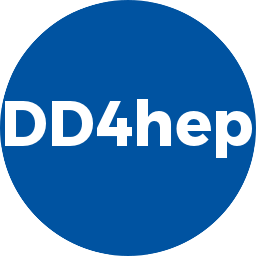 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
47 : description(
det), slice(cond_map)
63 std::pair<dd4hep::Condition::key_type,AlignmentsCalib::Entry*>
66 UsedConditions::iterator i =
used.find(tar_key.
hash);
67 if ( i !=
used.end() ) {
68 (*i).second->delta =
delta;
80 src_cond->hash =
key.hash;
86 except(
"AlignmentsCalib",
87 "++ The SOURCE alignment condition [%016llX]: %s#%s is invalid.",
93 entry->original = src_cond.
get<
Delta>();
96 entry->source = src_cond;
97 entry->target = tar_key.
hash;
99 return *(
used.emplace(tar_key,entry.release()).first);
116 if ( !
used.empty() ) {
117 for (
auto& e :
used ) {
118 e.second->delta.clear();
126 for(
auto& e :
used) {
127 e.second->source.get<
Delta>() = e.second->delta;
128 detail::deletePtr(e.second);
135 std::map<DetElement, Delta> deltas;
139 for (
auto& entry :
used ) {
140 Entry* e = entry.second;
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
Entry(const Entry &c)=delete
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
void destroyHandle(T &handle)
Helper to delete objects from heap and reset the handle.
Condition::key_type set(DetElement det, const Delta &delta)
(1) Add a new entry to an existing DetElement structure.
void clearDeltas()
Clear all delta data in the caches transaction stack.
Condition::key_type hash
Hashed key representation.
GlobalAlignmentStack::StackEntry Entry
Entry & operator=(const Entry &c)=delete
bool isValid() const
Check the validity of the object held by the handle.
virtual bool insert(DetElement detector, Condition::itemkey_type key, Condition condition)=0
Insert a new entry to the map. The detector element key and the item key make a unique global conditi...
Key definition to optimize ans simplyfy the access to conditions entities.
dd4hep::Condition::key_type target
UsedConditions used
Internal work stack of cached deltas.
Class describing an condition to re-adjust an alignment.
DetElement detector(const std::string &path) const
Convenience only: Access detector element by path.
Helper class to store information about alignment calibration items.
Main condition object handle.
Handle class describing a detector element.
ConditionsMap & slice
Reference to the alignment manager object.
static const Condition::itemkey_type alignmentKey
Key value of an alignment condition object "alignment".
Detector & description
Reference to the detector description object.
static const Condition::itemkey_type deltaKey
Key value of a delta condition "alignment_delta".
static const std::string deltaName
Key name of a delta condition "alignment_delta".
std::pair< Condition::key_type, Entry * > _set(DetElement det, const Delta &delta)
Implementation: Add a new entry to the transaction stack.
virtual Condition get(DetElement detector, Condition::itemkey_type key) const =0
Interface to access conditions by hash value. The detector element key and the item key make a unique...
Object encapsulating the result of a computation call to the alignments calculator.
T & bind()
Bind the data of the conditions object to a given format.
dd4hep::DetElement detector
T & get()
Generic getter. Specify the exact type, not a polymorph type.
void clear() noexcept(false)
We clear the entire cached stack of used entries.
unsigned long long int key_type
Forward definition of the key type.
void setFlag(mask_type option)
Flag operations: Set a conditons flag.
virtual ~AlignmentsCalib() noexcept(false)
Default destructor.
The main interface to the dd4hep detector description package.
AlignmentsCalculator::Result commit()
Commit all pending transactions. Returns number of altered entries.
Result compute(const std::map< DetElement, Delta > &deltas, ConditionsMap &alignments) const
Compute all alignment conditions of the internal dependency list.
AlignmentsCalib()=delete
No default constructor.
Namespace for implementation details of the AIDA detector description toolkit.
Alignment calculator instance to handle alignment dependencies.
Out version of the std auto_ptr implementation base either on auto_ptr or unique_ptr.