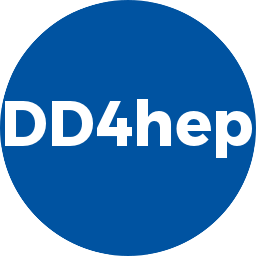 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
16 #include <DDG4/Geant4SensDetAction.inl>
21 #include <G4VSolid.hh>
33 using namespace detail;
68 POSITION_WEIGHTED = 1,
70 POSITION_PREPOINT = 3,
71 POSITION_POSTPOINT = 4
78 G4VPhysicalVolume* thisPV = 0;
79 double distance_to_inside = 0.0;
80 double distance_to_outside = 0.0;
81 double mean_time = 0.0;
82 double step_length = 0.0;
87 int hit_position_type = POSITION_MIDDLE;
90 EInside last_inside = kOutside;
91 long long int cell = 0;
92 bool single_deposit_mode =
false;
99 mean_pos.SetXYZ(0,0,0);
100 distance_to_inside = 0;
101 distance_to_outside = 0;
113 last_inside = kOutside;
119 if( DEBUG == printLevel() ) {
120 std::cout<<
" DEBUG: Geant4TrackerWeightedSD::start(const G4Step* step, const G4StepPoint* point) ...."<<std::endl;
130 sensitive->
mark(step->GetTrack());
132 parent = step->GetTrack()->GetParentID();
133 g4ID = step->GetTrack()->GetTrackID();
143 if( DEBUG == printLevel() ) {
144 std::cout<<
" DEBUG: Geant4TrackerWeightedSD::update(const G4Step* step) ...."<<std::endl;
149 post.
storePoint(step,step->GetPostStepPoint());
153 mean_pos.SetX(mean_pos.x()+mean.x()*post.
truth.
deposit);
154 mean_pos.SetY(mean_pos.y()+mean.y()*post.
truth.
deposit);
155 mean_pos.SetZ(mean_pos.z()+mean.z()*post.
truth.
deposit);
157 step_length += step->GetStepLength();
159 cell = sensitive->
cellID(step);
162 sensitive->
except(
"+++ Invalid CELL ID for hit!");
171 return current > 0 && current != tr->GetTrackID();
177 extractHit(collection, ended);
182 double dist = solid->DistanceToOut(G4ThreeVector(p.X(),p.Y(),p.Z()),
183 G4ThreeVector(
v.X(),
v.Y(),
v.Z()));
184 distance_to_outside =
dist;
190 double dist = solid->DistanceToOut(G4ThreeVector(p.X(),p.Y(),p.Z()),
191 G4ThreeVector(
v.X(),
v.Y(),
v.Z()));
192 distance_to_inside =
dist;
197 if( DEBUG == printLevel() ) {
198 std::cout<<
" DEBUG: Geant4TrackerWeightedSD::extractHit(Geant4HitCollection* collection, EInside ended) ...."<<std::endl;
199 std::cout<<
" DEBUG: =================================================="<<std::endl;
203 if ( current != -1 ) {
206 double time = deposit != 0 ? mean_time / deposit : mean_time;
207 char dist_in[64], dist_out[64];
209 switch(hit_position_type) {
210 case POSITION_WEIGHTED:
211 pos = deposit != 0 ? mean_pos / deposit : mean_pos;
213 case POSITION_PREPOINT:
216 case POSITION_POSTPOINT:
219 case POSITION_MIDDLE:
227 else if ( ended == kInside )
229 else if ( ended == kOutside )
234 deposit,time, step_length,
236 hit->
flag = hit_flag;
240 dist_in[0] = dist_out[0] = 0;
242 ::snprintf(dist_in,
sizeof(dist_in),
" [%.2e um]",distance_to_inside/CLHEP::um);
244 ::snprintf(dist_out,
sizeof(dist_out),
" [%.2e um]",distance_to_outside/CLHEP::um);
245 sensitive->
print(
"+++ G4Track:%5d CREATE hit[%03d]:%3d deps E:"
246 " %.2e keV Pos:%7.2f %7.2f %7.2f [mm] Start:%s%s%s%s End:%s%s%s%s",
249 pos.X()/CLHEP::mm,pos.Y()/CLHEP::mm,pos.Z()/CLHEP::mm,
258 collection->
add(hit);
264 G4bool
process(
const G4Step* step, G4TouchableHistory* ) {
266 if( DEBUG == printLevel() ) {
267 std::cout<<
" DEBUG: Geant4TrackerWeightedSD::process(const G4Step* step, G4TouchableHistory* ) ...."<<std::endl;
274 G4VSolid* preSolid = h.
solid(h.
