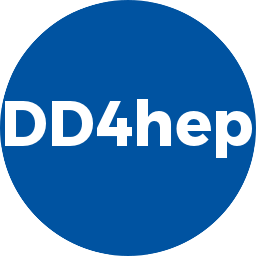 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_CONDITIONANY_H
14 #define DD4HEP_CONDITIONANY_H
27 class ConditionObject;
80 template <
typename PAYLOAD>
ConditionAny(
const std::string&
name,
const std::string& type, PAYLOAD&&
data);
121 const std::any&
get()
const;
126 const std::type_info&
any_type()
const;
130 template <
typename T> T&
as();
132 template <
typename T>
const T&
as()
const;
134 template <
typename T> T
value();
136 template <
typename T> T*
pointer();
138 template <
typename T>
const T*
pointer()
const;
152 template <
typename PAYLOAD>
inline
155 c.
get() = std::move(payload);
160 template <
typename PAYLOAD>
inline
163 c.
get() = std::move(payload);
193 return std::any_cast<T>(this->
get());
198 T* ptr_payload = std::any_cast<T>(&this->
get());
199 if ( ptr_payload )
return *ptr_payload;
200 throw std::runtime_error(
"ConditionAny: Cannot access value of std::any as a reference to "+typeName(
typeid(T)));
205 const T* ptr_payload = std::any_cast<T>(&this->
get());
206 if ( ptr_payload )
return *ptr_payload;
207 throw std::runtime_error(
"ConditionAny: Cannot access value of std::any as a reference to "+typeName(
typeid(T)));
212 return isValid() ? std::any_cast<T>(&this->
get()) :
nullptr;
217 return isValid() ? std::any_cast<const T>(&this->
get()) :
nullptr;
221 #endif // DD4HEP_CONDITIONANY_H
std::any & get()
Generic getter. Specify the exact type, not a polymorph type.
ConditionAny & operator=(ConditionAny &&c)=default
Assignment move operator.
detail::ConditionObject Object
Extern accessible definition of the contained element type.
void use_data(detail::ConditionObject *obj)
Verify that underlying data are either invalid of contain an instance of std::any.
bool isValid() const
Check the validity of the object held by the handle.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Condition::detkey_type detkey_type
High part of the key identifies the detector element.
Main condition object handle.
mask_type flags() const
Flag operations: Get condition flags.
bool testFlag(mask_type option) const
Flag operations: Test for a given a conditons flag.
void setFlag(mask_type option)
Flag operations: Set a conditons flag.
const char * name() const
Access the object name (or "" if not supported by the object)
itemkey_type item_key() const
Item part of the identifier.
Class describing the interval of validty type.
unsigned int detkey_type
High part of the key identifies the detector element.
Main condition object handle.
Class describing the interval of validty.
T & as()
Access the contained object inside std::any.
Q * data() const
Access to an unrelated object type.
T value()
Access a copy of the contained object inside std::any.
key_type key() const
Hash identifier.
detkey_type detector_key() const
DetElement part of the identifier.
void unFlag(mask_type option)
Flag operations: UN-Set a conditons flag.
detail::ConditionObject * m_element
Single and only data member: Reference to the actual element.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
unsigned long long int key_type
Forward definition of the key type.
unsigned int mask_type
Forward definition of the object properties.
ConditionAny(const Handle< Q > &e)
Constructor from another handle.
const std::string any_type_name() const
Access to the type information as string.
const IOV & iov() const
Access the IOV block.
The data class behind a conditions handle.
ConditionAny(ConditionAny &&c)=default
Move constructor.
const std::type_info & any_type() const
Access to the type information.
Condition::mask_type mask_type
Forward definition of the object properties.
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
ConditionAny & operator=(const ConditionAny &c)=default
Assignment copy operator.
T * pointer()
Access the contained object inside std::any.
bool has_value() const
Checks whether the object contains a value.
Condition::key_type key_type
Forward definition of the key type.
const IOVType & iovType() const
Access the IOV type.
ConditionAny & operator=(Handle< Q > &&c)
Assignment move operator.
ConditionAny(const ConditionAny &c)=default
Copy constructor.
ConditionAny()=default
Default constructor.
Condition::itemkey_type itemkey_type
Low part of the key identifies the item identifier.
ConditionAny(Object *p)
Initializing constructor.