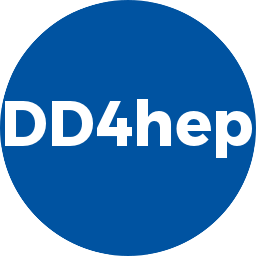 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
35 : detector(element),
delta(del), path(p), overlap(ov)
42 : detector(e.detector),
delta(e.
delta), path(e.path), overlap(e.overlap)
90 detail::destroyObjects(
m_stack);
96 if ( _stack().
get() )
return *_stack();
97 except(
"GlobalAlignmentStack",
"Stack not allocated -- may not be retrieved!");
98 throw std::runtime_error(
"Stack not allocated");
103 if ( _stack().
get() ) {
104 except(
"GlobalAlignmentStack",
"Stack already allocated. Multiple copies are not allowed!");
111 return _stack().get() != 0;
116 if ( _stack().
get() ) {
120 except(
"GlobalAlignmentStack",
"Attempt to delete non existing stack.");
125 if ( entry.get() && !full_path.empty() ) {
126 entry->path = full_path;
129 except(
"GlobalAlignmentStack",
"Attempt to apply an invalid alignment entry.");
140 if ( entry.get() && !entry->path.empty() ) {
141 Stack::const_iterator i =
m_stack.find(entry->path);
146 except(
"GlobalAlignmentStack",
"Invalid alignment entry [No such detector]");
148 printout(INFO,
"GlobalAlignmentStack",
"Add node:%s",e->
path.c_str());
152 except(
"GlobalAlignmentStack",
"The entry with path "+entry->path+
153 " cannot be re-aligned twice in one transaction.");
155 except(
"GlobalAlignmentStack",
"Attempt to apply an invalid alignment entry.");
161 Stack::iterator i =
m_stack.begin();
167 except(
"GlobalAlignmentStack",
"Alignment stack is empty. "
168 "Cannot pop entries - check size first!");
174 std::vector<const StackEntry*> result;
175 result.reserve(
m_stack.size());
static GlobalAlignmentStack & get()
Static client accessor.
DetElement detector
Reference to the detector element.
Alignment Stack object definition.
GlobalAlignmentStack()
Default constructor.
static void create()
Create an alignment stack instance. The creation of a second instance will be refused.
bool insert(const std::string &full_path, dd4hep_ptr< StackEntry > &new_entry)
Add a new entry to the cache. The key is the placement path.
bool isValid() const
Check the validity of the object held by the handle.
static void increment(T *)
Increment count according to type information.
dd4hep_ptr & adopt(T *ptr)
Assignment operator.
Class describing an condition to re-adjust an alignment.
StackEntry()=delete
Default constructor.
Handle class describing a detector element.
static void decrement(T *)
Decrement count according to type information.
std::vector< const StackEntry * > entries() const
Get all path entries to be aligned. Note: transient!
virtual ~StackEntry()
Default destructor.
dd4hep_ptr< StackEntry > pop()
Retrieve an alignment entry of the current stack.
Stack m_stack
The subdetector specific map of alignments caches.
std::string path
Path to the misaligned volume.
bool add(dd4hep_ptr< StackEntry > &new_entry)
Add a new entry to the cache. The key is the placement path.
virtual ~GlobalAlignmentStack()
Default destructor. Careful with this one:
static bool exists()
Check existence of alignment stack.
void release()
Clear data content and remove the slignment stack.
Namespace for implementation details of the AIDA detector description toolkit.
Out version of the std auto_ptr implementation base either on auto_ptr or unique_ptr.