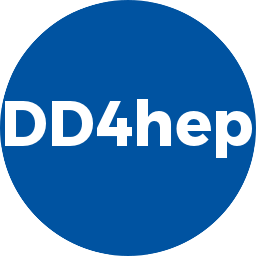 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
36 PrintLevel s_print = DEBUG;
41 bool old = s_print==ALWAYS;
42 s_print = value ? ALWAYS : DEBUG;
49 rot.SetComponents(r.z(), r.y(), r.x());
50 printout(s_print,
"<rotation>",
51 " Rotation: x=%9.3f y=%9.3f z=%9.3f phi=%7.4f psi=%7.4f theta=%7.4f",
52 r.x(), r.y(), r.z(), rot.Phi(), rot.Psi(), rot.Theta());
58 pos.SetXYZ(p.x(), p.y(), p.z());
59 printout(s_print,
"<position>",
" Position: x=%9.3f y=%9.3f z=%9.3f",
60 pos.X(), pos.Y(), pos.Z());
67 tr.SetXYZ(x=p.x(), y=p.y(), z=p.z());
68 printout(s_print,
"<translation>",
" Pivot: x=%9.3f y=%9.3f z=%9.3f",x,y,z);
76 xml_h child_rot, child_pos, child_piv;
78 if ( (child_pos=e.
child(
_U(position),
false)) )
80 if ( (child_rot=e.
child(
_U(rotation),
false)) ) {
82 if ( (child_piv=e.
child(
_U(pivot),
false)) )
85 if ( child_rot && child_pos && child_piv )
87 else if ( child_rot && child_pos )
89 else if ( child_rot && child_piv )
105 string val_type = e.
attr<
string>(
_U(value));
106 string key_type = e.
attr<
string>(
_U(
key));
112 if ( i.hasAttr(
_U(
key)) && i.hasAttr(
_U(value)) ) {
113 string key = i.attr<
string>(
_U(
key));
114 string val = i.attr<
string>(
_U(value));
126 string typ = elt.typeStr();
127 string val = elt.hasAttr(
_U(value)) ? elt.valueStr() : elt.text();
130 "++ Failed to convert unknown sequence conditions type: %s",typ.c_str());
Class to support the access to collections of XmlNodes (or XmlElements)
static bool bind_sequence(OBJECT &object, const std::string &typ, const std::string &val)
Binding function for sequences (unmapped STL containers)
void parse_sequence(Handle_t e, OpaqueDataBlock &block)
Converts linear STL containers from their string representation.
Class describing an opaque conditions data block.
Class to easily access the properties of single XmlElements.
void parse_delta(Handle_t e, OpaqueDataBlock &block)
Parse delta into an opaque data block.
Class describing an condition to re-adjust an alignment.
void parse(Handle_t e, RotationZYX &rot)
Convert rotation XML objects to dd4hep::RotationZYX.
void * bind(const BasicGrammar *grammar)
Bind data value.
bool setXMLParserDebug(bool new_value)
Set debug print level for this module. Default is OFF.
ROOT::Math::Translation3D Translation3D
dd4hep::xml::Collection_t xml_coll_t
Handle_t child(const XmlChar *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
static bool insert_map(const BINDER &b, OBJECT &o, const std::string &key_type, const std::string &key, const std::string &val_type, const std::string &val)
Filling function for STL maps.
ROOT::Math::XYZVector Position
dd4hep::xml::Dimension xml_dim_t
Namespace for the AIDA detector description toolkit.
void parse_mapping(Handle_t e, OpaqueDataBlock &block)
Converts opaque maps to OpaqueDataBlock objects.
static bool bind_map(const BINDER &b, OBJECT &o, const std::string &key_type, const std::string &val_type)
Binding function for STL maps. Does not fill data!
ROOT::Math::RotationZYX RotationZYX
dd4hep::xml::Handle_t xml_h
Helper class to bind STL map objects.
T attr(const Attribute a) const
Access typed attribute value by the XmlAttr.
dd4hep::xml::Dimension xml_dim_t