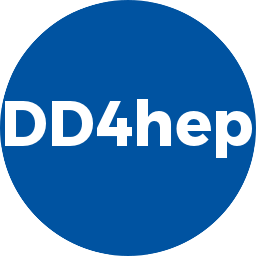 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DDCORE_VISMATERIALPROCESSOR_H
14 #define DD4HEP_DDCORE_VISMATERIALPROCESSOR_H
55 #endif // DD4HEP_DDCORE_VISMATERIALPROCESSOR_H
111 printout(ALWAYS,
name,
"++ SETUP Active element: %-11s Vis: %s",atom.name(),
activeVis.
name());
114 printout(ALWAYS,
name,
"++ SETUP Active material: %-11s Vis: %s", mat.name(),
activeVis.
name());
119 printout(ALWAYS,
name,
"++ SETUP Inactive material: %-11s Vis: %s",
"All-non-active",
inactiveVis.
name());
122 printout(ALWAYS,
name,
"++ SETUP Inactive material: %-11s Vis: %s", mat.name(),
activeVis.
name());
131 double frac_active = 0.0;
139 "++ Volume:%s [%s] active:%s fraction:%.3f active-vis:%s inactive-vis:%s",
183 static void* create_object(
Detector& description,
int argc,
char** argv) {
186 for (
int i=0; i<argc; ++i ) {
188 if ( ::strncmp(argv[i],
"-vis-active",6) == 0 ) {
193 else if ( ::strncmp(argv[i],
"-vis-inactive",6) == 0 ) {
198 else if ( ::strncmp(argv[i],
"-elt-active",6) == 0 ) {
199 Atom atom = helper.element(argv[++i]);
203 else if ( ::strncmp(argv[i],
"-mat-active",6) == 0 ) {
204 Material mat = helper.material(argv[++i]);
208 else if ( ::strncmp(argv[i],
"-mat-inactive",6) == 0 ) {
209 Material mat = helper.material(argv[++i]);
213 else if ( ::strncmp(argv[i],
"-all-inactive",6) == 0 ) {
217 else if ( ::strncmp(argv[i],
"-fraction",3) == 0 ) {
218 std::stringstream str(argv[++i]);
221 if ( !str.fail() )
continue;
224 else if ( ::strncmp(argv[i],
"-path",4) == 0 ) {
225 std::string path = argv[++i];
228 printout(ERROR,
"VisMaterialProcessor",
"++ Invalid DetElement path: %s",path.c_str());
230 else if ( ::strncmp(argv[i],
"-name",4) == 0 ) {
231 proc->
name = argv[++i];
234 else if ( ::strncmp(argv[i],
"-show",4) == 0 ) {
239 "Usage: DD4hep_VisMaterialProcessor -arg [-arg] \n"
240 " -vis-active <name> Set the visualization attribute for active materials\n"
241 " -vis-inactive <name> Set the visualization attribute for inactive materials\n"
242 " -elt-active <name> Add active element by name. If the fractional sum of \n"
243 " all active elements in a volume exceeds <fraction> \n"
244 " the volume is considered active \n"
245 " -mat-active <name> Add material by name to the list of active materials\n"
246 " -mat-inactive <name> Add material by name to the list of inactive materials\n"
247 " -all-inactive Auto set all volumes inactive, which are NOT active \n"
248 " -fraction <double> Set the fraction above which the active elment content\n"
249 " defines an active volume. \n"
250 " -show Print setup to output device (stdout) \n"
251 "\tArguments given: " << arguments(argc,argv) << std::endl << std::flush;
257 return (
void*)placement_proc;
Generic PlacedVolume processor.
std::vector< Material > inactiveMaterials
Handle class describing an element in the periodic table.
virtual ~VisMaterialProcessor()
Default destructor.
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
bool isValid() const
Check the validity of the object held by the handle.
const char * name() const
Access the object name (or "" if not supported by the object)
std::vector< Atom > activeElements
double fraction(Atom atom) const
Access the fraction of an element within the material.
Material material() const
Access to the Volume material.
Handle class describing a material.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
void _show()
Print properties.
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
std::vector< Material > activeMaterials
DD4hep DetElement creator for the CMS geometry.
DetectorHelper: class to shortcut certain questions to the dd4hep detector description interface.
virtual const HandleMap & visAttributes() const =0
Accessor to the map of visualisation attributes.
virtual int operator()(PlacedVolume pv, int level)
Callback to output PlacedVolume information of an single Placement.
T * ptr() const
Access to the held object.
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Namespace for the AIDA detector description toolkit.
Volume volume() const
Logical volume of this placement.
The main interface to the dd4hep detector description package.
VisAttr visAttributes() const
Access the visualisation attributes.
VisMaterialProcessor(Detector &desc)
Initializing constructor.