-18s --------------- Track KEEP reasoning ---------------",
"");
90 printout(level,
m_tag,
"+++ # of Tracks:%6d PDG Parent Primary Secondary Energy %-8s Calo Tracker Process/Par",
91 int(pm.size()),
"in [MeV]");
92 for(
const auto& p : pm)
93 print(low_lvl, p.first, p.second);
100 printout(level,
m_tag,
" +++ Hit: Cell: %016llX Pos:(%9.3g,%9.3g,%9.3g) Len:%9.3g [mm] E:%9.3g MeV TrackID:%6d PDG:%12d dep:%9.3g time:%9.3g [ns]",
107 PrintLevel low_lvl = level == ALWAYS ? ALWAYS : PrintLevel(level-1);
108 printout(level,
m_tag,
"+++ Hit Collection: %s # Tracker hits %d",container.c_str(),
int(hits->size()));
116 PrintLevel low_lvl = level == ALWAYS ? ALWAYS : PrintLevel(level-1);
117 printout(level,
m_tag,
" +++ Hit: Cell: %016llX Pos:(%9.3g,%9.3g,%9.3g) [mm] E:%9.3g MeV #Contributions:%3d",
121 printout(low_lvl,
m_tag,
" Contribution #%3d TrackID:%6d PDG:%12d %9.3g MeV %9.3g ns",
130 PrintLevel low_lvl = level == ALWAYS ? ALWAYS : PrintLevel(level-1);
131 printout(level,
m_tag,
"+++ Hit Collection: %s # Calorimeter hits %d",container.c_str(),
int(hits->size()));
virtual ~Geant4DataDump()
Standard destructor.
@ G4PARTICLE_CREATED_TRACKER_HIT
Position position
Hit position.
@ G4PARTICLE_ABOVE_ENERGY_THRESHOLD
int pdgID
Particle ID from the PDG table.
const ParticleMap & particles() const
Access the particle map.
@ G4PARTICLE_KEEP_PROCESS
Geant4DataDump(const std::string &tag)
Default constructor.
long long int cellID
cellID
Data structure to map particles produced during the generation and the simulation.
std::vector< SimpleCalorimeter::Hit * > CalorimeterHits
std::string m_tag
Tag variable.
DDG4 tracker hit class used by the generic DDG4 tracker sensitive detector.
double length
Length of the track segment contributing to this hit.
std::map< int, Particle * > ParticleMap
std::vector< SimpleTracker::Hit * > TrackerHits
double deposit
Total energy deposit in this hit.
Particles daughters
The list of daughters of this MC particle.
Contribution truth
Monte Carlo / Geant4 information.
double energyDeposit
Total energy deposit.
DDG4 calorimeter hit class used by the generic DDG4 calorimeter sensitive detector.
int trackID
Geant 4 Track identifier.
@ G4PARTICLE_HAS_SECONDARIES
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
dd4hep::detail::ReferenceBitMask< int > PropertyMask
Contributions truth
Hit contributions by individual particles.
Data structure to access derived MC particle information.
Particles parents
The list of parents of this MC particle.
@ G4PARTICLE_CREATED_CALORIMETER_HIT
Utility class describing the monte carlo contribution of a given particle to a hit.
double energyDeposit
Energy deposit in the tracker hit.
Data structure to store the MC particle information.
std::vector< Particle * > Particles
double energy() const
Scalar particle energy.
double time
Timestamp when this energy was deposited.
Position position
Hit position.
void print(PrintLevel level, Geant4ParticleHandle p) const
Print a single particle to the output logging using the specified print level.