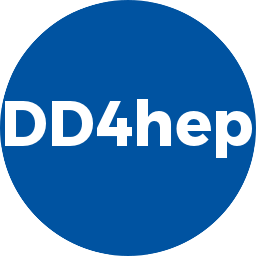 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
16 #include <IMPL/LCCollectionVec.h>
17 #include <IMPL/SimTrackerHitImpl.h>
18 #include <IMPL/SimCalorimeterHitImpl.h>
19 #include <IMPL/MCParticleImpl.h>
20 #include <UTIL/Operators.h>
21 #include <UTIL/ILDConf.h>
58 declareProperty(
"detailedHitsStoring", _detailedHitsStoring ) ;
69 virtual void begin(G4HCofThisEvent* hce)
override {
73 virtual void end(G4HCofThisEvent* hce)
override {
77 virtual bool process(
const G4Step* step, G4TouchableHistory* history)
override {
78 return Base::process(step,history);
82 return Base::processFastSim(spot,history);
85 virtual void clear(G4HCofThisEvent* hce)
override {
105 m_collectionID = Base::defineCollection<lcio::SimTrackerHitImpl>(m_sensitive.readout().name());
114 Position direction = postPos - prePos;
116 double hit_len = direction.R();
120 direction *= new_len/hit_len;
123 lcio::SimTrackerHitImpl* hit =
new lcio::SimTrackerHitImpl;
132 hit->setCellID0( cell & 0xffffffff ) ;
133 hit->setCellID1( ( cell >> 32 ) & 0xffffffff ) ;
135 printout(INFO,
"LcioTestTracker",
"%s> Add hit with deposit:%f Pos:%f %f %f - cellID0: 0x%x cellID1: 0x%x",
136 c_name(),step->GetTotalEnergyDeposit(),position.X(),position.Y(),position.Z() , hit->getCellID0() ,hit->getCellID1() );
138 double pos[3] = {position.x(), position.y(), position.z()};
139 hit->setPosition( pos ) ;
145 collection(m_collectionID)->add(hit);
152 G4TouchableHistory* )
154 except(
"Not implemented");
size_t m_collectionID
Collection identifiers.
virtual void clear(G4HCofThisEvent *hce) override
G4VSensitiveDetector interface: Method invoked if the event was aborted.
virtual void defineCollections()
Define collections created by this sensitivie action object.
virtual bool process(const G4Step *step, G4TouchableHistory *history) override
G4VSensitiveDetector interface: Method for generating hit(s) using the G4Step object.
Position prePos() const
Returns the pre-step position.
virtual void defineCollections() override
Define collections created by this sensitivie action object.
Geant4SensitiveAction< LcioTestTracker > LcioTestTrackerAction
Geant4SensitiveAction(Geant4Context *ctxt, const std::string &nam, DetElement det, Detector &description_ref)
bool _detailedHitsStoring
virtual void end(G4HCofThisEvent *hce) override
G4VSensitiveDetector interface: Method invoked at the end of each event.
static void increment(T *)
Increment count according to type information.
Spot definition for fast simulation and GFlash.
Deprecated: Simple SensitiveAction class ...
virtual bool process(const G4Step *step, G4TouchableHistory *history) final
G4VSensitiveDetector interface: Method for generating hit(s) using the G4Step object.
Helper class to ease the extraction of information from a G4Step object.
Handle class describing a detector element.
static void decrement(T *)
Decrement count according to type information.
virtual ~Geant4SensitiveAction()
Default destructor.
Deprecated: Simple SensitiveAction class ...
Position postPos() const
Returns the post-step position.
ROOT::Math::XYZVector Position
dd4hep::DDSegmentation::VolumeID VolumeID
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history) override
GFlash/FastSim interface: Method for generating hit(s) using the G4Step object.
#define DECLARE_GEANT4SENSITIVE_NS(name_space, name)
Namespace for the AIDA detector description toolkit.
double mean_length(const dd4hep::Position &p1, const dd4hep::Position &p2)
Calculate the mean length of two vectors.
dd4hep::Position mean_direction(const dd4hep::Position &p1, const dd4hep::Position &p2)
Calculate the mean direction of two vectors.
The main interface to the dd4hep detector description package.
The base class for Geant4 sensitive detector actions implemented by users.
virtual void begin(G4HCofThisEvent *hce) override
G4VSensitiveDetector interface: Method invoked at the begining of each event.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history) final
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
Generic context to extend user, run and event information.