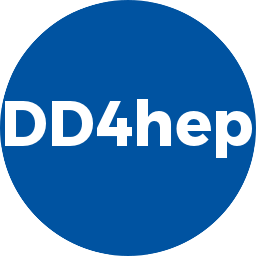 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DD4HEP_DETTYPE_H
15 #define DD4HEP_DETTYPE_H
71 inline void set(
unsigned long prop ) {
76 inline void unset(
unsigned long prop ) {
81 inline bool is(
unsigned long prop )
const {
82 return (
_type & prop ) == prop ;
86 inline bool isNot(
unsigned long prop )
const {
87 return (
_type & prop ) == 0 ;
101 os <<
"DetType( " << std::hex <<
"0x" << t.
_type <<
") : " << std::dec ;
127 #endif // DD4HEP_DETTYPE_H
bool isNot(unsigned long prop) const
true if detector has none of the given properties
bool is(unsigned long prop) const
true if detector has all properties
Helper class for encoding sub detector types in a flag word.
std::ostream & operator<<(std::ostream &os, const DetType &t)
void set(unsigned long prop)
set additional properties
unsigned long to_ulong() const
return the flag word
friend std::ostream & operator<<(std::ostream &os, const DetType &t)
void unset(unsigned long prop)
unset the given properties
DetType(unsigned long types)
DetectorTypeEnumeration
Different detector type flags.
Namespace for the AIDA detector description toolkit.