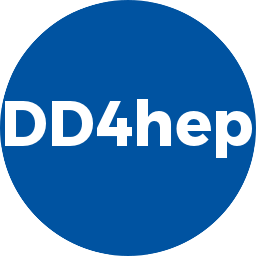 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_CONDITIONSMAPPEDPOOL_H
14 #define DDCOND_CONDITIONSMAPPEDPOOL_H
25 #include <unordered_map>
46 template<
typename MAPPING,
typename BASE>
57 template <
typename R,
typename T> std::size_t
loop(R& result, T functor) {
58 size_t len = result.size();
60 return result.size() - len;
70 virtual size_t size() const final {
78 if ( result )
return true;
82 printout(ERROR,
"MappedPool",
"ConditionsClash: %s %08llX <> %08llX %s",
83 present.
name(), present.
key(), condition.key(), condition.name());
149 entries[o.second->iov].emplace_back(o.second);});
159 unsigned int req_typ = req.iovType ? req.iovType->type : req.type;
160 const IOV::Key& req_key = req.key();
161 result.reserve(m.size());
162 for(
const auto& e : m) {
167 if ( req_typ == typ ) {
170 result.emplace_back(o);
173 result.emplace_back(o);
176 result.emplace_back(o);
208 template<
typename MAPPING,
typename BASE>
210 this->BASE::SetName(
"");
211 this->BASE::SetTitle(
"ConditionsMappedPool");
216 template<
typename MAPPING,
typename BASE>
229 dd4hep::except(
"ConditionsMappedPool",
"++ Insufficient arguments: arg[0] = ConditionManager!");
232 #define _CR(fun,x,b,y) void* fun(dd4hep::Detector&, int argc, char** argv) \
233 { return new b<x<dd4hep::Condition::key_type,dd4hep::Condition::Object*>,y>(_mgr(argc,argv)); }
ConditionsOperation< KeyedSelect< C > > keyedSelect(Condition::key_type key, C &coll)
Helper to create functor to select keyed objects from a conditions pool.
std::vector< Condition > RangeConditions
virtual size_t select(Condition::key_type key, RangeConditions &result) final
Select the conditions matching the DetElement and the conditions name.
AlignmentCondition::Object * cond
ConditionsMappedPool(ConditionsManager mgr)
Default constructor.
ConditionsOperation< KeyFind > keyFind(Condition::key_type key)
Helper to create functor to find conditions objects by hash key.
virtual bool insert(Condition condition) final
Register a new condition to this pool.
Condition::key_type hash
Hash value of the name.
OperatorWrapper< oper_type > operatorWrapper(oper_type &oper)
Helper function to create a OperatorWrapper<T> object from the argument type.
virtual ~ConditionsMappedUpdatePool()
Default destructor.
virtual size_t size() const final
Total entry count.
virtual void select_range(Condition::key_type key, const IOV &req, RangeConditions &result) final
Select the conditions matching the DetElement and the conditions name.
ConditionsMappedPool< MAPPING, BASE > Self
ConditionsOperation< PoolRemove< P > > poolRemove(P &pool)
Helper to create functor to remove objects from a conditions pool.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
virtual void insert(RangeConditions &new_entries) final
Register a new condition to this pool. May overload for performance reasons.
key_type key() const
Hash identifier.
std::pair< Key_value_type, Key_value_type > Key
static void increment(T *)
Increment count according to type information.
const char * name() const
Access the object name (or "" if not supported by the object)
Conditions selector functor. Default implementation selects everything evaluated.
virtual size_t popEntries(UpdatePool::UpdateEntries &entries) final
Adopt all entries sorted by IOV. Entries will be removed from the pool.
ConditionsMappedUpdatePool(ConditionsManager mgr)
Default constructor.
Basic conditions manager implementation.
Main condition object handle.
Namespace for implementation details of the AIDA detector description toolkit.
Class describing the interval of validty.
virtual size_t select_all(ConditionsPool &result) final
Select the conditions, used also by the DetElement of the condition.
ConditionsOperation< SequenceSelect< C > > sequenceSelect(C &coll)
Helper to create functor to select objects from a conditions pool into a sequential container.
Class implementing the conditions collection for a given IOV type.
static bool key_overlaps_lower_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the lower interval edge with IOV 'key'.
static void decrement(T *)
Decrement count according to type information.
virtual size_t select_all(RangeConditions &result) final
Select the conditions, used also by the DetElement of the condition.
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
static bool key_overlaps_higher_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the upper interval edge with IOV 'key'.
static bool key_is_contained(const Key &key, const Key &test)
Check if IOV 'test' is fully contained in IOV 'key'.
Key key() const
Get the local key of the IOV.
unsigned long long int key_type
Forward definition of the key type.
The data class behind a conditions handle.
virtual Condition exists(Condition::key_type key) const final
Check if a condition exists in the pool.
Manager class for condition handles.
Class implementing the conditions update pool for a given IOV type.
unsigned int type
IOV buffer type: Must be a bitmap!
const IOVType * iovType
Reference to IOV type.
Namespace for the AIDA detector description toolkit.
std::map< const IOV *, ConditionEntries > UpdateEntries
Update container specification.
ConditionsOperation< PoolSelect< P > > poolSelect(P &pool)
Helper to create functor to insert objects into a conditions pool.
Class implementing the conditions collection for a given IOV type.
virtual void clear() final
Full cleanup of all managed conditions.
std::size_t loop(R &result, T functor)
Helper function to loop over the conditions container and apply a functor.
Interface for conditions pool optimized to host conditions updates.
#define _CR(fun, x, b, y)
virtual size_t select_all(const ConditionsSelect &result) final
Select the conditions, used also by the DetElement of the condition.
unsigned int type
integer identifier used internally
const IOV * iov
Interval of validity.
ConditionsMappedPool< Mapping, Base > Self
virtual ~ConditionsMappedPool()
Default destructor.