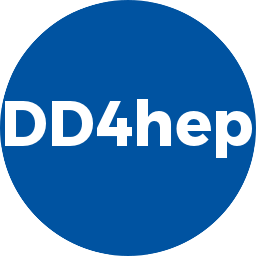 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETELEMENTVOLUMEIDS_H
14 #define DD4HEP_DETELEMENTVOLUMEIDS_H
50 std::map<DetElement, std::vector<Encoding> >
entries;
69 #endif // DD4HEP_DETELEMENTVOLUMEIDS_H
103 long assign_de_volumeIDs(
Detector& description,
int argc,
char** argv) {
104 std::string detector =
"/world";
105 for(
int i = 0; i < argc && argv[i]; ++i) {
106 if ( 0 == ::strncmp(
"-detector",argv[i],4) )
107 detector = argv[++i];
110 "Usage: -plugin DD4hep_DetElementVolumeIDs -arg [-arg] \n\n"
111 " -detector <string> Top level DetElement path. Default: '/world' \n"
112 " -help Print this help output \n"
113 " Arguments given: " << arguments(argc,argv) << std::endl << std::flush;
118 if ( detector !=
"/world" ) {
121 except(
"DD4hep_DetElementVolumeIDs",
"+++ Invalid DetElement path: %s",detector.c_str());
125 auto count = mgr.populate(element);
127 except(
"DD4hep_DetElementVolumeIDs",
128 "+++ NO volume identifiers assigned to DetElement %s. %s",
129 "Something went wrong!",detector.c_str());
131 return count > 0 ? 1 : 0;
134 DECLARE_APPLY(DD4hep_DetElementVolumeIDs,assign_de_volumeIDs)
144 for (VolIDs::const_iterator i = ids.begin(); i != ids.end(); ++i) {
145 const auto&
id = (*i);
150 volume_id |= ((f->
value(val << off) << off)&msk);
153 return { volume_id, mask };
159 : m_detDesc(description)
165 std::size_t count = 0UL;
171 except(
"DetElementVolumeIDs",
172 "+++ Top level DetElement %s has no valid placement. %s",
173 "[Something awfully wrong]",
det.path().c_str());
176 for (
const auto& i :
det.children() ) {
186 printout(WARNING,
"DetElementVolumeIDs",
"++ Detector element %s of type %s has no placement.",
189 printout(INFO,
"DetElementVolumeIDs",
"++ Assigned %ld volume identifiers to DetElements.", count);
194 except(
"DetElementVolumeIDs",
195 "+++ No sensitive detector available for top level DetElement %s.",
200 printout(INFO,
"DetElementVolumeIDs",
"++ Assigned %ld volume identifiers to DetElements.", count);
213 TGeoNode* node = pv.
ptr();
214 std::size_t count = 0;
218 Encoding vol_encoding = parent_encoding;
220 bool have_encoding = pv_ids.empty();
221 bool compound = e.
type() ==
"compound";
235 chain.emplace_back(node);
236 if ( sd.
isValid() && !pv_ids.empty() ) {
239 vol_encoding = update_encoding(ro.
idSpec(), pv_ids, parent_encoding);
240 have_encoding =
true;
243 printout(WARNING,
"DetElementVolumeIDs",
244 "%s: Strange constellation volume %s is sensitive, but has no readout! sd:%p",
248 for (
int idau = 0, ndau = node->GetNdaughters(); idau < ndau; ++idau) {
249 TGeoNode* daughter = node->GetDaughter(idau);
251 if ( place_dau.
data() ) {
256 for(
const auto& de : e.
children() ) {
257 if ( de.second.placement().ptr() == daughter ) {
271 except(
"DetElementVolumeIDs",
272 "Invalid not instrumented placement: %s %s", daughter->GetName(),
273 " [Internal error -- bad detector constructor]");
282 if ( !have_encoding && !compound ) {
283 printout(ERROR,
"DetElementVolumeIDs",
284 "Element %s: Missing SD encoding. Volume manager won't work!",
287 if ( is_sensitive || count > 0 ) {
300 entries[e].emplace_back(vol_encoding);
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
Helper class for BitFieldCoder that corresponds to one field value.
Handle class to hold the information of a sensitive detector.
Actor class to assign volume identifiers to DetElements in a subdetector tree.
const BitFieldElement * field(const std::string &field_name) const
Get the field descriptor of one field by name.
std::string type() const
Access detector type (structure, tracker, calorimeter, etc.).
Handle class holding a placed volume (also called physical volume)
Q & object() const
Access to an unrelated object type.
PlacedVolume placement() const
Access to the physical volume of this detector element.
Class implementing the ID encoding of the detector response.
FieldID value(CellID bitfield) const
calculate this field's value given an external 64 bit bitmap
#define DECLARE_APPLY(name, func)
bool isValid() const
Check the validity of the object held by the handle.
std::size_t scanPhysicalVolume(DetElement &parent, DetElement e, PlacedVolume pv, Encoding parent_encoding, SensitiveDetector &sd, PlacementPath &chain)
Scan a single physical volume and look for sensitive elements below.
bool isSensitive() const
Accessor if volume is sensitive (ie. is attached to a sensitive detector)
const char * name() const
Access the object name (or "" if not supported by the object)
Data class with properties of a detector element.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
Encoding/mask for sensitive volumes.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
std::size_t numberOfNodes
Node counter.
std::map< DetElement, std::vector< Encoding > > entries
Set of already added entries.
const Detector & m_detDesc
Reference to the Detector instance.
dd4hep::DDSegmentation::VolumeID VolumeID
T * ptr() const
Access to the held object.
std::vector< PlacedVolume > PlacementPath
Namespace for the AIDA detector description toolkit.
Readout readout() const
Access readout structure of the sensitive detector.
Volume volume() const
Logical volume of this placement.
Object * data() const
Check if placement is properly instrumented.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
DetElementVolumeIDs::Encoding Encoding
IDDescriptor idSpec() const
Access IDDescription structure.
std::size_t populate(DetElement e)
Populate the Volume manager.
const PlacedVolumeExtension::VolIDs & volIDs() const
Access to the volume IDs.
@ HAVE_SENSITIVE_DETECTOR
DetElementVolumeIDs(const Detector &description)
Default constructor.
Handle< NamedObject > sensitiveDetector() const
Access to the handle to the sensitive detector.