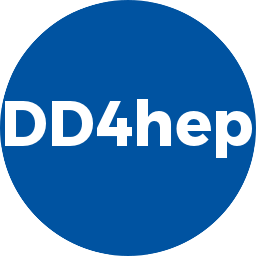 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_CONDITIONSSELECTORS_H
14 #define DDCOND_CONDITIONSSELECTORS_H
39 typedef std::pair<const Condition::key_type,Condition>
mapentry_t;
203 template <
typename oper_type> OperatorWrapper<oper_type>
operatorWrapper(oper_type& oper) {
204 return OperatorWrapper<oper_type>(oper);
213 template <
typename P>
inline ConditionsOperation<PoolRemove<P> >
poolRemove(P& pool)
214 {
return ConditionsOperation<PoolRemove<P> >(PoolRemove<P>(pool)); }
222 template <
typename P>
inline ConditionsOperation<PoolSelect<P> >
poolSelect(P& pool)
223 {
return ConditionsOperation<PoolSelect<P> >(PoolSelect<P>(pool)); }
232 {
return MapConditionsSelect<T>(collection); }
240 template <
typename C>
inline
242 typedef SequenceSelect<C> operator_t;
243 return ConditionsOperation<operator_t>(operator_t(coll));
252 template <
typename C>
inline
253 ConditionsOperation<SequenceSelect<C> >
mapSelect(C& coll) {
254 typedef MapSelect<C> operator_t;
255 return ConditionsOperation<operator_t>(operator_t(coll));
264 template <
typename C>
inline ConditionsOperation<ActiveSelect<C> >
activeSelect(C& coll)
265 {
return ConditionsOperation<ActiveSelect<C> >(ActiveSelect<C>(coll)); }
273 template <
typename C>
inline
275 {
return ConditionsOperation<KeyedSelect<C> >(KeyedSelect<C>(
key, coll)); }
284 {
return ConditionsOperation<KeyFind>(KeyFind(
key)); }
289 #endif // DDCOND_CONDITIONSSELECTORS_H
Mapped container selection operator for conditions mappings.
KeyedSelect(cond_t::key_type k, collection_type &p)
ConditionsOperation< KeyedSelect< C > > keyedSelect(Condition::key_type key, C &coll)
Helper to create functor to select keyed objects from a conditions pool.
AlignmentCondition::Object * cond
bool operator()(const mapentry_t &o) const
bool operator()(Condition::Object *o) const
bool operator()(const ptr_mapentry_t &o) const
bool operator()(const cond_t &o) const
ConditionsOperation< KeyFind > keyFind(Condition::key_type key)
Helper to create functor to find conditions objects by hash key.
Condition::key_type hash
Hash value of the name.
OperatorWrapper< oper_type > operatorWrapper(oper_type &oper)
Helper function to create a OperatorWrapper<T> object from the argument type.
bool operator()(const object_t *o) const
ConditionsOperation< PoolRemove< P > > poolRemove(P &pool)
Helper to create functor to remove objects from a conditions pool.
Condition::mask_type flags
Flags.
bool operator()(object_t *o) const
std::pair< const Condition::key_type, Condition > mapentry_t
Conditions selector functor. Default implementation selects everything evaluated.
Helper to select condition objects by hash key from a conditions pool.
KeyFind(cond_t::key_type h)
Helper to insert objects into a conditions pool.
Main condition object handle.
bool operator()(object_t *o) const
ConditionsOperation(const operator_t&o)
ConditionsOperation< SequenceSelect< C > > sequenceSelect(C &coll)
Helper to create functor to select objects from a conditions pool into a sequential container.
Arbitrary wrapper for user defined conditions operators.
Helper to wrap another object and make it copyable.
virtual bool operator()(Condition::Object *o) const
Overloadable entry: Selection callback: return true if the condition should be selected.
Definition of the selector object base class to cover type definitions.
bool operator()(Condition::Object *o) const
virtual size_t size() const
Overloadable entry: Return number of conditions selected. Default does nothing....
unsigned long long int key_type
Forward definition of the key type.
The data class behind a conditions handle.
Sequential container select operator for conditions mappings.
bool operator()(const ptr_mapentry_t &o) const
bool operator()(object_t *o) const
MapConditionsSelect< T > mapConditionsSelect(T &collection)
Helper to create functor to collect conditions using a ConditionsSelect base class.
bool operator()(Condition::Object *o) const
Container select operator for conditions mappings with conditions flagged active.
bool operator()(const cond_t &o) const
ConditionsOperation< SequenceSelect< C > > mapSelect(C &coll)
Helper to create functor to select objects from a conditions pool into a mapped container.
bool operator()(const mapentry_t &o) const
T * ptr() const
Access to the held object.
Helper to remove objects from a conditions pool. The removed condition is deleted.
Condition::Object object_t
Helper to select keyed objects from a conditions pool.
OperatorWrapper< operator_t> wrapper_t
Namespace for the AIDA detector description toolkit.
bool operator()(object_t *o) const
Helper class for common stuff used frequently.
bool operator()(object_t *o) const
ConditionsOperation< PoolSelect< P > > poolSelect(P &pool)
Helper to create functor to insert objects into a conditions pool.
collection_type & collection
ConditionsOperation< operator_t> wrapper_t
MapConditionsSelect(T &o)
ConditionsOperation< ActiveSelect< C > > activeSelect(C &coll)
Helper to select active objects from a conditions pool.
std::pair< const Condition::key_type, object_t * > ptr_mapentry_t
OperatorWrapper(operator_t&o)
Helper to collect conditions using a ConditionsSelect base class.