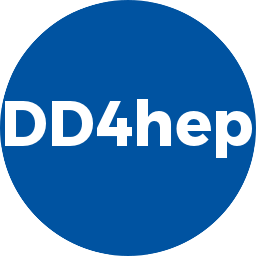 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #include <TGeoManager.h>
24 const std::string& det_name = detector;
32 if ( par.ptr() !=
ptr()->world().
ptr() ) {
35 const auto& ids = pv.
volIDs();
36 for(
const auto& i : ids ) {
37 if ( i.first ==
"system" ) {
52 if ( de.
id() ==
id )
return de;
60 TGeoElementTable* tab = mgr.GetElementTable();
61 TGeoElement* elt = tab->FindElement(nam.c_str());
64 transform(n.begin(), n.end(), n.begin(), ::toupper);
65 elt = tab->FindElement(n.c_str());
67 transform(n.begin(), n.end(), n.begin(), ::tolower);
68 elt = tab->FindElement(n.c_str());
70 n[0] = ::toupper(n[0]);
71 elt = tab->FindElement(n.c_str());
81 TGeoMedium* med = mgr.GetMedium(nam.c_str());
84 transform(n.begin(), n.end(), n.begin(), ::toupper);
85 med = mgr.GetMedium(n.c_str());
87 transform(n.begin(), n.end(), n.begin(), ::tolower);
88 med = mgr.GetMedium(n.c_str());
90 n[0] = ::toupper(n[0]);
91 med = mgr.GetMedium(n.c_str());
virtual TGeoManager & manager() const =0
Access the geometry manager of this instance.
Atom element(const std::string &name) const
Access an element from the element table by name.
Handle class describing an element in the periodic table.
Handle class to hold the information of a sensitive detector.
Handle class holding a placed volume (also called physical volume)
virtual const HandleMap & detectors() const =0
Accessor to the map of sub-detectors.
bool isValid() const
Check the validity of the object held by the handle.
Handle class describing a material.
DetElement detectorByID(int id) const
Find a detector element by its system ID.
Handle class describing a detector element.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
std::map< std::string, Handle< NamedObject > > HandleMap
Type definition of a map of named handles.
SensitiveDetector sensitiveDetector(const std::string &detector) const
Access the sensitive detector of a given subdetector (if the sub-detector is sensitive!...
Detector * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
Detector * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
int id() const
Get the detector identifier.
const PlacedVolumeExtension::VolIDs & volIDs() const
Access to the volume IDs.
Material material(const std::string &name) const
Access a material from the material table by name.