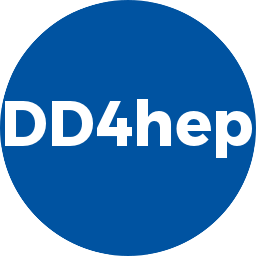 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DDCORE_VISDENSITYPROCESSOR_H
14 #define DD4HEP_DDCORE_VISDENSITYPROCESSOR_H
48 #endif // DD4HEP_DDCORE_VISDENSITYPROCESSOR_H
88 "++ SETUP Minimal material density: %.4f [g/cm3] Vis: %s",
106 static void* create_object(
Detector& description,
int argc,
char** argv) {
109 for (
int i=0; i<argc; ++i ) {
111 if ( ::strncmp(argv[i],
"-vis",6) == 0 ) {
116 else if ( ::strncmp(argv[i],
"-min-vis",6) == 0 ) {
121 else if ( ::strncmp(argv[i],
"-min-density",6) == 0 ) {
126 else if ( ::strncmp(argv[i],
"-name",4) == 0 ) {
127 std::string name = argv[++i];
128 proc->
name = std::move(name);
131 else if ( ::strncmp(argv[i],
"-show",4) == 0 ) {
136 "Usage: DD4hep_VisDensityProcessor -arg [-arg] \n"
137 " -vis <name> Set the visualization attribute for inactive materials\n"
138 " -min-vis <name> Set the visualization attribute for inactive materials\n"
139 " -min-density <number> Minimal density to show the volume. \n"
140 " -show Print setup to output device (stdout) \n"
141 "\tArguments given: " << arguments(argc,argv) << std::endl << std::flush;
147 return (
void*)placement_proc;
Generic PlacedVolume processor.
DD4hep DetElement creator for the CMS geometry.
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
bool isValid() const
Check the validity of the object held by the handle.
const char * name() const
Access the object name (or "" if not supported by the object)
Material material() const
Access to the Volume material.
double density() const
density of the underlying material
Handle class describing a material.
Handle class holding a placed volume (also called physical volume)
virtual ~VisDensityProcessor()
Default destructor.
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
DetectorHelper: class to shortcut certain questions to the dd4hep detector description interface.
void _show()
Print properties.
virtual const HandleMap & visAttributes() const =0
Accessor to the map of visualisation attributes.
double _toDouble(const std::string &value)
String conversions: string to double value.
T * ptr() const
Access to the held object.
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Namespace for the AIDA detector description toolkit.
VisDensityProcessor(Detector &desc)
Initializing constructor.
Volume volume() const
Logical volume of this placement.
The main interface to the dd4hep detector description package.
VisAttr visAttributes() const
Access the visualisation attributes.
virtual int operator()(PlacedVolume pv, int level)
Callback to output PlacedVolume information of an single Placement.