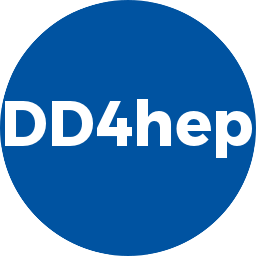 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDCORE_SRC_PLUGINS_LCDDCONVERTER_H
15 #define DDCORE_SRC_PLUGINS_LCDDCONVERTER_H
42 class SensitiveDetectorObject;
55 typedef std::map<LimitSet, XmlElement*>
LimitMap;
58 typedef std::map<SensitiveDetector, XmlElement*>
SensDetMap;
60 typedef std::map<IDDescriptor, XmlElement*>
IdSpecMap;
61 typedef std::map<VisAttr, XmlElement*>
VisMap;
62 typedef std::map<const TGeoShape*, XmlElement*>
SolidMap;
63 typedef std::map<OverlayedField, XmlElement*>
FieldMap;
64 typedef std::map<const TGeoMatrix*, XmlElement*>
TrafoMap;
90 typedef std::map<std::string, const TNamed*>
CheckIter;
95 void check(
const std::string& name,
const TNamed* n, std::map<std::string, const TNamed*>& array)
const;
157 virtual xml_h handleSolid(
const std::string& name,
const TGeoShape* volume)
const;
162 virtual void collectVolume(
const std::string& name,
const TGeoVolume* volume)
const;
202 #endif // DDCORE_SRC_PLUGINS_LCDDCONVERTER_H
std::map< Volume, XmlElement * > VolumeMap
std::map< PlacedVolume, XmlElement * > PlacementMap
Handle class describing an element in the periodic table.
Detector & m_detDesc
Reference to detector description.
Handle class to hold the information of a sensitive detector.
std::map< std::string, PropertyValues > Properties
std::map< SensitiveDetector, XmlElement * > SensDetMap
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
Data container to store information obtained during the geometry scan.
Class implementing the ID encoding of the detector response.
std::map< Region, XmlElement * > RegionMap
xml_doc_t createDetector(DetElement top)
Create geometry conversion in Detector format.
virtual xml_h handleField(const std::string &name, OverlayedField field) const
Convert the electric or magnetic fields into the corresponding Xml object(s).
std::map< std::string, const TNamed * > positions
std::set< LimitSet > limits
virtual xml_h handleRotation(const std::string &name, const TGeoMatrix *trafo) const
Convert the Rotation into the corresponding Xml object(s).
Class to easily access the properties of single XmlElements.
virtual xml_h handleElement(const std::string &name, Atom element) const
Convert the geometry type element into the corresponding Xml object(s).
GeometryInfo & data() const
Geometry converter from dd4hep to Geant 4 in Detector format.
virtual xml_h handleSolid(const std::string &name, const TGeoShape *volume) const
Convert the geometry type solid into the corresponding Xml object(s).
Class describing a field overlay with several sources.
virtual xml_h handleMaterial(const std::string &name, Material medium) const
Convert the geometry type material into the corresponding Xml object(s).
std::map< std::string, const TNamed * > solids
virtual xml_h handlePlacement(const std::string &name, PlacedVolume node) const
Convert the geometry type volume placement into the corresponding Xml object(s).
xml::XmlElement XmlElement
xml_doc_t createVis(DetElement top)
Create geometry conversion in Vis format.
void checkPosition(const std::string &name, const TNamed *n) const
Handle class describing a material.
virtual void collectVolume(const std::string &name, const TGeoVolume *volume) const
Dump logical volume in GDML format to output stream.
void checkMaterial(const std::string &name, Material n) const
Handle class describing a detector element.
xml_doc_t createGDML(DetElement top)
Create geometry conversion in GDML format.
Handle class holding a placed volume (also called physical volume)
std::map< OverlayedField, XmlElement * > FieldMap
The base class for all dd4hep geometry crawlers.
std::map< const TGeoShape *, XmlElement * > SolidMap
Handle class describing a set of limits as they are used for simulation.
Data structure of the geometry converter from dd4hep to Geant 4 in Detector format.
void checkVolume(const std::string &name, const TNamed *n) const
void checkVolumes(const std::string &name, Volume volume) const
Data integrity checker.
std::map< std::string, const TNamed * > volumes
std::set< Region > regions
virtual xml_h handleVolume(const std::string &name, Volume volume) const
Convert the geometry type logical volume into the corresponding Xml object(s).
std::map< VisAttr, XmlElement * > VisMap
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
std::map< Atom, XmlElement * > ElementMap
Handle class describing a region as used in simulation.
std::map< LimitSet, XmlElement * > LimitMap
virtual ~LCDDConverter()
Standard destructor.
std::map< std::string, const TNamed * > materials
void handleProperties(Detector::Properties &prp) const
Handle the geant 4 specific properties.
Class supporting the basic functionality of an XML document.
virtual xml_h handleRegion(const std::string &name, Region region) const
Convert the geometry type region into the corresponding Xml object(s).
std::map< const TGeoMatrix *, XmlElement * > TrafoMap
std::set< SensitiveDetector > sensitives
void checkShape(const std::string &name, const TNamed *n) const
virtual xml_h handleIdSpec(const std::string &name, IDDescriptor idspec) const
Convert the geometry id dictionary entry to the corresponding Xml object(s).
virtual xml_h handleLimitSet(const std::string &name, LimitSet limitset) const
Convert the geometry type LimitSet into the corresponding Xml object(s).
virtual xml_h handleVolumeVis(const std::string &name, const TGeoVolume *volume) const
Dump logical volume in GDML format to output stream.
T * ptr() const
Access to the held object.
virtual void handleHeader() const
Add header information in Detector format.
User abstraction class to manipulate XML elements within a document.
void check(const std::string &name, const TNamed *n, std::map< std::string, const TNamed * > &array) const
Namespace for the AIDA detector description toolkit.
PlacementMap xmlPlacements
LCDDConverter(Detector &description)
Initializing Constructor.
The main interface to the dd4hep detector description package.
virtual xml_h handleSensitive(const std::string &name, SensitiveDetector sens_det) const
Convert the geometry type SensitiveDetector into the corresponding Xml object(s).
std::map< std::string, const TNamed * > rotations
Handle class supporting generic Segmentations of sensitive detectors.
std::map< IDDescriptor, XmlElement * > IdSpecMap
virtual xml_h handleSegmentation(Segmentation seg) const
Convert the segmentation of a SensitiveDetector into the corresponding Detector object.
std::set< std::string > NameSet
GeometryInfo()
Helper constructor.
virtual xml_h handlePosition(const std::string &name, const TGeoMatrix *trafo) const
Convert the Position into the corresponding Xml object(s).
std::map< Material, XmlElement * > MaterialMap
std::map< std::string, const TNamed * > CheckIter
void checkRotation(const std::string &name, const TNamed *n) const
virtual xml_h handleVis(const std::string &name, VisAttr vis) const
Convert the geometry visualisation attributes to the corresponding Xml object(s).