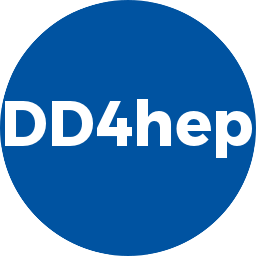 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCORE_SRC_PLUGINS_DETECTORCHECKSUM_H
14 #define DDCORE_SRC_PLUGINS_DETECTORCHECKSUM_H
54 using VisMap = std::map<VisAttr, entry_t>;
57 using FieldMap = std::map<OverlayedField, entry_t>;
58 using TrafoMap = std::map<const TGeoMatrix*, entry_t>;
134 void hash_debug(
const std::string& prefix,
const entry_t& str,
int flag=0)
const;
135 entry_t
make_entry(std::stringstream& log)
const;
136 std::stringstream
logger()
const;
237 #endif // DDCORE_SRC_PLUGINS_DETECTORCHECKSUM_H
std::map< Material, entry_t > MaterialMap
std::stringstream debug_hash
virtual const entry_t & handleHeader() const
Add header information in Detector format.
virtual const entry_t & handleDetElement(DetElement det) const
std::string attr_name(T handle) const
std::map< PlacedVolume, entry_t > PlacementMap
void dump_materials() const
Dump materials used in this apparatus.
std::map< OverlayedField, entry_t > FieldMap
void checksumPlacement(PlacedVolume pv, hashes_t &hashes, bool recursive) const
std::map< const TGeoMatrix *, entry_t > TrafoMap
std::map< SensitiveDetector, entry_t > SensDetMap
Handle class describing an element in the periodic table.
void dump_positions() const
Dump positions used in this apparatus.
Geometry converter from dd4hep to Geant 4 in Detector format.
void collect_det_elements(DetElement top) const
Handle class to hold the information of a sensitive detector.
virtual const entry_t & handleSolid(Solid solid) const
Convert the geometry type solid into the corresponding gdml string.
void dump_placements() const
Dump placements used in this apparatus.
std::map< std::string, PropertyValues > Properties
void dump_solids() const
Dump solids used in this apparatus.
std::string m_len_unit_nam
MapOfDetElements mapOfDetElements
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
Data container to store information obtained during the geometry scan.
PlacementMap mapOfPlacements
std::map< Alignment, entry_t > AlignmentMap
void dump_detelements() const
Dump detelements used in this apparatus.
void dump_volumes() const
Dump volumes used in this apparatus.
std::map< Solid, entry_t > SolidMap
int hash_readout
Property: Include readout property in detector hash.
virtual const entry_t & handleSegmentation(Segmentation seg) const
Convert the segmentation of a SensitiveDetector into the corresponding Detector object.
int have_hash_strings
Property: Keep hash-strings, not only hash values (debugging)
AlignmentMap mapOfAlignments
Class implementing the ID encoding of the detector response.
virtual const entry_t & handleMaterial(Material medium) const
Convert the geometry type material into the corresponding gdml string.
GeometryInfo & data() const
void dump_elements() const
Dump elements used in this apparatus.
void dump_iddescriptors() const
Dump iddescriptors used in this apparatus.
virtual ~DetectorChecksum()
Standard destructor.
virtual const entry_t & handleIdSpec(IDDescriptor idspec) const
Convert the geometry id dictionary entry to the corresponding gdml string.
std::stringstream logger() const
int max_level
Property: maximum depth level for printouts.
void hash_debug(const std::string &prefix, const entry_t &str, int flag=0) const
void dump_sensitives() const
Dump sensitives used in this apparatus.
virtual const entry_t & handleField(OverlayedField field) const
Convert the electric or magnetic fields into the corresponding gdml string.
Class describing a field overlay with several sources.
virtual const entry_t & handleElement(Atom element) const
Convert the geometry type element into the corresponding gdml string.
std::map< Segmentation, entry_t > SegmentationMap
Handle class describing a material.
virtual const entry_t & handleRotation(const TGeoMatrix *trafo) const
Convert the Rotation into the corresponding gdml string.
int debug
Property: debug level.
void dump_rotations() const
Dump rotations used in this apparatus.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
virtual const entry_t & handleVolume(Volume volume) const
Convert the geometry type logical volume into the corresponding gdml string.
std::map< VisAttr, entry_t > VisMap
The base class for all dd4hep geometry crawlers.
std::string refName(T handle) const
int hash_meshes
Property: Include meshed solids in detector hash.
std::map< DetElement, entry_t > MapOfDetElements
Handle class describing a set of limits as they are used for simulation.
const entry_t & handleAlignment(Alignment alignment) const
Convert alignment entry into the corresponding gdml string.
Handle class describing a region as used in simulation.
Main handle class to hold an alignment object.
DetectorChecksum(Detector &description)
Initializing Constructor.
void dump_segmentations() const
Dump segmentations used in this apparatus.
virtual void collectVolume(Volume volume) const
Dump logical volume in GDML format to output stream.
std::string m_densunit_nam
virtual const entry_t & handlePosition(const TGeoMatrix *trafo) const
Convert the Position into the corresponding gdml string.
virtual const entry_t & handlePlacement(PlacedVolume node) const
Convert the geometry type volume placement into the corresponding gdml string.
std::map< Region, entry_t > RegionMap
std::map< IDDescriptor, entry_t > IdSpecMap
const entry_t & handleProperties(Detector::Properties &prp) const
Handle the geant 4 specific properties.
virtual const entry_t & handleSensitive(SensitiveDetector sens_det) const
Convert the geometry type SensitiveDetector into the corresponding gdml string.
SegmentationMap mapOfSegmentations
void checksumDetElement(int level, DetElement det, hashes_t &hashes, bool recursive) const
std::map< Volume, entry_t > VolumeMap
virtual const entry_t & handleLimitSet(LimitSet limitset) const
Convert the geometry type LimitSet into the corresponding gdml string.
std::map< Atom, entry_t > ElementMap
int precision
Property: precision of hashed printouts.
Data structure of the geometry converter from dd4hep to Geant 4 in Detector format.
entry_t make_entry(std::stringstream &log) const
Detector & m_detDesc
Reference to detector description.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
void analyzeDetector(DetElement top)
Create geometry conversion in Detector format.
Handle class supporting generic Segmentations of sensitive detectors.
std::map< LimitSet, entry_t > LimitMap
MaterialMap mapOfMaterials
std::vector< hash_t > hashes_t
virtual const entry_t & handleVis(VisAttr vis) const
Convert the geometry visualisation attributes to the corresponding gdml string.
std::string m_ang_unit_nam
virtual const entry_t & handleRegion(Region region) const
Convert the geometry type region into the corresponding gdml string.
void handle(const O *o, const C &c, F pmf)
std::string m_atomunit_nam
std::string m_ene_unit_nam