pre);
278 EInside pre_inside = preSolid->Inside(local_pre);
279 EInside post_inside = postSolid->Inside(local_post);
283 const void* postSD = h.
postSD();
284 const void* preSD = h.
preSD();
285 G4VSolid* solid = (preSD == thisSD) ? preSolid : postSolid;
287 if ( current == h.
trkID() && thisPV != 0 && prePV != thisPV ) {
288 if( DEBUG == printLevel() ) {
289 std::cout<<
" DEBUG: Geant4TrackerWeightedSD: if ( current == h.trkID() && thisPV != 0 && prePV != thisPV ),"
290 <<
" Track went into new Volume, extracted the hit in prePV, then start a new hit in thisPV."
293 extractHit(post_inside);
299 update(step).calc_dist_out(solid).extractHit(post_inside);
303 else if ( current == h.
trkID() && postSD != thisSD ) {
304 update(step).calc_dist_out(solid).extractHit(kOutside);
308 else if ( current == h.
trkID() && postSD == thisSD && post_inside == kSurface ) {
309 update(step).calc_dist_out(solid).extractHit(kSurface);
313 else if ( current == h.
trkID() && postSD == thisSD && post_inside == kOutside ) {
314 update(step).calc_dist_out(solid).extractHit(post_inside);
318 else if ( current == h.
trkID() && postSD == thisSD && post_inside == kInside ) {
319 last_inside = post_inside;
320 update(step).calc_dist_out(solid);
325 else if ( current != h.
trkID() && current >= 0 ) {
326 extractHit(last_inside);
331 EInside inside = pre_inside;
333 if ( preSD != thisSD ) {
335 inside = post_inside;
336 sensitive->
print(
"++++++++++ Using POST step volume to start hit -- dubious ?");
342 if ( inside == kSurface )
344 else if ( inside == kInside )
346 else if ( inside == kOutside )
350 if ( inside == kInside ) {
356 last_inside = post_inside;
358 calc_dist_out(solid);
363 extractHit(post_inside);
366 else if ( post_inside == kSurface ) {
367 extractHit(post_inside);
370 else if ( thisSD == preSD && (preSD != postSD || prePV != postPV) ) {
371 extractHit(post_inside);
374 else if ( thisSD == postSD && (preSD != postSD || prePV != postPV) ) {
375 sensitive->
error(
"+++++ WRONG!!! Extract. How did we get here?");
376 extractHit(post_inside);
379 else if ( single_deposit_mode ) {
380 extractHit(post_inside);
394 sensitive->
print(
"++++++++++ Found dangling hit: Is the hit extraction logic correct?");
395 extractHit(coll,last_inside);
405 std::stringstream str;
406 str <<
" ----- step in detector " << h.
sdName( s->GetPreStepPoint() )
407 <<
" prePos " << h.
prePos()
411 <<
" preVolume " << h.
volName( s->GetPreStepPoint() )
412 <<
" postVolume " << h.
volName( s->GetPostStepPoint() )
414 <<
" momentum : " << std::scientific
415 << s->GetPreStepPoint()->GetMomentum()[0] <<
", "
416 << s->GetPreStepPoint()->GetMomentum()[1]<<
", "
417 << s->GetPreStepPoint()->GetMomentum()[2]
419 << s->GetPostStepPoint()->GetMomentum()[0] <<
", "
420 << s->GetPostStepPoint()->GetMomentum()[1] <<
", "
421 << s->GetPostStepPoint()->GetMomentum()[2]
422 <<
", PDG: " << s->GetTrack()->GetDefinition()->GetPDGEncoding();
423 std::cout << str.str() << std::endl;
428 sensitive->
except(
"GFlash/FastSim action is not implemented for SD: %s", sensitive->
c_name());
435 declareProperty(
"HitPositionCombination", m_userData.hit_position_type);
436 declareProperty(
"CollectSingleDeposits", m_userData.single_deposit_mode);
437 m_userData.e_cut = m_sensitive.energyCutoff();
438 m_userData.sensitive =
this;
443 m_userData.startEvent();
448 m_userData.endEvent();
453 m_collectionID = declareReadoutFilteredCollection<Geant4Tracker::Hit>();
464 return m_userData.process(step, history);
470 return m_userData.process(spot, history);
virtual void begin(G4HCofThisEvent *hce) final
G4VSensitiveDetector interface: Method invoked at the begining of each event.
#define DECLARE_GEANT4SENSITIVE(name)
Direction momentum
Hit direction.
Position position
Hit position.
