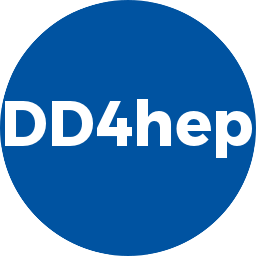 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
20 class G4LogicalVolume;
50 const G4LogicalVolume*
volume,
51 const std::string&
path);
59 typedef std::vector<StepInfo*>
Steps;
68 virtual void operator()(
const G4Step* step, G4SteppingManager* mgr);
70 virtual void begin(
const G4Track* track);
72 virtual void end(
const G4Track* track);
94 #include <CLHEP/Units/SystemOfUnits.h>
95 #include <G4LogicalVolume.hh>
96 #include <G4Material.hh>
97 #include <G4VSolid.hh>
107 const G4LogicalVolume* vol,
108 const std::string& p)
109 : pre(prePos), post(postPos), path(p), volume(vol)
115 : pre(c.pre), post(c.post), path(c.path), volume(c.volume)
152 for_each(
m_steps.begin(),
m_steps.end(),detail::DestroyObject<StepInfo*>());
159 printP2(
"Starting tracking action for track ID=%d",track->GetTrackID());
160 for_each(
m_steps.begin(),
m_steps.end(),detail::DestroyObject<StepInfo*>());
168 constexpr
const char* line =
" +--------------------------------------------------------------------------------------------------------------------------------------------------\n";
169 constexpr
const char* fmt =
" | %5d %11.4f %9.3f (%7.2f,%7.2f,%7.2f) Path:\"/world%s\" Shape:%s Mat:%s\n";
173 ::printf(
"%s + Geometry scan between: x_0 = (%7.2f,%7.2f,%7.2f) [cm] and x_1 = (%7.2f,%7.2f,%7.2f) [cm] TrackID:%d: \n%s",
174 line,start.X()/CLHEP::cm,start.Y()/CLHEP::cm,start.Z()/CLHEP::cm,
175 stop.X()/CLHEP::cm,stop.Y()/CLHEP::cm,stop.Z()/CLHEP::cm,
176 track->GetTrackID(),line);
178 ::printf(
" | Num. \\ Thickness Length Endpoint Volume , Shape , Material\n");
179 ::printf(
" | Layer \\ [cm] [cm] ( cm, cm, cm) \n");
182 for(Steps::const_iterator i=
m_steps.begin(); i!=
m_steps.end(); ++i, ++count) {
183 const G4LogicalVolume* logVol = (*i)->volume;
184 G4Material* material = logVol->GetMaterial();
185 G4VSolid* solid = logVol->GetSolid();
187 const Position& postPos = (*i)->post;
188 Position direction = postPos - prePos;
189 double length = direction.R()/CLHEP::cm;
193 postPos.X()/CLHEP::cm,postPos.Y()/CLHEP::cm,postPos.Z()/CLHEP::cm,
194 (*i)->path.c_str(), typeName(
typeid(*solid)).c_str(), material->GetName().c_str());
196 for_each(
m_steps.begin(),
m_steps.end(),detail::DestroyObject<StepInfo*>());
void printP2(const char *fmt,...) const
Support for messages with variable output level using output level+2.
Helper class to ease the extraction of information from a G4Touchable object.
bool m_needsControl
Default property: Flag to create control instance.
Position prePos() const
Returns the pre-step position.
Concrete implementation of the Geant4 stepping action sequence.
std::string path
Path to this volume.
virtual ~Geant4GeometryScanner()
Default destructor.
static void increment(T *)
Increment count according to type information.
void callAtBegin(Q *p, void(T::*f)(const G4Track *), CallbackSequence::Location where=CallbackSequence::END)
Register Pre-track action callback.
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
void beginEvent(const G4Event *event)
Registered callback on Begin-event.
virtual void end(const G4Track *track)
End-of-tracking callback.
std::size_t printf(const char *fmt,...)
Helper class to ease the extraction of information from a G4Step object.
Geant4EventActionSequence & eventAction() const
Access to the main event action sequence from the kernel object.
void callAtBegin(Q *p, void(T::*f)(const G4Event *))
Register begin-of-event callback.
virtual void operator()(const G4Step *step, G4SteppingManager *mgr)
User stepping callback.
~StepInfo()
Default destructor.
StepInfo & operator=(const StepInfo &c)
Assignment operator.
static void decrement(T *)
Decrement count according to type information.
Class to perform directional material scans using Geantinos.
Geant4GeometryScanner(Geant4Context *context, const std::string &name)
Standard constructor.
const std::string & name() const
Access name of the action.
virtual void begin(const G4Track *track)
Begin-of-tracking callback.
Position postPos() const
Returns the post-step position.
ROOT::Math::XYZVector Position
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Position pre
Pre-step and Post-step position.
std::string path() const
Helper: Access the placement path of a Geant4 touchable object as a string.
const G4LogicalVolume * volume
Reference to the logical volue.
Namespace for the AIDA detector description toolkit.
StepInfo(const Position &pre, const Position &post, const G4LogicalVolume *volume, const std::string &path)
Initializing constructor.
Structure to hold the information of one simulation step.
std::vector< StepInfo * > Steps
Generic context to extend user, run and event information.
G4LogicalVolume * logvol(const G4StepPoint *p) const
Geant4Context * context() const
Access the context.
Geant4TrackingActionSequence & trackingAction() const
Access to the main tracking action sequence from the kernel object.
void callAtEnd(Q *p, void(T::*f)(const G4Track *), CallbackSequence::Location where=CallbackSequence::END)
Register Post-track action callback.