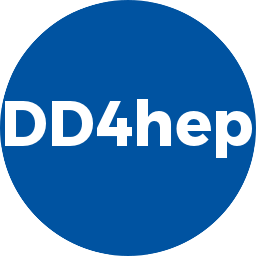 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
27 string det_name = x_det.nameStr();
31 bool useRot = x_det.hasChild(
_U(rotation));
32 bool usePos = x_det.hasChild(
_U(position));
36 sdet.setType(
"compound");
40 pos =
Position(x_det.position().x(), x_det.position().y(), x_det.position().z());
43 rot =
RotationZYX(x_det.rotation().x(), x_det.rotation().y(), x_det.rotation().z());
46 if ( x_det.hasChild(
_U(shape)) ) {
48 string type = x_shape.typeStr();
51 printout(DEBUG,det_name,
"+++ Creating detector assembly with shape of type:%s",type.c_str());
52 vol =
Volume(det_name,solid,mat);
55 printout(DEBUG,det_name,
"+++ Creating detector assembly without shape");
59 vol.
setAttributes(description,x_det.regionStr(),x_det.limitsStr(),x_det.visStr());
63 if( useRot && usePos ){
72 sdet.setPlacement(pv);
75 string nam = component.nameStr();
Class to support the access to collections of XmlNodes (or XmlElements)
#define DECLARE_DETELEMENT(name, func)
Handle class holding a placed volume (also called physical volume)
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
Class to easily access the properties of single XmlElements.
Implementation class extending the ROOT assembly volumes (TGeoVolumeAssembly)
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
dd4hep::xml::Component xml_comp_t
Handle class describing a material.
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
DD4hep internal namespace.
Solid createShape(Detector &description, const std::string &shape_type, xml::Element element)
Create a solid shape using the plugin mechanism from the attributes of the XML element.
dd4hep::xml::DetElement xml_det_t
void setDetectorTypeFlag(dd4hep::xml::Handle_t e, dd4hep::DetElement sdet)
ROOT::Math::Transform3D Transform3D
ROOT::Math::XYZVector Position
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
ROOT::Math::RotationZYX RotationZYX
const Volume & setAttributes(const Detector &description, const std::string ®ion, const std::string &limits, const std::string &vis) const
Attach attributes to the volume.
virtual void declareParent(const std::string &detector_name, const DetElement &det)=0
Register new parent detector using the detector name.
dd4hep::xml::Dimension xml_dim_t