G4bool process(const Geant4FastSimSpot *, G4TouchableHistory *)
GFLash processing callback.
virtual void defineCollections()
Define collections created by this sensitivie action object.
long flag
User flag to classify hits.
virtual size_t GetSize() const override
Access the collection size.
Position prePos() const
Returns the pre-step position.
int pdgID
Particle ID from the PDG table.
Geant4ActionSD & detector() const
Access to the sensitive detector object.
TrackerWeighted & calc_dist_out(const G4VSolid *solid)
G4ThreeVector globalToLocalG4(double x, double y, double z) const
Coordinate transformation to local coordinates.
const char * volName(const G4StepPoint *p, const char *undefined="") const
virtual void initialize() final
Initialization overload for specialization.
Sensitive detector meant for tracking detectors with multiple ways to combine steps.
Generic hit container class using Geant4HitWrapper objects.
void copyFrom(const Hit &c)
Explicit assignment operation.
void endEvent()
Post-event action callback.
long long int cellID
cellID
int trkID() const
Access the G4 track ID.
const G4ThreeVector & prePosG4() const
Returns the pre-step position as a G4ThreeVector.
Spot definition for fast simulation and GFlash.
long long int volumeID(const G4Step *step)
Returns the volumeID of the sensitive volume corresponding to the step.
virtual void end(G4HCofThisEvent *hce) final
G4VSensitiveDetector interface: Method invoked at the end of each event.
Geant4HitCollection * collection(std::size_t which)
Retrieve the hits collection associated with this detector by its serial number.
virtual bool process(const G4Step *step, G4TouchableHistory *history) final
G4VSensitiveDetector interface: Method for generating hit(s) using the G4Step object.
G4VPhysicalVolume * preVolume() const
void extractHit(Geant4HitCollection *collection, EInside ended)
const char * postStepStatus() const
Returns the post-step status in form of a string.
G4bool process(const G4Step *step, G4TouchableHistory *)
Method for generating hit(s) using the information of G4Step object.
Helper class to ease the extraction of information from a G4Step object.
virtual void clear(G4HCofThisEvent *hce) final
G4VSensitiveDetector interface: Method invoked if the event was aborted.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
void mark(const G4Track *track) const
Mark the track to be kept for MC truth propagation during hit processing.
void error(const char *fmt,...) const
Support of error messages.
DDG4 tracker hit class used by the generic DDG4 tracker sensitive detector.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
long g4ID
Original Geant 4 track identifier of the creating track (debugging)
void extractHit(EInside ended)
Extract hit information and add the created hit to the collection.
bool mustSaveTrack(const G4Track *tr) const
Helper function to decide if the hit has to be extracted and saved in the collection.
double deposit
Total energy deposit in this hit.
TrackerWeighted & update(const G4Step *step)
Update energy and track information during hit info accumulation.
G4VSolid * solid(const G4StepPoint *p) const
Template class to ease the construction of sensitive detectors using particle template specialization...
G4VPhysicalVolume * postVolume() const
Contribution truth
Monte Carlo / Geant4 information.
void startEvent()
Pre event action callback.
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
const G4ThreeVector & postPosG4() const
Returns the post-step position as a G4ThreeVector.
int trackID
Geant 4 Track identifier.
Position postPos() const
Returns the post-step position.
ROOT::Math::XYZVector Position
TrackerWeighted & calc_dist_in(const G4VSolid *solid)
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
G4VSensitiveDetector * preSD() const
const char * c_name() const
Access name of the action.
double dist(const Position &p0, const Position &p1)
TrackerWeighted & start(const G4Step *step, const G4StepPoint *point)
Start a new hit.
long long int cellID(const G4Step *step)
Returns the cellID of the sensitive volume corresponding to the step.
TrackerWeighted & clear()
Clear collected information and restart for new hit.
TrackerWeighted()=default
Default constructor.
Namespace for the AIDA detector description toolkit.
Hit & storePoint(const G4Step *step, const G4StepPoint *point)
Store Geant4 point and step information into tracker hit structure.
std::string sdName(const G4StepPoint *p, const std::string &undefined="") const
void add(TYPE *hit_pointer)
Add a new hit with a check, that the hit is of the same type.
The base class for Geant4 sensitive detector actions implemented by users.
G4VSensitiveDetector * postSD() const
Geant4SensitiveAction< TrackerWeighted > Geant4TrackerWeightedAction
const char * preStepStatus() const
Returns the pre-step status in form of a string.
Geant4 sensitive detector combining all deposits of one G4Track within one sensitive element.
Hit & clear()
Clear hit content.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history) final
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
double time
Timestamp when this energy was deposited.
void dumpStep(const Geant4StepHandler &h, const G4Step *s)
dumpStep