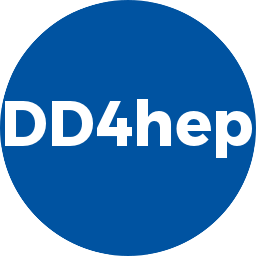 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 static const BasicGrammar* s_any_grammar = &BasicGrammar::instance<std::any>();
34 if ( hash_key != 0 && hash_key != ~0x0ULL ) {
41 except(
"ConditionAny",
"+++ Cannot create a any-condition with an invalid key: %016llX",hash_key);
56 except(
"ConditionAny",
57 "+++ Cannot assign unbound conditions data to handle. [Invalid operation]");
60 except(
"ConditionAny",
61 "+++ Cannot assign data of type " +
63 " to handle holding std::any. [Invalid operation]");
118 return *(std::any*)o.
ptr();
120 throw std::bad_cast();
127 return *(std::any*)o.
ptr();
129 throw std::bad_cast();
134 return this->
get().has_value();
141 const std::any* a = (
const std::any*)o->
data.
ptr();
151 const std::any* a = (
const std::any*)o->
data.
ptr();
152 return typeName(a->type());
154 return typeName(
typeid(
void));
std::any & get()
Generic getter. Specify the exact type, not a polymorph type.
Condition::key_type hash
Hash value of the name.
detail::ConditionObject Object
Extern accessible definition of the contained element type.
void use_data(detail::ConditionObject *obj)
Verify that underlying data are either invalid of contain an instance of std::any.
Condition::mask_type flags
Flags.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Condition::detkey_type detkey_type
High part of the key identifies the detector element.
mask_type flags() const
Flag operations: Get condition flags.
bool testFlag(mask_type option) const
Flag operations: Test for a given a conditons flag.
void setFlag(mask_type option)
Flag operations: Set a conditons flag.
Helper union to interprete conditions keys.
itemkey_type item_key() const
Item part of the identifier.
Class describing the interval of validty type.
const std::string & type_name() const
Access to the type information name.
void unFlag(Condition::mask_type option)
Flag operations: UN-Set a conditons flag.
void * bind(const BasicGrammar *grammar)
Bind data value.
Class describing the interval of validty.
Base class describing string evaluation to C++ objects using boost::spirit.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
const IOVType * iovType() const
Access safely the IOV-type.
key_type key() const
Hash identifier.
Class describing an opaque data block.
detkey_type detector_key() const
DetElement part of the identifier.
void unFlag(mask_type option)
Flag operations: UN-Set a conditons flag.
void * ptr() const
Write access to the data buffer. Is only valid after call to bind<T>()
detail::ConditionObject * m_element
Single and only data member: Reference to the actual element.
const void * ptr() const
Access to the data buffer (read only!). Is only valid after call to bind<T>()
void setFlag(Condition::mask_type option)
Flag operations: Set a conditons flag.
const IOV * iovData() const
Access safely the IOV.
const std::string any_type_name() const
Access to the type information as string.
const IOV & iov() const
Access the IOV block.
The data class behind a conditions handle.
detail::ConditionObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
const std::type_info & any_type() const
Access to the type information.
Condition::mask_type mask_type
Forward definition of the object properties.
detail::ConditionObject * ptr() const
Access to the held object.
Condition::detkey_type det_key
Namespace for the AIDA detector description toolkit.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
OpaqueDataBlock data
Data block.
const BasicGrammar * grammar
Data type.
bool has_value() const
Checks whether the object contains a value.
Condition::key_type key_type
Forward definition of the key type.
const IOVType & iovType() const
Access the IOV type.
ConditionAny()=default
Default constructor.
struct dd4hep::ConditionKey::KeyMaker::@2 values
bool testFlag(Condition::mask_type option) const
Flag operations: Test for a given a conditons flag.
Condition::itemkey_type itemkey_type
Low part of the key identifies the item identifier.
Condition::itemkey_type item_key