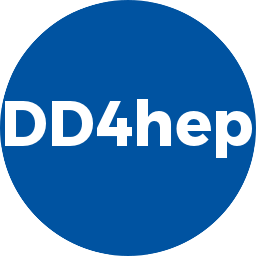 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <CLHEP/Units/SystemOfUnits.h>
24 #include <G4ParticleTable.hh>
25 #include <G4ParticleDefinition.hh>
87 warning(
"printInteraction: Bad primary event [NULL-Pointer].");
92 warning(
"printInteraction: Bad interaction identifier 0x%08X [Unknown Mask].",mask);
102 warning(
"printInteraction: Invalid interaction pointer [NULL-Pointer].");
105 for(
const auto& iv : inter->
vertices ) {
107 print(
"+-> Interaction [%d] [%.3f , %.3f] GeV %s pos:(%.3f %.3f %.3f)[mm]",
109 v->x/CLHEP::mm,
v->y/CLHEP::mm,
v->z/CLHEP::mm);
111 for (
int i :
v->out ) {
124 G4ParticleTable* particleTable = G4ParticleTable::GetParticleTable();
137 ROOT::Math::XYZVector unit_direction, position =
m_position;
141 vtx->
x = position.X();
142 vtx->
y = position.Y();
143 vtx->
z = position.Z();
144 inter->vertices[
m_mask].emplace_back( vtx );
146 double momentum = 0.0;
148 Particle* p =
new Particle();
150 unit_direction = direction.unit();
151 p->id = inter->nextPID();
156 p->psx = unit_direction.X()*momentum;
157 p->psy = unit_direction.Y()*momentum;
158 p->psz = unit_direction.Z()*momentum;
173 inter->particles.emplace(p->id,p);
174 vtx->
out.insert(p->id);
175 printout(INFO,
name(),
"Particle [%d] %-12s Mom:%.3f GeV vertex:(%6.3f %6.3f %6.3f)[mm] direction:(%6.3f %6.3f %6.3f)",
177 vtx->
x/CLHEP::mm, vtx->
y/CLHEP::mm, vtx->
z/CLHEP::mm,
178 direction.X(), direction.Y(), direction.Z());
int m_multiplicity
Property: Desired multiplicity of the particles to be shot.
virtual ~Geant4ParticleGenerator()
Default destructor.
Class modelling a complete primary event with multiple interactions.
bool m_needsControl
Default property: Flag to create control instance.
double rndm(int i=0)
Create flat distributed random numbers in the interval ]0,1].
int m_mask
Property: User mask passed to all particles in the generated interaction.
void dumpWithVertex(int level, const std::string &src, const char *tag) const
Output type 3:+++ "tag" ID: 0 e- status:00000014 type: 11 Vertex:(+0.00e+00,+0.00e+00,...
Geant4ParticleGenerator(Geant4Context *context, const std::string &name)
Standard constructor.
Class modelling a single interaction with multiple primary vertices and particles.
int mask
Vertex mask to associate particles from collision.
VertexMap vertices
The map of primary vertices for the particles.
double m_momentumMin
Property: Minimal momentum value.
static void increment(T *)
Increment count according to type information.
virtual void getParticleMultiplicity(int &multiplicity) const
Particle modification. Caller presets defaults to: (multiplicity=m_multiplicity)
ParticleMap particles
The map of particles participating in this primary interaction.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
Geant4Random & random() const
Access the random number generator.
void warning(const char *fmt,...) const
Support of warning messages.
double m_energy
Property: Fixed momentum value, overwrites momentumMin and momentumMax if set.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
G4ParticleDefinition * m_particle
Pointer to geant4 particle definition.
ROOT::Math::XYZVector m_direction
Property: Shooting direction of the gun.
virtual void getVertexPosition(ROOT::Math::XYZVector &position) const
Particle's vertex modification. Caller presets defaults to: (multiplicity=m_multiplicity)
void getParticleMomentumUniform(double &momentum) const
Uniform particle momentum.
virtual void operator()(G4Event *event) override
Callback to generate primary particles.
ROOT::Math::XYZVector m_position
Property: Position of the gun in space.
double m_momentumMax
Property: Maximal momentum value.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
Concrete implementation of the Geant4 generator action base class.
Mini interface to THE random generator of the application.
const std::string & name() const
Access name of the action.
PrintLevel outputLevel() const
Access the output level.
Particles out
The list of outgoing particles.
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
std::string m_particleName
Property: Particle name.
Data structure to access derived MC particle information.
Data structure to store the MC particle information.
Data structure to store the MC vertex information.
virtual void getParticleDirection(int num, ROOT::Math::XYZVector &direction, double &momentum) const
Particle modification. Caller presets defaults to: ( direction = m_direction, momentum = m_energy)
virtual void printInteraction(int mask) const
Print single particle interaction identified by its mask.
User event context for DDG4.
void add(int id, Geant4PrimaryInteraction *interaction)
Add a new interaction object to the event.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4PrimaryInteraction * get(int id) const
Retrieve an interaction by its ID.